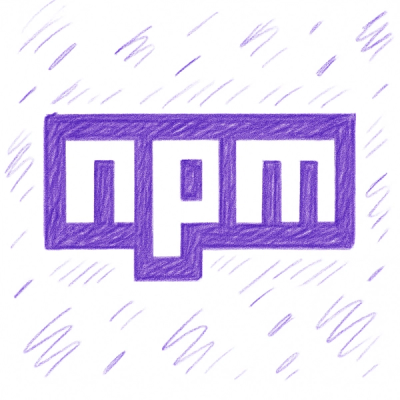
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
simple-sock
offers a straightforward interface for TCP and UDP sockets in Python, with support for optional SSL/TLS encryption and routing for TCP connections.
from simple_sock import Server
app = Server()
@app.tcp_listen()
def handle_tcp(data):
print("Received:", data.msg)
return "Response from TCP server"
app.run("localhost", 5454) # Optional parameters: keyfile=None, certfile=None, timeout=0, buffer_size=1024
from simple_sock import Server
app = Server()
@app.parse_route
def parse_route(data):
return data.msg.split()[0]
@app.tcp_listen()
def default_route(data):
return "Default response"
@app.tcp_listen("route1")
def route1(data):
return "Response for route1"
app.run("localhost", 5454)
from simple_sock import Client
client = Client()
client.connect("localhost", 5454) # Optional parameters: udp=False, tls=False
client.send("Hello from TCP client")
response = client.recv()
print(response)
client.close()
UDP usage is similar; use @app.udp_listen()
for servers and set udp=True
for clients. Example:
# UDP Server
app = Server(udp=True)
@app.udp_listen()
def handle_udp(data):
print("Received:", data.msg)
return "Response from UDP server"
app.run("localhost", 5455")
# UDP Client
client.connect("localhost", 5455, udp=True)
client.send("Hello from UDP client")
response, addr = client.recv()
print(response)
client.close()
MIT License
For questions, contact Leonardo Oliveira at email.
FAQs
A Python library for simplified network and HTTP connections management.
We found that simple-sock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.