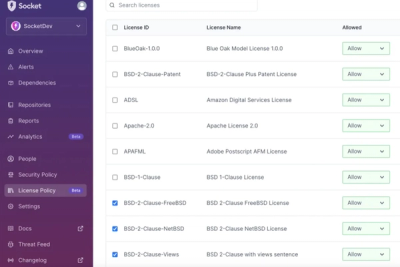
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
SQLite is a lightweight database written in C. Python has in-built support to interact with the database (locally) which is either stored on disk or in memory.
With sqlite_rx
, clients should be able to communicate with an SQLiteServer
in a fast, simple and secure manner and execute queries remotely.
Key Features
pip install -U sqlite_rx
SQLiteServer
runs in a single thread and follows an event-driven concurrency model (using tornado's
event loop) which minimizes the cost of concurrent client connections. Following snippet shows how you can start the server process.
# server.py
from sqlite_rx.server import SQLiteServer
def main():
# database is a path-like object giving the pathname
# of the database file to be opened.
# You can use ":memory:" to open a database connection to a database
# that resides in RAM instead of on disk
server = SQLiteServer(database=":memory:",
bind_address="tcp://127.0.0.1:5000")
server.start()
server.join()
if __name__ == '__main__':
main()
The following snippet shows how you can instantiate an SQLiteClient
and execute a simple CREATE TABLE
query.
# client.py
from sqlite_rx.client import SQLiteClient
client = SQLiteClient(connect_address="tcp://127.0.0.1:5000")
with client:
query = "CREATE TABLE stocks (date text, trans text, symbol text, qty real, price real)"
result = client.execute(query)
>> python client.py
{'error': None, 'items': []}
FAQs
Python SQLite Client and Server
We found that sqlite-rx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.