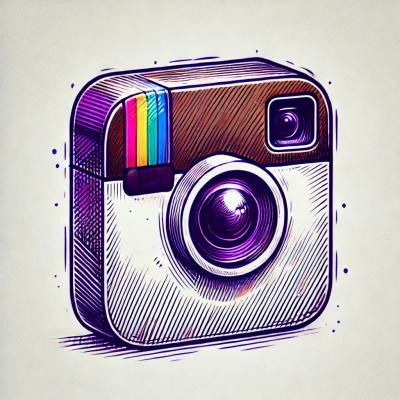
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
building on the awesome https://github.com/namuyan/srudp this is an extension to allow Secure Reliable Data Stream(s) that works like TCP. Purpose is to enable users to create P2P connections between clients in closed NAT.
Rendezvous data can either be passed manually or exchanged via the Autonomi Network. (Then pointers to the Communication partners need to be passed instead of cleartext IP:PORT combinations. Communication partners then can update their connection info when they go online and get available for connections. Since this data can be polled/discovered on a trigger this enables fully decentralized p2p communication even without any centralized servers involved for peer discovery.)
sequenceDiagram
participant A as Client A
participant B as Client B
A->>B: udp-hole-punching
B->>A: udp-hole-punching (fail)
B->>A: send B's publicKey
A->>B: send sharedKey (encrypted by sharedPoint)
B->>A: send establish flag (encrypted by sharedKey)
A->>B: A established
B->>A: B established
gossip protocol on massive-multi-user connections? (not because of the traffic but because of the connection count)
explicit data-routing through other participant; signature based "connect via" if for some reason not everyone can connect to everyone but "everyone is connected to someone"
protocol-relay-connection - for scenarios where even outgoing udp is being blocked
tutorial for users "cannot work on my condition"
poetry add srmudp
cd srmudp
poetry install
poetry run python -m mypy --config-file=mypy.ini srmudp
poetry run python -m unittest discover
These days, PCs are usually located in local environment protected by NAT. It is difficult to transfer data between two parties without special port-forwarding rules being set up on the router. In order to solve this problem, connection is realized by UDP hole punching without the use of UPnP.
UDP is a socket protocol without any handshakes and reliability guarantees. Therefore, there is no connection state, data may be lost, spoofing the source is easy. This is why, you cannot simply use it the same way as TCP. With this library, you can treat the connection just like a TCP connection without worrying about the above issues. In other words, it has a connection state, guarantees data to be delivered (if connected), and is the source is difficult to forge due to cryptography.
MIT
FAQs
Unknown package
We found that srmudp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.