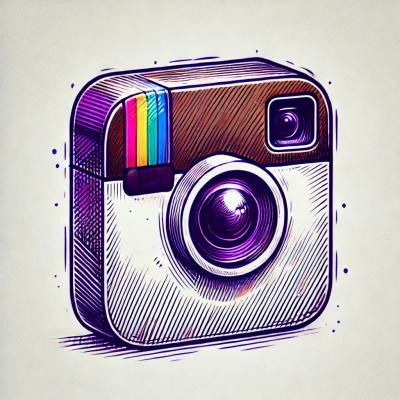
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
StatefulPy provides transparent, persistent state management for regular Python functions.
MIGHT NOT BE FULLY FUNCTIONAL YET, STILL BEING WORKED ON
pip install statefulpy
For Redis support:
pip install statefulpy[redis]
The default serializer used by StatefulPy is Pickle for performance reasons.
However, Pickle is insecure if processing untrusted input.
For public-facing or untrusted data applications, use the JSON serializer instead:
@stateful(backend="sqlite", db_path="state.db", serializer="json")
def my_function():
# Your function code...
from statefulpy import stateful
@stateful(backend="sqlite", db_path="counter.db")
def counter():
# Initialize state if needed
if "count" not in counter.state:
counter.state["count"] = 0
# Update state
counter.state["count"] += 1
return counter.state["count"]
# The counter value persists across runs
print(counter()) # 1 (first run)
print(counter()) # 2
# Restart your program...
print(counter()) # 3 (value loaded from storage)
@stateful(backend="sqlite", db_path="state.db", serializer="pickle")
def my_function():
# Your function code...
@stateful(backend="redis", redis_url="redis://localhost:6379/0")
def my_function():
# Your function code...
from statefulpy import set_backend
# Set default backend for all @stateful functions
set_backend("redis", redis_url="redis://localhost:6379/0")
StatefulPy supports multiple serialization formats depending on your needs:
Using JSON serializer (Recommended for security):
@stateful(backend="sqlite", db_path="state.db", serializer="json")
def my_function():
# Your function code...
Using Pickle serializer (Faster, but only for trusted data):
⚠️ Warning: Only use Pickle when you fully trust your data sources.
@stateful(backend="sqlite", db_path="state.db", serializer="pickle")
def my_function():
# Your function code...
StatefulPy includes CLI utilities for managing backends:
Initialize storage:
statefulpy init --backend sqlite --path state.db
Migrate between backends:
statefulpy migrate --from sqlite --to redis --from-path state.db --to-path redis://localhost:6379/0
Check backend health:
statefulpy healthcheck --backend redis --path redis://localhost:6379/0
This project is licensed under the MIT License.
See the LICENSE file for full details.
FAQs
Transparent persistent state management for Python functions
We found that statefulpy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.