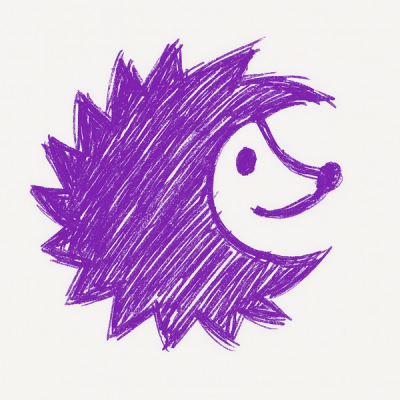
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
An intuitive and type-safe library for converting 2D Python lists to fancy ASCII/Unicode tables
Documentation and examples are available at table2ascii.rtfd.io
pip install -U table2ascii
Requirements: Python 3.7+
from table2ascii import table2ascii
output = table2ascii(
header=["#", "G", "H", "R", "S"],
body=[["1", "30", "40", "35", "30"], ["2", "30", "40", "35", "30"]],
footer=["SUM", "130", "140", "135", "130"],
)
print(output)
"""
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
โ # G H R S โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ 1 30 40 35 30 โ
โ 2 30 40 35 30 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ SUM 130 140 135 130 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
"""
from table2ascii import table2ascii
output = table2ascii(
body=[["Assignment", "30", "40", "35", "30"], ["Bonus", "10", "20", "5", "10"]],
first_col_heading=True,
)
print(output)
"""
โโโโโโโโโโโโโโฆโโโโโโโโโโโโโโโโโโโโ
โ Assignment โ 30 40 35 30 โ
โ Bonus โ 10 20 5 10 โ
โโโโโโโโโโโโโโฉโโโโโโโโโโโโโโโโโโโโ
"""
from table2ascii import table2ascii, Alignment
output = table2ascii(
header=["Product", "Category", "Price", "Rating"],
body=[
["Milk", "Dairy", "$2.99", "6.283"],
["Cheese", "Dairy", "$10.99", "8.2"],
["Apples", "Produce", "$0.99", "10.00"],
],
column_widths=[12, 12, 12, 12],
alignments=[Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL],
)
print(output)
"""
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
โ Product Category Price Rating โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโข
โ Milk Dairy $2.99 6.283 โ
โ Cheese Dairy $10.99 8.2 โ
โ Apples Produce $0.99 10.00 โ
โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ
"""
See a list of 30+ preset styles here.
from table2ascii import table2ascii, Alignment, PresetStyle
output = table2ascii(
header=["First", "Second", "Third", "Fourth"],
body=[["10", "30", "40", "35"], ["20", "10", "20", "5"]],
column_widths=[10, 10, 10, 10],
style=PresetStyle.ascii_box
)
print(output)
"""
+----------+----------+----------+----------+
| First | Second | Third | Fourth |
+----------+----------+----------+----------+
| 10 | 30 | 40 | 35 |
+----------+----------+----------+----------+
| 20 | 10 | 20 | 5 |
+----------+----------+----------+----------+
"""
output = table2ascii(
header=["First", "Second", "Third", "Fourth"],
body=[["10", "30", "40", "35"], ["20", "10", "20", "5"]],
style=PresetStyle.plain,
cell_padding=0,
alignments=Alignment.LEFT,
)
print(output)
"""
First Second Third Fourth
10 30 40 35
20 10 20 5
"""
Check TableStyle
for more info and PresetStyle
for examples.
from table2ascii import table2ascii, TableStyle
my_style = TableStyle.from_string("*-..*||:+-+:+ *''*")
output = table2ascii(
header=["First", "Second", "Third"],
body=[["10", "30", "40"], ["20", "10", "20"], ["30", "20", "30"]],
style=my_style
)
print(output)
"""
*-------.--------.-------*
| First : Second : Third |
+-------:--------:-------+
| 10 : 30 : 40 |
| 20 : 10 : 20 |
| 30 : 20 : 30 |
*-------'--------'-------*
"""
from table2ascii import table2ascii, Merge, PresetStyle
output = table2ascii(
header=["#", "G", "Merge", Merge.LEFT, "S"],
body=[
[1, 5, 6, 200, Merge.LEFT],
[2, "E", "Long cell", Merge.LEFT, Merge.LEFT],
["Bonus", Merge.LEFT, Merge.LEFT, "F", "G"],
],
footer=["SUM", "100", "200", Merge.LEFT, "300"],
style=PresetStyle.double_thin_box,
first_col_heading=True,
)
print(output)
"""
โโโโโโโฆโโโโโโคโโโโโโโโคโโโโโโ
โ # โ G โ Merge โ S โ
โ โโโโโโฌโโโโโโชโโโโคโโโโงโโโโโโฃ
โ 1 โ 5 โ 6 โ 200 โ
โโโโโโโซโโโโโโผโโโโดโโโโโโโโโโข
โ 2 โ E โ Long cell โ
โโโโโโโจโโโโโโดโโโโฌโโโโฌโโโโโโข
โ Bonus โ F โ G โ
โ โโโโโโฆโโโโโโคโโโโงโโโโชโโโโโโฃ
โ SUM โ 100 โ 200 โ 300 โ
โโโโโโโฉโโโโโโงโโโโโโโโงโโโโโโ
"""
All parameters are optional. At least one of header
, body
, and footer
must be provided.
Refer to the documentation for more information.
Option | Supported Types | Description |
---|---|---|
header | Sequence[SupportsStr] , None (Default: None ) | First table row seperated by header row separator. Values should support str() |
body | Sequence[Sequence[SupportsStr]] , None (Default: None ) | 2D List of rows for the main section of the table. Values should support str() |
footer | Sequence[SupportsStr] , None (Default: None ) | Last table row seperated by header row separator. Values should support str() |
column_widths | Sequence[Optional[int]] , None (Default: None / automatic) | List of column widths in characters for each column |
alignments | Sequence[Alignment] , Alignment , None (Default: None / all centered) | Column alignments (ex. [Alignment.LEFT, Alignment.CENTER, Alignment.RIGHT, Alignment.DECIMAL] ) |
number_alignments | Sequence[Alignment] , Alignment , None (Default: None ) | Column alignments for numeric values. alignments will be used if not specified. |
style | TableStyle (Default: double_thin_compact ) | Table style to use for the table* |
first_col_heading | bool (Default: False ) | Whether to add a heading column separator after the first column |
last_col_heading | bool (Default: False ) | Whether to add a heading column separator before the last column |
cell_padding | int (Default: 1 ) | The minimum number of spaces to add between the cell content and the cell border |
use_wcwidth | bool (Default: True ) | Whether to use wcwidth instead of len() to calculate cell width |
*See a list of all preset styles here.
See the API Reference for more info.
Contributions are welcome!
See CONTRIBUTING.md for more details on how to get involved.
FAQs
Convert 2D Python lists into Unicode/ASCII tables
We found that table2ascii demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.