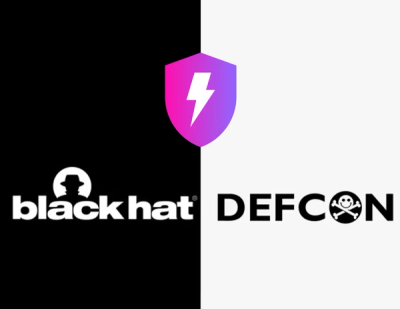
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
tempmail-python is a Python library for generating and managing temporary email addresses using the 1secmail service. It provides functions for creating email addresses, checking for new messages, and retrieving message contents.
You can install tempmail-python using pip:
pip install tempmail-python
Or you can install it from source:
pip install git+https://github.com/cubicbyte/tempmail-python.git
Receive a message (e.g. activation code)
from tempmail import EMail
email = EMail()
print(email.address) # qwerty123@1secmail.com
# ... request some email ...
msg = email.wait_for_message()
print(msg.body) # Hello World!\n
Get all messages in the inbox
from tempmail import EMail
email = EMail('example@1secmail.com')
inbox = email.get_inbox()
for msg_info in inbox:
print(msg_info.subject, msg_info.message.body)
Download an attachment
from tempmail import EMail
email = EMail(username='example', domain='1secmail.com')
msg = email.wait_for_message()
if msg.attachments:
attachment = msg.attachments[0]
data = attachment.download()
# Print
print(data) # b'Hello World!\n'
print(data.decode('utf-8')) # Hello World!\n
# Save to file
with open(attachment.filename, 'wb') as f:
f.write(data)
Get reddit activation code
from tempmail import EMail
def reddit_filter(msg):
return (msg.from_addr == 'noreply@reddit.com' and
msg.subject == 'Verify your Reddit email address')
email = EMail(address='redditaccount@1secmail.com')
msg = email.wait_for_message(filter=reddit_filter)
# get_activation_code(html=msg.html_body)
Some other features:
from tempmail.providers import OneSecMail
email = OneSecMail()
# request_email(email=email.address)
# Speed up inbox refresh rate
OneSecMail.inbox_update_interval = 0.1 # every 100ms
# Accept only emails with a specific subject, raise error after 60 seconds
msg = email.wait_for_message(timeout=60, filter=lambda m: m.subject == 'Hello World!')
print(msg.body)
tempmail-python is licensed under the MIT License. See the LICENSE file for more information.
FAQs
Python library for generating and managing temporary email addresses.
We found that tempmail-python demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.