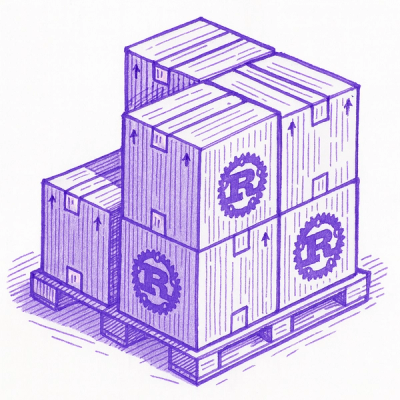
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
tensorbox
tensorbox
allows you to interact with dataclasses of tensors as if they were tensors. Simply use @tensorbox
instead of @dataclass
.
from jaxtyping import Float
from tensorbox import tensorbox
from torch import Tensor
# Define a @tensorbox class. The jaxtyping annotations describe each attribute's scalar (unbatched) shape.
@tensorbox
class Gaussians:
mean: Float[Tensor, "dim"]
covariance: Float[Tensor, "dim dim"]
color: Float[Tensor, "3"]
# Define Gaussians with batch size (10, 10) and dim=3.
gaussians = Gaussians(
torch.zeros((10, 10, 3), dtype=torch.float32),
torch.zeros((10, 10, 3, 3), dtype=torch.float32),
torch.zeros((10, 10, 3), dtype=torch.float32),
)
# Define a function that uses Gaussians as input. When a @tensorbox class is subscripted, each attribute's shape becomes the concatenation of the subscript (batch shape) and the attribute's original (scalar) shape. This means fn expects the following shapes:
# - mean: "batch_a batch_b dim"
# - covariances: "batch_a batch_b dim dim"
# - color: "batch_a batch_b 3"
def fn(g: Gaussians["batch_a batch_b"]):
...
A @tensorbox
class will automatically infer its batch shape:
@tensorbox
class Camera:
intrinsics: Float[Tensor, "3 3"]
extrinsics: Float[Tensor, "4 4"]
cameras = Camera(
torch.zeros((512, 4, 3, 3), dtype=torch.float32),
torch.zeros((512, 4, 4, 4), dtype=torch.float32),
)
cameras.shape # (512, 4)
You can define and use nested @tensorbox
classes as follows:
@tensorbox
class Leaf:
rgb: Float[Tensor, "3"]
scale: Float[Tensor, ""]
@tensorbox
class Tree:
pair: Leaf["2"]
def fn(tree: Tree["*batch"]):
# tree.pair.rgb has shape (*batch, 2, 3)
...
@tensorbox
classes can be used directly with the following torch
functions:
torch.cat
torch.stack
Note that dim
arguments are always specified relative to the @tensorbox
class's batch shape.
tensorbox
is very similar to TensorDict, but has a few key differences:
jaxtyping
annotations.FAQs
Type annotations and runtime checking for dataclass-like containers of tensors.
We found that tensorbox demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.