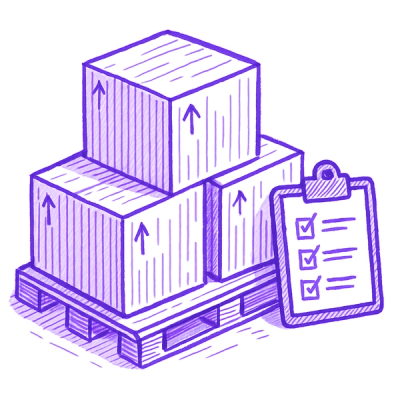
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
text-to-speech-program
Advanced tools
This package provides a text-to-speech server, using gtts
and pydub
, and a client program to interact with the server.
To install the package from pypi, follow the instructions below:
pip install text_to_speech_program
Execute which tts-program-server
to see where it was installed, probably in /home/USERNAME/.local/bin/tts-program-server
.
curl -fsSL https://raw.githubusercontent.com/trucomanx/text_to_speech_program/main/install_linux_service.sh | sh
After the last code, the program server starts at with the operating system. Now the next commands are accepted (Use them if necessary).
You only need to start the server if it has been stopped or is disabled from starting with Linux boot.
sudo systemctl start tts-program-server
sudo systemctl stop tts-program-server
sudo systemctl disable tts-program-server
journalctl -u tts-program-server -f
You only need to start the server if it has been stopped. If the program server was not added to the Linux service, then to start the text-to-speech server, use the command below:
tts-program-server
This starts a server that will listen, by default, on http://127.0.0.1:5000
and will be ready to receive text conversion requests.
To modify these values see tts-program-server config
.
The client can submit conversion text-to-speech tasks or remove pending jobs from the server.
Adding a text-to-speech task.
tts-program-client send /caminho/para/arquivo.json
JSON file example:
{
"text": "Some text to convert.\n\n OK",
"language": "en",
"split_pattern": [".", "\n\n"],
"speed":1.25
}
Adding a text-to-speech task.
tts-program-client senddict '{
"text": "Some text to convert. OK",
"language": "en",
"split_pattern": ["."],
"speed":1.25
}'
or if host is localhost
and port is 5000
. See tts-program-client config
to conf the localhost and port.
curl -X POST http://localhost:5000/add_task \
-H "Content-Type: application/json" \
-d '{
"text": "Some text to convert. OK",
"language": "en",
"split_pattern": ["."], "speed":1.25
}'
tts-program-client remove <ID>
Replace <ID>
with the unique ID returned when adding a task.
import requests
def remove_task(server_url,task_id):
# Send DELETE request to server
response = requests.delete(f'{server_url}/remove_task/{task_id}')
if response.status_code == 200:
print(response.json()["message"])
return response.json()["message"]
else:
print("Error removing task:",task_id)
return None
def send_json_from_dict(server_url,data):
"""
Sends a POST request to the server with a JSON payload.
Args:
server_url (str): The base URL of the server.
data (dict): A dictionary containing the data to be sent as JSON.
Returns:
str: The ID of the task if successfully sent, or None if there was an error.
"""
# Send POST request to the server
response = requests.post(f'{server_url}/add_task', json=data)
if response.status_code == 200:
print(f"Task sent successfully! ID: {response.json()['id']}")
return response.json()['id'];
else:
print("Error submitting task.")
return None;
# Example usage:
SERVER_URL = 'http://localhost:5000'; # If host is localhost and port is 5000
DATA={
"text": "Some text to convert. OK",
"language": "en",
"split_pattern": ["."],
"speed":1.25
}
ID=send_json_from_dict(SERVER_URL,DATA);
#msg=remove_task(SERVER_URL,ID);
This project is licensed under the GPL license. See the LICENSE
file for more details.
FAQs
A text-to-speech server and client using gtts and pydub
We found that text-to-speech-program demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.