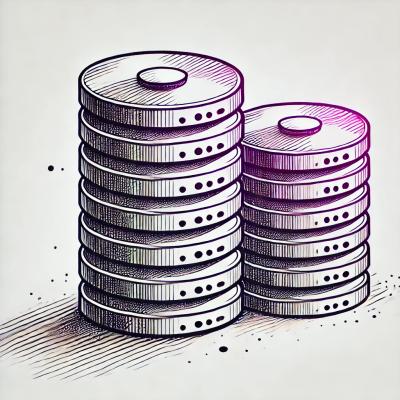
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
.. image:: https://img.shields.io/pypi/v/tiingo.svg?maxAge=600 :target: https://pypi.python.org/pypi/tiingo
.. image:: https://img.shields.io/codecov/c/github/hydrosquall/tiingo-python.svg?maxAge=600 :target: https://codecov.io/gh/hydrosquall/tiingo-python :alt: Coverage
.. image:: https://readthedocs.org/projects/tiingo-python/badge/?version=latest&maxAge=600 :target: https://tiingo-python.readthedocs.io/en/latest/?badge=latest :alt: Documentation Status
.. image:: https://pyup.io/repos/github/hydrosquall/tiingo-python/shield.svg?maxAge=600 :target: https://pyup.io/repos/github/hydrosquall/tiingo-python/ :alt: Updates
.. image:: https://mybinder.org/badge_logo.svg :target: https://mybinder.org/v2/gh/hydrosquall/tiingo-python/master?filepath=examples%2Fbasic-usage-with-pandas.ipynb :alt: Launch Binder
Tiingo is a financial data platform making high quality financial tools available to all. Tiingo has a REST and Real-Time Data API, which this library helps you access. The API includes support for these endpoints:
If you'd like to try this library before installing, click below to open a folder of online runnable examples.
.. image:: https://mybinder.org/badge_logo.svg :target: https://mybinder.org/v2/gh/hydrosquall/tiingo-python/master?filepath=examples :alt: Launch Binder
First, install the library from PyPi:
.. code-block:: shell
pip install tiingo
If you prefer to receive your results in pandas DataFrame
or Series
format, and you do not already have pandas installed, install it as an optional dependency:
.. code-block:: shell
pip install tiingo[pandas]
Next, initialize your client. It is recommended to use an environment variable to initialize your client for convenience.
.. code-block:: python
from tiingo import TiingoClient
client = TiingoClient()
Alternately, you may use a dictionary to customize/authorize your client.
.. code-block:: python
config = {}
config['session'] = True
config['api_key'] = "MY_SECRET_API_KEY"
client = TiingoClient(config)
Now you can use TiingoClient
to make your API calls. (Other parameters are available for each endpoint beyond what is used in the below examples, inspect the docstring
for each function for details.).
.. code-block:: python
ticker_metadata = client.get_ticker_metadata("GOOGL")
ticker_price = client.get_ticker_price("GOOGL", frequency="weekly")
ticker_price = client.get_ticker_price("GOOGL", frequency="1min", columns="open,close,volume")
historical_prices = client.get_ticker_price("GOOGL", fmt='json', startDate='2017-08-01', endDate='2017-08-31', frequency='daily')
tickers = client.list_stock_tickers()
articles = client.get_news(tickers=['GOOGL', 'AAPL'], tags=['Laptops'], sources=['washingtonpost.com'], startDate='2017-01-01', endDate='2017-08-31')
definitions = client.get_fundamentals_definitions('GOOGL')
fundamentals_daily = client.get_fundamentals_daily('GOOGL', startDate='2020-01-01', endDate='2020-12-31')
fundamentals_stmnts = client.get_fundamentals_statements('GOOGL', startDate='2020-01-01', endDate='2020-12-31', asReported=True)
To receive results in pandas
format, use the get_dataframe()
method:
.. code-block:: python
#Get a pd.DataFrame of the price history of a single symbol (default is daily): ticker_history = client.get_dataframe("GOOGL")
#The method returns all of the available information on a symbol, such as open, high, low, close, #adjusted close, etc. This page in the tiingo api documentation lists the available information on each #symbol: https://api.tiingo.com/docs/tiingo/daily#priceData.
#Frequencies and start and end dates can be specified similarly to the json method above.
#Get a pd.Series of only one column of the available response data by specifying one of the valid the #'metric_name' parameters: ticker_history = client.get_dataframe("GOOGL", metric_name='adjClose')
#Get a pd.DataFrame for a list of symbols for a specified metric_name (default is adjClose if no #metric_name is specified): ticker_history = client.get_dataframe(['GOOGL', 'AAPL'], frequency='weekly', metric_name='volume', startDate='2017-01-01', endDate='2018-05-31')
#Get a pd.DataFrame for a list of symbols for "close" and "volume" columns: ticker_history = client.get_dataframe(['GOOGL', 'AAPL'], frequency='weekly', columns="close,volume" startDate='2017-01-01', endDate='2018-05-31')
You can specify any of the end of day frequencies (daily, weekly, monthly, and annually) or any intraday frequency for both the get_ticker_price
and get_dataframe
methods. Weekly frequencies resample to the end of day on Friday, monthly frequencies resample to the last day of the month, and annually frequencies resample to the end of
day on 12-31 of each year. The intraday frequencies are specified using an integer followed by "Min" or "Hour", for example "30Min" or "1Hour".
It's also possible to specify which columns you're interested in, for example: "open", "close", "low", "high" and "volume" (see End of Day response docs <https://www.tiingo.com/documentation/end-of-day>
_ for future columns).
.. code-block:: python
client.get_crypto_metadata(['BTCUSD'], fmt='json')
#You can obtain top-of-book cryptocurrency quotes from the get_crypto_top_of_book()
method.
crypto_price = client.get_crypto_top_of_book(['BTCUSD'])``
client.get_crypto_price_history(tickers = ['BTCUSD'], startDate='2020-12-2', endDate='2020-12-3', resampleFreq='1Hour')
.. code-block:: python
from tiingo import TiingoWebsocketClient
def cb_fn(msg):
# Example response
# msg = {
# "service":"iex" # An identifier telling you this is IEX data.
# The value returned by this will correspond to the endpoint argument.
#
# # Will always return "A" meaning new price quotes. There are also H type Heartbeat msgs used to keep the connection alive
# "messageType":"A" # A value telling you what kind of data packet this is from our IEX feed.
#
# # see https://api.tiingo.com/documentation/websockets/iex > Response for more info
# "data":[] # an array containing trade information and a timestamp
#
# }
print(msg)
subscribe = {
'eventName':'subscribe',
'authorization':'API_KEY_GOES_HERE',
#see https://api.tiingo.com/documentation/websockets/iex > Request for more info
'eventData': {
'thresholdLevel':5
}
}
# any logic should be implemented in the callback function (cb_fn)
TiingoWebsocketClient(subscribe,endpoint="iex",on_msg_cb=cb_fn)
tiingo-python
Documentation: https://tiingo-python.readthedocs.io.fmt="object"
as a keyword to have your responses come back as NamedTuples
, which should have a lower memory impact than regular Python dictionaries.Feel free to file a PR that implements any of the above items.
.. _Riingo: https://github.com/business-science/riingo
This package was created with Cookiecutter_ and the audreyr/cookiecutter-pypackage
_ project template.
.. _Cookiecutter: https://github.com/audreyr/cookiecutter
.. _audreyr/cookiecutter-pypackage
: https://github.com/audreyr/cookiecutter-pypackage
vcr.py
(@condemil #32)/news
APIFAQs
REST Client for Tiingo Data Platform API
We found that tiingo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.