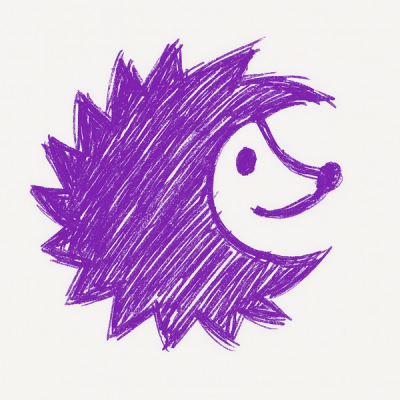
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
A class for converting and manipulating datetime objects based on given timezone offset or name.
A Python library that provides an easy-to-use interface for converting and manipulating datetime objects across different time zones.
pip install timezone-adapter
from datetime import datetime
from timezone_adapter import TimeZoneAdapter
# Using numeric offset (hours)
tz_adapter = TimeZoneAdapter(-5) # UTC-5
# Using timezone name
tz_adapter = TimeZoneAdapter('America/Bogota')
# Convert to UTC
local_time = datetime.now()
utc_time = tz_adapter.to_utc(local_time)
# Get range of dates for today
min_date, max_date = tz_adapter.get_min_max_datetime_today()
__init__(timezone: Union[int, str])
Initialize with either a numeric offset (hours from UTC) or timezone name.
to_utc(date_time: datetime) -> datetime
Convert a local datetime to UTC.
from_utc(date_time: datetime) -> datetime
Convert a UTC datetime to local time.
get_min_max_datetime_today() -> Tuple[datetime, datetime]
Get the minimum and maximum datetime for the current day.
get_min_max_datetime_by_date(date_time: datetime) -> Tuple[datetime, datetime]
Get the minimum and maximum datetime for a specific date.
Clone the repository
git clone https://github.com/miguepoloc/timezone-adapter.git
cd timezone-adapter
Create and activate virtual environment
python -m venv .venv
source .venv/bin/activate # On Windows: .venv\Scripts\activate
Install development dependencies
pip install -e ".[dev]"
Run tests
pytest
We use several tools to ensure code quality:
black
for code formattingisort
for import sortingmypy
for type checkingflake8
for style guide enforcementRun all checks with:
pre-commit run --all-files
Contributions are welcome! Please feel free to submit a Pull Request.
git checkout -b feature/AmazingFeature
)git commit -m 'Add some AmazingFeature'
)git push origin feature/AmazingFeature
)This project is licensed under the MIT License - see the LICENSE file for details.
Miguel Angel Polo Castañeda - @miguepoloc
pytz
library for timezone supportFAQs
A class for converting and manipulating datetime objects based on given timezone offset or name.
We found that timezone-adapter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.