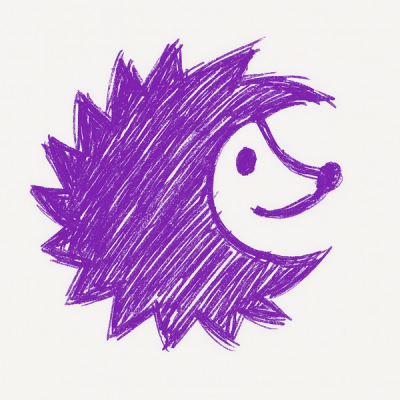
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
tradebest-ctp-python
Advanced tools
tradebest-ctp-python 是一个功能完善、使用便捷的中国金融期货交易平台(CTP)的Python接口封装。
pip install tradebest-ctp-python
from tradebest_ctp import mdapi
class CMdImpl(mdapi.CThostFtdcMdSpi):
def __init__(self, md_front):
mdapi.CThostFtdcMdSpi.__init__(self)
self.md_front = md_front
self.api = None
def Run(self):
self.api = mdapi.CThostFtdcMdApi.CreateFtdcMdApi()
self.api.RegisterFront(self.md_front)
self.api.RegisterSpi(self)
self.api.Init()
def OnFrontConnected(self):
print("OnFrontConnected")
# 登录
req = mdapi.CThostFtdcReqUserLoginField()
self.api.ReqUserLogin(req, 0)
def OnRspUserLogin(self, pRspUserLogin, pRspInfo, nRequestID, bIsLast):
if pRspInfo is not None and pRspInfo.ErrorID != 0:
print(f"Login failed. {pRspInfo.ErrorMsg}")
return
print(f"Login succeed.{pRspUserLogin.TradingDay}")
# 订阅行情
self.api.SubscribeMarketData(["au2406".encode('utf-8')], 1)
def OnRtnDepthMarketData(self, pDepthMarketData):
print(f"{pDepthMarketData.InstrumentID} - {pDepthMarketData.LastPrice} - {pDepthMarketData.Volume}")
if __name__ == '__main__':
md = CMdImpl("tcp://180.168.146.187:10131")
md.Run()
input("Press enter key to exit.")
from tradebest_ctp import tdapi
class TdImpl(tdapi.CThostFtdcTraderSpi):
def __init__(self, host, broker, user, password, appid, authcode):
super().__init__()
self.broker = broker
self.user = user
self.password = password
self.appid = appid
self.authcode = authcode
self.api = tdapi.CThostFtdcTraderApi.CreateFtdcTraderApi()
self.api.RegisterSpi(self)
self.api.RegisterFront(host)
self.api.SubscribePrivateTopic(tdapi.THOST_TERT_QUICK)
self.api.SubscribePublicTopic(tdapi.THOST_TERT_QUICK)
def Run(self):
self.api.Init()
def OnFrontConnected(self):
print("OnFrontConnected")
# 认证
req = tdapi.CThostFtdcReqAuthenticateField()
req.BrokerID = self.broker
req.UserID = self.user
req.AppID = self.appid
req.AuthCode = self.authcode
self.api.ReqAuthenticate(req, 0)
def OnRspAuthenticate(self, pRspAuthenticateField, pRspInfo, nRequestID, bIsLast):
if pRspInfo and pRspInfo.ErrorID != 0:
print("认证失败:{}".format(pRspInfo.ErrorMsg))
return
print("Authenticate succeed.")
# 登录
req = tdapi.CThostFtdcReqUserLoginField()
req.BrokerID = self.broker
req.UserID = self.user
req.Password = self.password
self.api.ReqUserLogin(req, 0)
def OnRspUserLogin(self, pRspUserLogin, pRspInfo, nRequestID, bIsLast):
if pRspInfo is not None and pRspInfo.ErrorID != 0:
print(f"Login failed. {pRspInfo.ErrorMsg}")
return
print(f"Login succeed. TradingDay: {pRspUserLogin.TradingDay}")
# 查询账户资金
self.QryAccount()
def QryAccount(self):
req = tdapi.CThostFtdcQryTradingAccountField()
req.BrokerID = self.broker
req.InvestorID = self.user
self.api.ReqQryTradingAccount(req, 0)
def OnRspQryTradingAccount(self, pTradingAccount, pRspInfo, nRequestID, bIsLast):
if pRspInfo is not None and pRspInfo.ErrorID != 0:
print(f"查询账户资金失败: {pRspInfo.ErrorMsg}")
return
if pTradingAccount is not None:
print(f"账户资金: 可用资金={pTradingAccount.Available}, "
f"当前保证金={pTradingAccount.CurrMargin}, "
f"平仓盈亏={pTradingAccount.CloseProfit}, "
f"持仓盈亏={pTradingAccount.PositionProfit}")
if __name__ == '__main__':
td = TdImpl(
"tcp://180.168.146.187:10101", # 交易前置地址
"9999", # 经纪公司代码
"123456", # 投资者代码
"password", # 密码
"simnow_client_test", # AppID
"0000000000000000" # 授权码
)
td.Run()
input("Press enter key to exit.")
tradebest-ctp-python当前版本支持以下CTP API版本:
未来将只支持最新的CTP API版本,不会支持旧版本。
tradebest-ctp-python支持以下Python版本:
BSD 3-Clause License
FAQs
Python wrapper for CTP API
We found that tradebest-ctp-python demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.