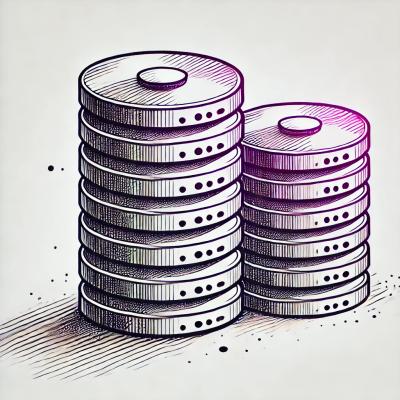
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
The Tripo3d Python SDK is the official Python client library for the Tripo 3D Generation API. With this SDK, you can easily generate high-quality 3D models.
pip install tripo3d
import asyncio
from tripo3d import TripoClient
async def main():
# Initialize client with API key
client = TripoClient(api_key="your_api_key")
# Or read from environment variable
# export TRIPO_API_KEY=your_api_key
# client = TripoClient()
# Use the client
# ...
# Close client connection
await client.close()
# Run async function
asyncio.run(main())
import asyncio
from tripo3d import TripoClient
async def text_to_model_example():
async with TripoClient() as client:
# Create text to 3D model task
task_id = await client.text_to_model(
prompt="A cute cat",
negative_prompt="low quality, blurry",
)
print(f"Task ID: {task_id}")
# Wait for task completion
task = await client.wait_for_task(task_id)
if task.status == TaskStatus.SUCCESS:
print(f"Task completed successfully!")
# Download model files
downloaded_files = await client.download_task_models(task, "./output")
# Print downloaded file paths
for model_type, file_path in downloaded_files.items():
if file_path:
print(f"Downloaded {model_type}: {file_path}")
asyncio.run(text_to_model_example())
import asyncio
from tripo3d import TripoClient
async def image_to_model_example():
async with TripoClient() as client:
# Create image to 3D model task
task_id = await client.image_to_model(
image="path/to/your/image.jpg",
)
print(f"Task ID: {task_id}")
# Wait for task completion and show progress
task = await client.wait_for_task(task_id, verbose=True)
if task.status == TaskStatus.SUCCESS:
print(f"Task completed successfully!")
# Download model files
downloaded_files = await client.download_task_models(task, "./output")
# Print downloaded file paths
for model_type, file_path in downloaded_files.items():
if file_path:
print(f"Downloaded {model_type}: {file_path}")
asyncio.run(image_to_model_example())
import asyncio
from tripo3d import TripoClient
async def multiview_to_model_example():
async with TripoClient() as client:
# Create multi-view to 3D model task
task_id = await client.multiview_to_model(
images=[
"path/to/front.jpg", # Front view (required)
"path/to/back.jpg", # Back view (optional)
"path/to/left.jpg", # Left view (optional)
"path/to/right.jpg" # Right view (optional)
],
)
print(f"Task ID: {task_id}")
# Wait for task completion and show progress
task = await client.wait_for_task(task_id, verbose=True)
if task.status == TaskStatus.SUCCESS:
print(f"Task completed successfully!")
# Download model files
downloaded_files = await client.download_task_models(task, "./output")
# Print downloaded file paths
for model_type, file_path in downloaded_files.items():
if file_path:
print(f"Downloaded {model_type}: {file_path}")
asyncio.run(multiview_to_model_example())
import asyncio
from tripo3d import TripoClient
async def check_balance():
async with TripoClient() as client:
balance = await client.get_balance()
print(f"Available balance: {balance.balance}")
print(f"Frozen amount: {balance.frozen}")
asyncio.run(check_balance())
For more advanced usage examples, check out the examples directory.
The complete API documentation can be found here.
MIT
FAQs
Python client for the Tripo 3D Generation API
We found that tripo3d demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.