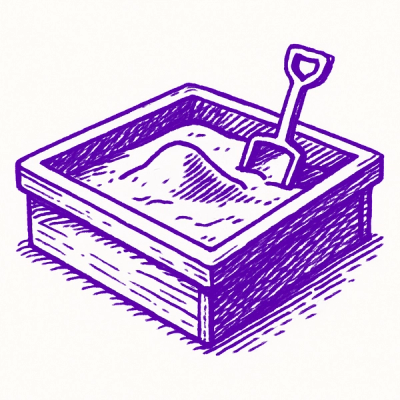
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
A simple UUID-based file lock implementation for Python. This package provides a lightweight and effective way to coordinate access to shared resources using a lock file mechanism. This does not require any file locking capabilities of the underlying filesystem or network share.
This mechanism ensures that only one process can hold the lock at any time, making it ideal for coordinating file or resource access across multiple processes.
Note: In order for this to work over a network share the verification_delay
needs to be larger than the time it takes for a client to detect that another has created a file.
Install the package via pip:
pip install uuid-filelock
You can use the UUIDFileLock
class to acquire and release locks in your Python code. The class also supports usage within a with
clause for convenience.
import time
from uuid_filelock import UUIDFileLock
lock_file_path = "/tmp/mylockfile.lock"
lock = UUIDFileLock(lock_file_path)
print("Acquire lock...")
lock.acquire()
print("Lock acquired!")
# Perform critical section tasks here
# Simulating work
time.sleep(5)
# Release the lock
lock.release()
print("Lock released.")
with
Clauseimport time
from uuid_filelock import UUIDFileLock
lock_file_path = "/tmp/mylockfile.lock"
with UUIDFileLock(lock_file_path) as lock:
print("Lock acquired!")
# Perform critical section tasks
time.sleep(5) # Simulate work
print("Work done!")
# The lock is automatically released when the block exits.
print("Lock released.")
lock_file
(str): The path to the lock file.prefix
(str, default=""): A custom prefix for the UUID to help debugging.verification_delay
(float, default=1.0): Time to wait before verifying that the lock was acquired.retry_interval
(float, default=0.1): Time to wait before retrying if the lock cannot be acquired.timeout_interval
(float, default=-1): Timeout for acquiring lock. Set to negative value for no timeout.You can change the default values for verification_delay
and retry_interval
via UUIDFileLock.verification_delay=<new_value>
and UUIDFileLock.retry_interval=<new_value>
respectively.
This package is licensed under the MIT License.
FAQs
A simple UUID-based file lock implementation for Python.
We found that uuid-filelock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.