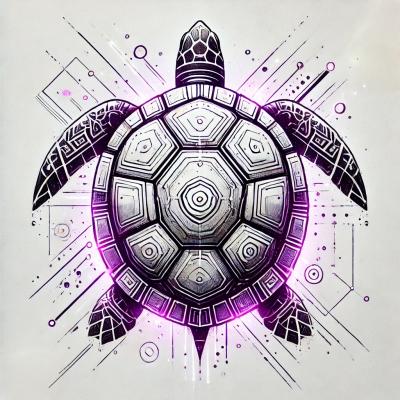
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Dataclass with data validation. Checks the value of its fields by their annotations.
ValidatedDC
is a regular Python dataclass.
typing
module, namely: Any
, List
, Literal
, Optional
, Union
. These aliases can be embedded in each other.dict
instead of the ValidatedDC
instance specified in the field annotation (useful, for example, when retrieving data via api).is_valid()
function at any time.get_errors()
function will show the full traceback of errors in the fields, including errors of nested classes.See detailed in the examples
folder.
pip install validated-dc
Versions 3.7
, 3.8
and 3.9
are currently supported.
To work with version python 3.7
you need to install:
pip install typing_extensions
from dataclasses import dataclass
from typing import List, Union
from validated_dc import ValidatedDC, get_errors, is_valid
# Some combinations of List and Union
@dataclass
class Foo(ValidatedDC):
value: Union[int, List[int]]
@dataclass
class Bar(ValidatedDC):
foo: Union[Foo, List[Foo]]
# --- Valid input ---
foo = {'value': 1}
instance = Bar(foo=foo)
assert get_errors(instance) is None
assert instance == Bar(foo=Foo(value=1))
foo = {'value': [1, 2]}
instance = Bar(foo=foo)
assert get_errors(instance) is None
assert instance == Bar(foo=Foo(value=[1, 2]))
foo = [{'value': 1}, {'value': 2}]
instance = Bar(foo=foo)
assert get_errors(instance) is None
assert instance == Bar(foo=[Foo(value=1), Foo(value=2)])
foo = [{'value': [1, 2]}, {'value': [3, 4]}]
instance = Bar(foo=foo)
assert get_errors(instance) is None
assert instance == Bar(foo=[Foo(value=[1, 2]), Foo(value=[3, 4])])
# --- Invalid input ---
foo = {'value': 'S'}
instance = Bar(foo=foo)
assert get_errors(instance)
assert instance == Bar(foo={'value': 'S'})
# fix
instance.foo['value'] = 1
assert is_valid(instance)
assert get_errors(instance) is None
assert instance == Bar(foo=Foo(value=1))
foo = [{'value': [1, 2]}, {'value': ['S', 4]}]
instance = Bar(foo=foo)
assert get_errors(instance)
assert instance == Bar(foo=[{'value': [1, 2]}, {'value': ['S', 4]}])
# fix
instance.foo[1]['value'][0] = 3
assert is_valid(instance)
assert get_errors(instance) is None
assert instance == Bar(foo=[Foo(value=[1, 2]), Foo(value=[3, 4])])
# --- get_errors() ---
foo = {'value': 'S'}
instance = Bar(foo=foo)
print(get_errors(instance))
# {
# 'foo': [
# # An unsuccessful attempt to use the dictionary to create a Foo instance
# InstanceValidationError(
# value_repr="{'value': 'S'}",
# value_type=<class 'dict'>,
# annotation=<class '__main__.Foo'>,
# exception=None,
# errors={
# 'value': [
# BasicValidationError( # because the str isn't an int
# value_repr='S', value_type=<class 'str'>,
# annotation=<class 'int'>, exception=None
# ),
# BasicValidationError( # and the str is not a list of int
# value_repr='S', value_type=<class 'str'>,
# annotation=typing.List[int], exception=None
# )
# ]
# }
# ),
# BasicValidationError( # the dict is not a list of Foo
# value_repr="{'value': 'S'}",
# value_type=<class 'dict'>,
# annotation=typing.List[__main__.Foo],
# exception=None
# )
# ]
# }
FAQs
Dataclass with data validation.
We found that validated-dc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.