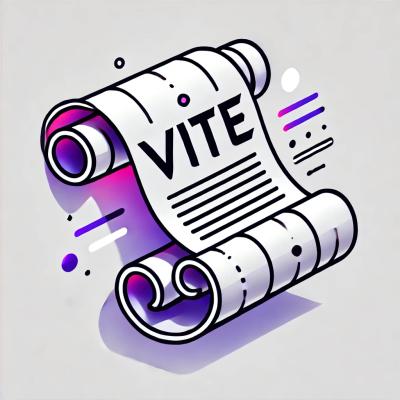
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Python client for the WeGlide API. WeGlide is an online platform where glider pilots can share and analyze their flights, participate in competitions, and connect with the global gliding community.
A Python client library for the WeGlide API. WeGlide is an online platform where glider pilots can share and analyze their flights, participate in competitions, and connect with the global gliding community.
This package is automatically generated by the OpenAPI Generator project:
pip install weglide-client
Clone the repository and install in development mode:
git clone https://github.com/develmusa/weglide-python-client.git
cd weglide-python-client
pip install -e .
pip install --group dev .
Or using uv
:
git clone https://github.com/develmusa/weglide-python-client.git
cd weglide-python-client
uv venv
uv pip install -e .
uv pip install --group dev .
import weglide_client
from weglide_client.rest import ApiException
from pprint import pprint
# Configure the API client
# The host defaults to https://api.weglide.org if not specified
configuration = weglide_client.Configuration(
host="https://api.weglide.org"
)
# Create an API client instance
with weglide_client.ApiClient(configuration) as api_client:
# Create an instance of an API class
airport_api = weglide_client.AirportApi(api_client)
try:
# Get information about a specific airport
airport_id = 161522 # Example airport ID
api_response = airport_api.get_airport_v1_airport_id_get(id=airport_id)
print("Airport information:")
pprint(api_response)
except ApiException as e:
print(f"Exception when calling AirportApi: {e}")
For endpoints that require authentication, you'll need to provide an API token:
import weglide_client
from weglide_client.rest import ApiException
# Configure API key authorization
configuration = weglide_client.Configuration(
host="https://api.weglide.org",
api_key={"Authorization": "YOUR_API_KEY"},
api_key_prefix={"Authorization": "Bearer"}
)
# Use the authenticated client
with weglide_client.ApiClient(configuration) as api_client:
# Access protected endpoints
flight_api = weglide_client.FlightApi(api_client)
# ...
You can also configure the client using environment variables:
import os
import weglide_client
# Set environment variables
os.environ["WEGLIDE_API_URL"] = "https://api.weglide.org"
os.environ["WEGLIDE_API_KEY"] = "YOUR_API_KEY"
# Configure using environment variables
configuration = weglide_client.Configuration()
configuration.host = os.environ.get("WEGLIDE_API_URL", "https://api.weglide.org")
if "WEGLIDE_API_KEY" in os.environ:
configuration.api_key = {"Authorization": os.environ["WEGLIDE_API_KEY"]}
configuration.api_key_prefix = {"Authorization": "Bearer"}
The client provides access to the following WeGlide API endpoints:
For detailed API documentation, visit the WeGlide API Documentation.
import weglide_client
from weglide_client.rest import ApiException
configuration = weglide_client.Configuration()
with weglide_client.ApiClient(configuration) as api_client:
flight_api = weglide_client.FlightApi(api_client)
try:
# Get recent flights
flights = flight_api.get_flights_v1_flight_get(limit=10)
for flight in flights:
print(f"Flight ID: {flight.id}, Pilot: {flight.user.name}, Date: {flight.date}")
except ApiException as e:
print(f"Exception when calling FlightApi: {e}")
import weglide_client
from weglide_client.rest import ApiException
configuration = weglide_client.Configuration()
with weglide_client.ApiClient(configuration) as api_client:
airport_api = weglide_client.AirportApi(api_client)
try:
# Search for airports
airports = airport_api.search_airports_v1_airport_search_get(
query="Munich",
limit=5
)
for airport in airports:
print(f"Airport: {airport.name}, ICAO: {airport.icao}, Elevation: {airport.elevation}m")
except ApiException as e:
print(f"Exception when calling AirportApi: {e}")
Run the tests using unittest:
python -m unittest discover -s test
Or using tox to test across multiple Python versions:
tox
This project is licensed under the MIT License.
FAQs
Python client for the WeGlide API. WeGlide is an online platform where glider pilots can share and analyze their flights, participate in competitions, and connect with the global gliding community.
We found that weglide-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.