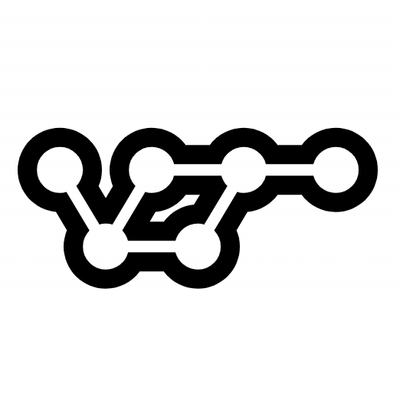
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Python wind parser is a parser used to retrieve arguments for command line interfaces and turn them into python dictionary
Python wind parser is a parser used to retrieve arguments for command line interfaces and turn them into python dictionary.
This parser was created for the speed framework.
Installation with pip :
$ pip install wind-parser
After installing wind-parser you can just instantiate the Parser class with sys.argv
as argument (sys.argv
isn't required but it's better for the code clarity)
Here is an example of how the parser is used:
# command.py
import sys
from wind_parser import Parser
parser = Parser(sys.argv) # This variable stores the arguments with their values in a python dictionary
if __name__ == '__main__':
print(parser) # Print the dictionary
Note : You can also use
p.args
for printing the dictionary
Then you can run in your terminal:
$ python command.py --name=John --age=32 --hobbies Football,Basketball,Cinema --verbose
Output:
{'name':'John', 'age':'32', 'hobbies': ['Football', 'Basketball', 'Cinema'], 'verbose':True}
To access the value of an argument, you can choose between:
print(p['name'])
# or
print(p.args['name'])
print(p.name)
Note : With this method, you will not be able to retrieve arguments with a
-
in its name.
The different types of arguments supported:
-a 1
, --a=1
, -a item1,item2,item3
--verbose
, -v
, --help
Here are the types of the different possible values depending on the type of argument:
Arguments | Python type |
---|---|
--name=John or --age 16 | str |
--verbose or -v | bool (always True) |
--files main.py,m.cfg,test.txt | list[str] |
FAQs
Python wind parser is a parser used to retrieve arguments for command line interfaces and turn them into python dictionary
We found that wind-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.