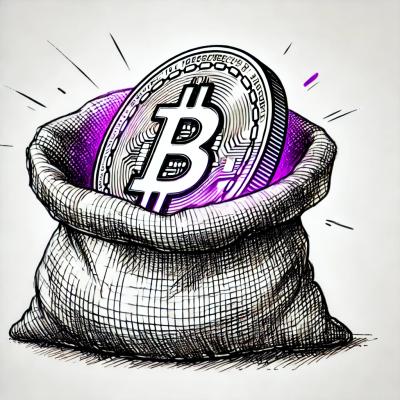
Research
Security News
Malicious npm Packages Use Telegram to Exfiltrate BullX Credentials
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
To install windowstoast
from PyPi run:
pip install windowstoast
from windowstoast import Toast
t = Toast(AppID, NotificationID, ActivationType='protocol', Duration='short', Launch='file:', Scenario='default', Popup=True)
t.show()
#AppID = The application ID from the installed applications in windows. You can get that manually or use my another module 'windowsapps' for that.
#NotificationID = ID you can assign to the notification to identify it later.
#ActivationType = Type of activation for notification. 'system' or 'protocol'.
#Duration = Can be 'short' or 'long'.
#Launch = You can specify your file path to open on notification click, or add your own protocol action.
#Popup = Notification will not pop up if set to false.
t.add_text(text, maxlines=None, attribution=None)
#text = Your text title(If there is only single text) or message(If there is already other text element present).
#maxlines = No. of lines to be occupied by the text.
#attribution = For attribute text at bottom of toast notification
t.add_image(source, placement=None, hint-crop='circle')
#source = Source to the image file
#placement = If specified as 'logo' or 'appLogoOverride' them image will appear as a logo on the left of notification.
#hint-crop = Will make the image circular if specified as 'circle' or squared if specified 'square'.
t.add_audio(source="", silent=False)
#source = Source for the audio file.
#silent = Will make the notification appear silently if set True.
t.add_progress(status, title, value, value_label)
#status = Text below the progress bar indicatiing its current state. Ex- 'Downloading...', 'Receiving...', etc.
#title = Title about the progress bar.
#value = A value between 0 and 1 for the progress.
#value_label = A text below the progress bar showing the current progress to the user. Ex- '26/100 Completed!', '54%', etc.
t.add_content_menu(content, arguments, activationType='protocol')
#content = Name for the context menu.
#argument = Specific argument for the menu.
#activationType = 'protocol' for a protocol activation in windows.
t.add_button(content='Dismiss', argument='Dismiss', activationType='system')
#content = Name for the button.
#argument = Argument specified for the button.
#activationType = Can be specified as 'system' for just dissmisal of notification on button click or set as 'protocol' to launch your custom protocol.
#You can add maximum of 5 buttons.
# First create your own protocol in the windows registry by:
import windowstoast
create_protocol(protocol_uri, comman_target) # Run as Admin required for this!
#protocol_uri = Your own unique protocol uri name. Ex- 'My-Custom-Uri'
#command_target = Path to your own target application with arguments. Ex- r'"Absoulute\Path\To\My\Application.exe" "%1"'
# If you want to remove your protocol:
remove_protocol('Protocol_name') # Run as Admin required for this!
#Create your own Application.exe which takes the argument. Ex-
from sys import argv
a = argv[1:]
print(a)
# Here a is the value of argument that you will specify with the button in Notification.
#Now after making sure you have created your protocol and Application.exe, add button in the notification
t.add_button('My Button','My-Custom-Uri:My_Argument', 'protocol')
# Here you can specify your own protocol uri to execute an action or some other uri like - 'E:\Others\my_file.xyz' to open a file or 'https://www.google.com' to open website.
notificationID = '12345'
t = Toast('My Application ID', notificationID)
t.add_text('Title')
t.add_progress('Status...', 'Progress Title', '0', '0% Completed!')
t.show()
# To update the notification, simply create another notification with same notification id.
for i in range(10):
t = Toast('My Application ID', notificationID, Popup=False) # Set Popup to False whenever you update, otherwise notification will popup each time.
t.add_audio(silent=True) # Set silent to True whenever you update, otherwise audio will play each time.
t.add_text('Title')
t.add_progress('Status...', 'Progress Title', str(i/10), str(i)+'% Completed!')
t.show()
# It is recommended to update notification only after atleast 1.5 seconds.
FAQs
Toast notifications for Windows 11
We found that windowstoast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.
Security News
AI-generated slop reports are making bug bounty triage harder, wasting maintainer time, and straining trust in vulnerability disclosure programs.