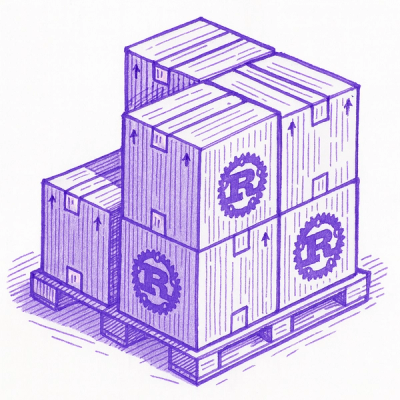
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
A small header-only math library for vectors and matrices.
Build | Status |
---|---|
Ubuntu | |
Windows |
This library is designed to be a potential replacement to various other great
libraries like Eigen
and glm
, but with a narrow focus on 2, 3, and 4
dimensional vectors and matrices. These appear commonly when using spatial
algebra in robotics, which is the main application area this library was
initially developed for.
Clone this package into your third_party
dependencies:
# Replace "third_party" with your own dependencies-folder name
git clone https://github.com/wpumacay/math3d.git third_party/math3d
There's a CMake
target called math::math
. Just add the source directory in
your CMake
workflow, and use the given target as follows:
# Add the Math3d subdirectory
add(third_party/math3d)
# Link against the exposed math::math target
target_link_library(MY_LIBRARY PRIVATE math::math)
Use the provided setup.py
file:
python setup.py install
And import the types from the math3d
package:
import math3d as m3d
#include <vec3_t.h>
#include <mat3_t.h>
int main()
{
// Create a vec3-float32 and show it on the console
::math::Vector3f vec = { 1.0f, 2.0f, 3.0f };
std::cout << "vec: " << vec << std::endl;
// Create a mat3 float32, show its entries and its inverse
auto mat = ::math::Matrix3f( 3.0f, 9.0f, 3.0f,
9.0f, 0.0f, 3.0f,
2.0f, 3.0f, 8.0f );
std::cout << "mat:" << std::endl;
std::cout << mat << std::endl;
std::cout << "mat.inverse():" << std::endl;
std::cout << ::math::inverse( mat ) << std::endl;
return 0;
}
import numpy as np
from math3d import Vector3f, Matrix3f
# Create a vec3-float32 and show it on the console
vec = Vector3f(np.array([1.0, 2.0, 3.0], dtype=np.float32))
print(vec)
# Create a mat3 float32, show its entries and its inverse
mat = Matrix3f(np.array([[ 3.0, 9.0, 3.0 ],
[ 9.0, 0.0, 3.0 ],
[ 2.0, 3.0, 8.0 ]], dtype=np.float32))
print(mat)
print("inverse(): \n\r{}".format(mat.inverse()))
FAQs
A basic math library for spatial algebra
We found that wp-math3d demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.