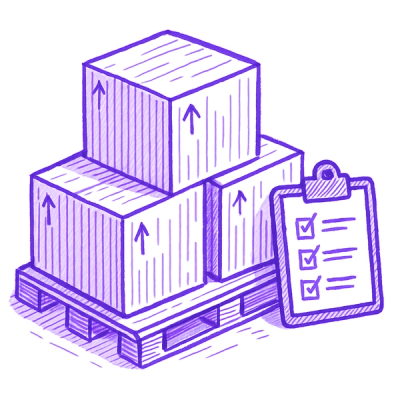
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
SQLite-powered document database with MongoDB-like syntax, full-text search, and advanced querying capabilities
A high-performance document database built on SQLite with MongoDB-like syntax. ZenithDB combines the simplicity and reliability of SQLite with the flexibility of document databases.
ZenithDB is a powerful, lightweight document database that provides a MongoDB-like experience while leveraging SQLite as the storage engine. It's perfect for:
pip install zenithdb
from zenithdb import Database
# Initialize database
db = Database("myapp.db")
users = db.collection("users")
# Add document validation
def age_validator(doc):
return isinstance(doc.get('age'), int) and doc['age'] >= 0
users.set_validator(age_validator)
# Insert documents
users.insert({
"name": "John Doe",
"age": 30,
"tags": ["premium"],
"profile": {"city": "New York"}
})
# Query documents
users.find({
"age": {"$gt": 25},
"tags": {"$contains": "premium"}
})
# Full-text search
users.find({"*": {"$contains": "John"}})
# Nested updates
users.update(
{"name": "John Doe"},
{"$set": {
"profile.city": "Brooklyn",
"tags.0": "vip"
}}
)
# Aggregations
users.aggregate([{
"group": {
"field": "profile.city",
"function": "COUNT",
"alias": "count"
}
}])
# List and count collections
db.list_collections()
db.count_collections()
# Drop collections
db.drop_collection("users")
db.drop_all_collections()
# Print collection contents
users.print_collection()
users.count()
ZenithDB supports two querying styles:
# Find documents with comparison operators
users.find({
"age": {"$gt": 25, "$lte": 50},
"profile.city": "New York",
"tags": {"$contains": "premium"}
})
# Full-text search across all fields
results = users.find({"*": {"$contains": "search term"}})
# Pattern matching with regex, starts with, and ends with
users.find({
"email": {"$regex": "^[a-z0-9]+@example\\.com$"},
"name": {"$startsWith": "Jo"},
"domain": {"$endsWith": ".org"}
})
from zenithdb import Query
q = Query()
results = users.find(
(q.age > 25) &
(q.age <= 50) &
(q.profile.city == "New York") &
q.tags.contains("premium")
)
# Pattern matching with Query builder
results = users.find(
q.email.regex("^[a-z0-9]+@example\\.com$") &
q.name.starts_with("Jo") &
q.domain.ends_with(".org")
)
# With sorting and pagination
q = Query(collection="users", database=db)
q.sort("age", ascending=False)
q.limit(10).skip(20) # Page 3 with 10 items per page
results = q.execute()
# Create single field index
db.create_index("users", "email")
# Create compound index
db.create_index("users", ["profile.city", "age"])
# Create unique index
db.create_index("users", "username", unique=True)
# Create full-text search index for efficient text searching
db.create_index("users", ["name", "bio", "tags"], full_text=True)
# Perform efficient text search using the FTS index
users.search_text("python developer")
# Search specific fields only
users.search_text("developer", fields=["bio"])
# List indexes and drop index
db.list_indexes("users")
db.drop_index("idx_users_email")
db.drop_index("fts_users_name_bio_tags") # Drop a full-text search index
# Get bulk operations interface
bulk_ops = users.bulk_operations()
# Use transaction for atomic operations
with bulk_ops.transaction():
# Bulk insert
ids = bulk_ops.bulk_insert("users", [
{"name": "User1", "age": 31},
{"name": "User2", "age": 32}
])
# Bulk update
bulk_ops.bulk_update("users", [
{"_id": ids[0], "status": "active"},
{"_id": ids[1], "status": "inactive"}
])
from zenithdb.migrations import MigrationManager
# Initialize migration manager
manager = MigrationManager(db)
# Create migration
migration = {
'version': '001',
'name': 'add_users',
'up': lambda: db.collection('users').insert({'admin': True}),
'down': lambda: db.collection('users').delete({})
}
# Apply migration
manager.apply_migration(migration)
# Get current version
current_version = manager.get_current_version()
# Backup the database to a file
db.backup("backup_2025_02_27.db")
# Restore from a backup file
db.restore("backup_2025_02_27.db")
ZenithDB 2.0 includes advanced aggregation functions beyond basic COUNT, SUM, and AVG:
from zenithdb import AggregateFunction
# Calculate median age by country
median_age = users.aggregate([{
"group": {
"field": "profile.location.country",
"function": AggregateFunction.MEDIAN,
"target": "age",
"alias": "median_age"
}
}])
# Calculate standard deviation of salaries
salary_stddev = users.aggregate([{
"group": {
"field": "department",
"function": AggregateFunction.STDDEV,
"target": "salary",
"alias": "salary_stddev"
}
}])
# Count distinct values
distinct_cities = users.aggregate([{
"group": {
"field": "profile.location.country",
"function": AggregateFunction.COUNT_DISTINCT,
"target": "profile.location.city",
"alias": "unique_cities"
}
}])
ZenithDB includes tools for monitoring and optimizing query performance:
# Initialize with debugging for query plan analysis
db = Database("myapp.db", debug=True)
# Create appropriate indexes
db.create_index("users", ["age", "status"])
# Run query to see if indexes are used
users.find({"age": {"$gt": 25}, "status": "active"})
# Output: ✓ Using index: idx_users_age_status
# Install development dependencies
pip install -e ".[dev]"
# Run tests
pytest tests/
pytest tests/test_migrations.py
pytest --cov=zenithdb tests/
Version 2.0 of ZenithDB introduces several improvements with minimal breaking changes:
To migrate from v1.x:
pip install --upgrade zenithdb
Feature | ZenithDB | SQLite | MongoDB | TinyDB |
---|---|---|---|---|
Document Storage | ✅ | ❌ (requires JSON) | ✅ | ✅ |
Nested Queries | ✅ | ❌ (limited) | ✅ | ✅ |
Full-text Search | ✅ | ❌ (needs extension) | ✅ | ❌ |
Indexing | ✅ | ✅ | ✅ | ❌ |
Transactions | ✅ | ✅ | ✅ | ❌ |
Zero Dependencies | ✅ | ✅ | ❌ | ✅ |
Server Required | ❌ | ❌ | ✅ | ❌ |
Embedded Use | ✅ | ✅ | ❌ | ✅ |
This project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! For feature requests, bug reports, or code contributions, please open an issue or pull request on GitHub.
For complete examples of all features, check out usage.py.
Note: ZenithDB is primarily a learning and development tool. While it's suitable for small to medium applications, it's not recommended as a production database for high-traffic or mission-critical systems.
FAQs
SQLite-powered document database with MongoDB-like syntax, full-text search, and advanced querying capabilities
We found that zenithdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.