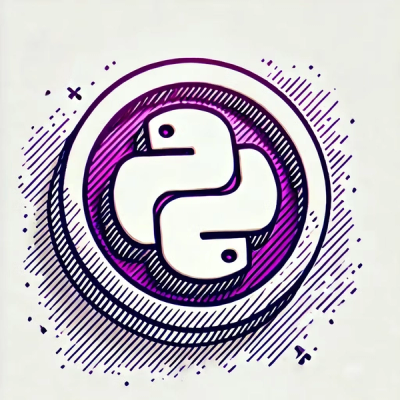
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
A couple of classes that represent useful control flow patterns (conditional loops, pipelines etc).
In pseudo-funtional programming.
Add this line to your application's Gemfile:
gem 'bsflow'
And then execute:
$ bundle
Or install it yourself as:
$ gem install bsflow
Reqiure proper class.
require "bsflow/pipeline"
Use it.
square_number_generator = BSFlow:Pipeline.new(procs: [random_int, square])
All classes have just one public method: #call.
Some classes have dependencies (injected in constructor) called "procs". They are objects that respond to #call method.
It passes a value through every proc and returns a final value. Output of the previous proc is input of the next proc.
Source code:
module BSFlow
class Pipeline
def initialize(*args, procs: [])
@procs = args + procs
end
def call(*args)
output = @procs[0].call(*args)
if @procs.length == 1
return output
else
@procs[1..-1].each do |proc|
output = proc.call(output)
end
output
end
end
end
end
require "bsflow/pipeline"
BSFlow:Pipeline.new(procs: procs) # => new_pipeline
# or
BSFlow:Pipeline.new(*procs) # => new_pipeline
# or
BSFlow:Pipeline.new(*first_part_of_procs, procs: rest_of_procs) # => new_pipeline
Paramaters:
.call
message. The first proc takes the "main" input of the class (any number of arguments). The result is passed to the next proc as input. The output of the last proc is the output of .call
method of Pipeline class.
The procs can be passed as a normal arguments or as a list argumentsIt allows to "connect" standard output stream to an object that expects #call
mathod.
Source code:
module BSFlow
class StdoutAdapter
def initialize(stdout:)
@stdout = stdout
end
def call(string)
@stdout.puts(string)
end
end
end
require "bsflow/stdout_adapter"
BSFlow:StdoutAdapter.new(procs: procs) # => new_adapter
Paramaters:
.#puts
message with one argument.It allows to "connect" standard input stream to an object that expects #call
mathod.
Source code:
module BSFlow
class StdinAdapter
def initialize(stdin:)
@stdin = stdin
end
def call()
@stdin.gets.chomp
end
end
end
require "bsflow/stdin_adapter"
BSFlow:StdinAdapter.new(stdin: stdin) # => new_adapter
Paramaters:
.#gets
message. The chomped output of this object is the output of .call
method of StdinAdapter class.It passes a value to [] method of a hash or any objects that response to [] method.
Source code:
module BSFlow
class SquareBracketsAdapter
def initialize(map:)
@map = map
end
def call(symbol)
@map[symbol]
end
end
end
require "bsflow/square_brackets_adapter"
BSFlow:SquareBracketsAdapter.new(map: map) # => new_square_brackets_adapter
Paramaters:
.#[]
message. It takes the "main" input of the class (one argument). The output of this object is the output of .call
method of SquareBracketsAdapter class.It just returns first pased argument and ignores the rest. Used to reduce number of arguments.
Source code:
module BSFlow
class FirstArg
def call(*args)
args.first
end
end
end
require "bsflow/first_arg"
BSFlow:FirstArg.new # => new_first_arg
It just returns last pased argument and ignores the rest. Used to reduce number of arguments.
Source code:
module BSFlow
class LastArg
def call(*args)
args.last
end
end
end
require "bsflow/last_arg"
BSFlow:LastArg.new # => new_last_arg
It returns unmodified argument.
Source code:
module BSFlow
class Self
def call(input)
input
end
end
end
require "bsflow/self"
BSFlow:Self.new # => new_self
It returns true no matter what input it takes.
Source code:
module BSFlow
class True
def call(input)
true
end
end
end
require "bsflow/true"
BSFlow:True.new # => new_true
It returns false no matter what input it takes.
Source code:
module BSFlow
class False
def call(input)
false
end
end
end
require "bsflow/false"
BSFlow:False.new # => new_false
It passes a value to a proc and returns the unmodified value. So it is a one way ticket for data but it is usefull for sending data to some output stream or queue.
Source code:
module BSFlow
class Pass
def initialize(proc:)
@proc = proc
end
def call(input)
@proc.call(input)
input
end
end
end
require "bsflow/pass"
BSFlow:Pass.new(proc: proc) # => new_pass
Paramaters:
.call
message with one argument.It calls a proc and returns its output. All arguments are ignored.
Source code:
module BSFlow
class DropArgs
def initialize(proc:)
@proc = proc
end
def call(*args)
@proc.call
end
end
end
require "bsflow/drop_args"
BSFlow:DropArgs.new(proc: proc) # => new_args_dropper
Paramaters:
.call
message without arguments.It passes its .call
arguments to each injected sub_proc, then it passes their outputs to injected combine_proc and returns the output.
Source code:
module BSFlow
class Combine
def initialize(*args, combine_proc: nil, sub_procs: [])
if combine_proc
@sub_procs = sub_procs
@combine_proc = combine_proc
else
@sub_procs = args[0..-2]
@combine_proc = args.last
end
end
def call(*args)
sub_proc_outputs = []
@sub_procs.each do |sub_proc|
sub_proc_outputs << sub_proc.call(*args)
end
@combine_proc.call(*sub_proc_outputs)
end
end
end
require "bsflow/combine"
BSFlow::Combine.new(sub_procs: sub_procs, combine_proc: combine_proc) # => new_combine
# or
BSFlow::Combine.new(*sub_procs, combine_proc) # => new_combine
Paramaters:
.call
message. Each of them takes arguments from combine object's call method and return an output. All aoutpus are pased to combine_proc..call
message. The output of this proc is the output of the .call
method of the Combine class.It passes input to condition_proc and if the result is not true it pases the input to loop_proc until the result is true.
Source code:
module BSFlow
class UntilTrueLoop
def initialize(condition_proc:, loop_proc:)
@loop_proc = loop_proc
@condition_proc = condition_proc
end
def call(input)
until @condition_proc.call(input) do
input = @loop_proc.call(input)
end
input
end
end
end
require "bsflow/until_true_loop"
BSFlow::UntilTrueLoop.new(condition_proc: condition_proc, loop_proc: loop_proc) # => new_loop
Paramaters:
.call
message with one argument. At the beginning it takes the input from .call
method from UntilTrueLoop class. If an output is truthy the output is returned as the output of .call
message of UntilTrueLoop class..call
message with one argument.The gem is available as open source under the terms of the MIT License.
FAQs
Unknown package
We found that bsflow demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.