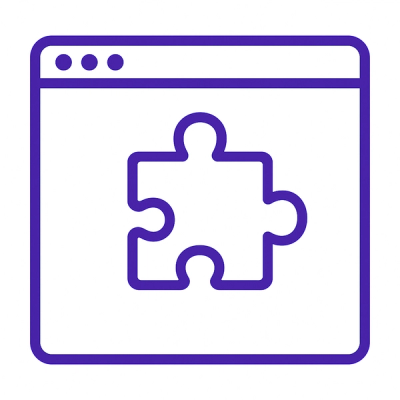
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
A simple wrapper for the OpenSSL Cipher library for Ruby and Rails applications. This gem provides you with an easy way to encrypt and decrypt any data using both symmetrical and asymmetrical algorithms.
Full documentation can be found in the Wiki section of the repo.
Run this command
gem install encryption
or add this line to your Gemfile
gem "encryption"
A simple example of how the gem works:
Encryption.key = "Secretly yours,\n very long encryption key"
data = "this is to stay secret"
encrypted_str = Encryption.encrypt(data)
Encryption.decrypt(encrypted_str) == data # true
Sometimes it is useful to use an own instance with custom settings, rather than the global Encryption instance. Here is how you can achieve it.
encryptor = Encryption::Symmetric.new
encryptor.key = "Secretly yours,\n very long encryption key"
data = "this is to stay secret"
encrypted_str = encryptor.encrypt(data)
encryptor.decrypt(encrypted_str) == data # true
For symmetric encryption / decryption you need to set an encryption key. The rest of the settings are optional. Here is a list of all of them:
Encryption.key
- Your encryption key
Encryption.iv # Optional
- Encryption initialization vector. Defaults to the character "\0"
(Optional)
Encryption.cipher # Optional
- Your encryption algorithm. Defaults to aes-256-cbc
(Optional)
Run openssl list-cipher-commands
in the terminal to list all installed ciphers or call OpenSSL::Cipher.ciphers
in Ruby, which will return an array, containing all available algorithms.
You can optionally configure both a global instance and a custom instance with a block:
Encryption.config do |config|
config.key = "don't look at me!"
config.iv = "is there a better way to initialize OpenSSL?"
config.cipher = "camellia-128-ecb" # if you feel adventurous
end
The encryption
gem also provides easier syntax for asymmetric encryption.
keypair = Encryption::Keypair.new # Accepts two optional arguments size = 2048 and password = nil
keypair.public_key # Instance of Encryption::PublicKey
keypair.private_key # Instance of Encryption::PrivateKey
# Or this for short
public_key, private_key = Encryption::Keypair.generate(2048)
# Then you can export each to string
private_key.to_s
# or to PEM format
private_key.to_pem
# and optionally encrypt is with a passphrase
private_key.to_pem('passphrase')
Encryption::PublicKey
and Encryption::PrivateKey
Both classes have the same methods
# Import an existing key
Encryption::PublicKey.new(filename[, password]) # From file
Encryption::PublicKey.new(string[, password]) # From string
# Encrypt / Decrypt data
public_key = Encryption::PublicKey.new("existing key")
public_key.encrypt("2001: A Space Odyssey")
public_key.decrypt("H3LL0¡")
# Note that you can use both public and private keys to encrypt and decrypt data
The gem adds the encrypt
and decrypt
methods to the String
class.
You can use them as follows:
# With the global Encryption instance
"Hello".encrypt
"Hello".encrypt!
"h3LL0".decrypt
"h3LL0".decrypt!
# With custom settings (and custom encryptor instance)
"Contact".encrypt(key: 'encryption key', iv: 'initialization vector', cipher: 'encryption algorithm', encode: true)
# Note the encode option which will result in a base64 encoded string
"3NcPyptED".decrypt!(encoded: true) # Will decrypt an encoded string
# Or with a custom encryptor
encryptor = Encryption::Symmetric.new
encryptor.key = 'random string'
"Interstate 60".encrypt(encryptor: encryptor)
This gem is distributed under The MIT License.
Itay Grudev <itay()grudev...com>
FAQs
Unknown package
We found that encryption demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.