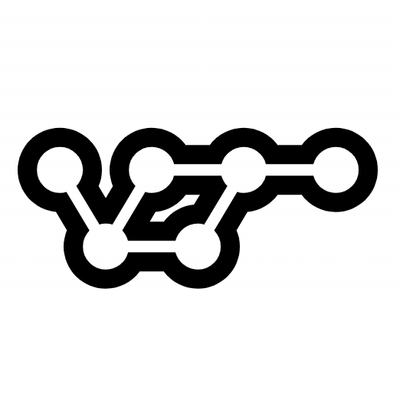
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
This is a Ruby client for Replicate. It lets you run models from your Ruby code and do various other things on Replicate.
Add this line to your application's Gemfile:
gem 'replicate-ruby'
Grab your token from replicate.com/account and authenticate by configuring api_token
:
Replicate.configure do |config|
config.api_token = "your_api_token"
end
You can retrieve a model:
# Latest version
model = Replicate.client.retrieve_model("stability-ai/stable-diffusion")
version = model.latest_version
# List of versions
version = Replicate.client.retrieve_model("stability-ai/stable-diffusion", version: :all)
# Specific version
version = Replicate.client.retrieve_model("stability-ai/stable-diffusion", version: "<id>")
And then run predictions on it:
prediction = version.predict(prompt: "a handsome teddy bear")
# manually refetch the prediction status
prediction = prediction.refetch
# or cancel a running prediction
prediction = prediction.cancel
# and if a prediction returns with status succeeded, you can retrieve the output
output = prediction.output
# Optionally you can submit a webhook url for replicate to send a POST request once a prediction has completed
prediction = version.predict(prompt: "a handsome teddy bear", "https://webhook.url/path") # call predict
id = prediction.id # store prediction id in your backend
prediction = Replicate.client.retrieve_prediction(id) # retrieve prediction during webhook with id from backend
There is support for the experimental dreambooth endpoint.
First, upload your training dataset:
upload = Replicate.client.upload_zip('tmp/data.zip') # replace with the path to your zip file
Then start training a new model using, for instance:
training = Replicate.client.create_training(
input: {
instance_prompt: "zwx style",
class_prompt: "style",
instance_data: upload.serving_url,
max_train_steps: 5000
},
model: 'yourusername/yourmodel'
)
As soon as the model has finished training, you can run predictions on it:
prediction = Replicate.client.create_prediction(
input: {
prompt: 'your prompt, zwx style'
},
version: training.version
)
You can also download the output.zip
file from the dreambooth training prediction, unzip it, and then convert the trained model to a Stable Diffusion checkpoint with convert_diffusers_to_sd.py.
python ./convert_diffusers_to_sd.py --model_path ~/Downloads/output --checkpoint_path ~/Downloads/output.ckpt
After checking out the repo, run bin/setup
to install dependencies. Then, run rake test
to run the tests. You can also run bin/console
for an interactive prompt that will allow you to experiment.
FAQs
Unknown package
We found that replicate-ruby demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.