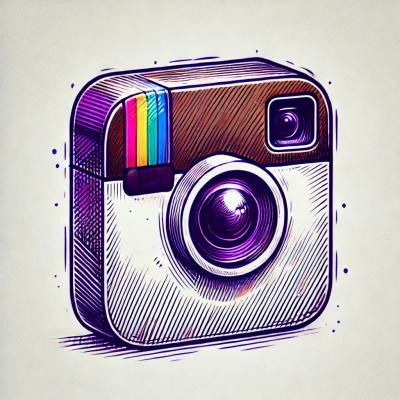
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Ruby-TLS decouples the management of encrypted communications, putting you in charge of the transport layer. It can be used as an alternative to Ruby's SSLSocket.
Install it with RubyGems
gem install ruby-tls
or add this to your Gemfile if you use Bundler:
gem "ruby-tls"
Windows users will require an installation of OpenSSL (32bit or 64bit matching the Ruby installation)
require 'rubygems'
require 'ruby-tls'
class transport
def initialize
is_server = true
callback_obj = self
options = {
verify_peer: true,
private_key: '/file/path.pem',
cert_chain: '/file/path.crt',
ciphers: 'ECDHE-RSA-AES128-GCM-SHA256:ECDHE-RSA-RC4-SHA:ECDHE-RSA-AES128-SHA:AES128-GCM-SHA256:RC4:HIGH:!MD5:!aNULL:!EDH:!CAMELLIA:@STRENGTH' # (default)
# protocols: ["h2", "http/1.1"], # Can be used where OpenSSL >= 1.0.2 (Application Level Protocol negotiation)
# fallback: "http/1.1", # Optional fallback to a default protocol when either client or server doesn't support ALPN
# client_ca: '/file/path.pem'
}
@ssl_layer = RubyTls::SSL::Box.new(is_server, callback_obj, options)
end
def close_cb
puts "The transport layer should be shutdown"
end
def dispatch_cb(data)
puts "Clear text data that has been decrypted"
end
def transmit_cb(data)
puts "Encrypted data for transmission to remote"
# @tcp.send data
end
def handshake_cb(protocol)
puts "initial handshake has completed"
end
def verify_cb(cert)
# Return true or false
is_cert_valid? cert
end
def start_tls
# Start SSL negotiation when you are ready
@ssl_layer.start
end
def send(data)
@ssl_layer.encrypt(data)
end
end
#
# Create a new TLS connection
#
connection = transport.new
#
# Init the handshake
#
connection.start_tls
#
# Start sending data to the remote, this will trigger the
# transmit_cb with encrypted data to send.
#
connection.send('client request')
#
# Similarly when data is received from the remote it should be
# passed to connection.decrypt where the dispatch_cb will be
# called with clear text
#
MIT
FAQs
Unknown package
We found that ruby-tls demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.