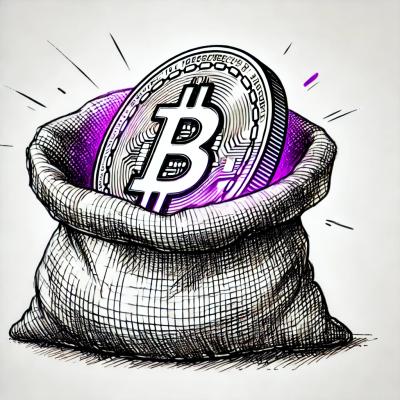
Research
Security News
Malicious npm Packages Use Telegram to Exfiltrate BullX Credentials
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
You can use Spotify's Web API to discover music and podcasts, manage your Spotify library, control audio playback, and much more. Browse our available Web API endpoints using the sidebar at left, or via the navigation bar on top of this page on smaller screens.
In order to make successful Web API requests your app will need a valid access token. One can be obtained through OAuth 2.0.
The base URI for all Web API requests is https://api.spotify.com/v1
.
Need help? See our Web API guides for more information, or visit the Spotify for Developers community forum to ask questions and connect with other developers.
Install the gem from the command line:
gem install spotify-api-sdk -v 1.0.0
Or add the gem to your Gemfile and run bundle
:
gem 'spotify-api-sdk', '1.0.0'
For additional gem details, see the RubyGems page for the spotify-api-sdk gem.
Note: Documentation for the client can be found here.
The following parameters are configurable for the API Client:
Parameter | Type | Description |
---|---|---|
environment | Environment | The API environment. Default: Environment.PRODUCTION |
connection | Faraday::Connection | The Faraday connection object passed by the SDK user for making requests |
adapter | Faraday::Adapter | The Faraday adapter object passed by the SDK user for performing http requests |
timeout | Float | The value to use for connection timeout. Default: 60 |
max_retries | Integer | The number of times to retry an endpoint call if it fails. Default: 0 |
retry_interval | Float | Pause in seconds between retries. Default: 1 |
backoff_factor | Float | The amount to multiply each successive retry's interval amount by in order to provide backoff. Default: 2 |
retry_statuses | Array | A list of HTTP statuses to retry. Default: [408, 413, 429, 500, 502, 503, 504, 521, 522, 524] |
retry_methods | Array | A list of HTTP methods to retry. Default: %i[get put] |
http_callback | HttpCallBack | The Http CallBack allows defining callables for pre and post API calls. |
authorization_code_auth_credentials | AuthorizationCodeAuthCredentials | The credential object for OAuth 2 Authorization Code Grant |
The API client can be initialized as follows:
client = SpotifyWebApi::Client.new(
authorization_code_auth_credentials: AuthorizationCodeAuthCredentials.new(
o_auth_client_id: 'OAuthClientId',
o_auth_client_secret: 'OAuthClientSecret',
o_auth_redirect_uri: 'OAuthRedirectUri',
o_auth_scopes: [
OAuthScopeEnum::APP_REMOTE_CONTROL,
OAuthScopeEnum::PLAYLIST_READ_PRIVATE
]
),
environment: Environment::PRODUCTION
)
API calls return an ApiResponse
object that includes the following fields:
Field | Description |
---|---|
status_code | Status code of the HTTP response |
reason_phrase | Reason phrase of the HTTP response |
headers | Headers of the HTTP response as a Hash |
raw_body | The body of the HTTP response as a String |
request | HTTP request info |
errors | Errors, if they exist |
data | The deserialized body of the HTTP response |
This API uses the following authentication schemes.
FAQs
Unknown package
We found that spotify-api-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.
Security News
AI-generated slop reports are making bug bounty triage harder, wasting maintainer time, and straining trust in vulnerability disclosure programs.