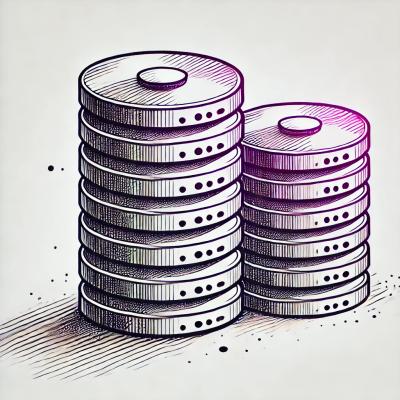
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
A Ruby library for interaction with the Tropo Web API (http://tropo.com) using JSON.
The Tropo Remote API provides a RESTful JSON API for controlling realtime communications applications from your own web servers.
Note: If using with ActiveSupport, v2.3.5 or better of ActiveSupport is required.
$ sudo gem install tropo-webapi-ruby
Optional, if you would like to use with Sinatra:
$ sudo gem install sinatra
$ gemserver
$ sudo gem install yard
From within the project:
$ yardoc
require 'rubygems'
require 'tropo-webapi-ruby'
# Will return the properly formatted JSON to pass to Tropo
response = Tropo::Generator.ask({ :say => 'Hello world!' })
# Will return a Ruby Hash, with some transformations, from the JSON string received from Tropo
response = Tropo::Generator.parse(json_string)
# Will provide instance variables that will allow you to easily reference session type
tropo = Tropo::Generator.new
response = tropo.parse(json_string)
p 'Hey, this is a voice session!' if tropo.voice_session
p 'Hey, this is a text messaging session!' if tropo.text_session
Using the great RESTful Web Services framework Sinatra for Ruby.
require 'rubygems'
require 'sinatra'
require 'tropo-webapi-ruby'
post '/helloworld.json' do
Tropo::Generator.say 'Hello World!'
end
post '/helloworld_block.json' do
tropo = Tropo::Generator.new do
say 'Hello World!'
end
tropo.response
end
post '/helloworld_twice.json' do
tropo = Tropo::Generator.new
tropo.say 'Hello World!'
tropo.say 'Hello again.'
tropo.response
end
# Produces a Ruby hash:
#
# { :session =>
# { :timestamp => Tue Jan 19 18:27:46 -0500 2010,
# :user_type =>"HUMAN",
# :initial_text => nil,
# :account_id =>"0",
# :headers => [{ "value" => "70", "key"=>"Max-Forwards" },
# { "value" => "385", "key"=>"Content-Length" },
# { "value" => "<sip:127.0.0.1:49152>", "key"=>"Contact" },
# { "value" => "replaces", "key"=>"Supported" },
# { "value" => "<sip:sample.json@localhost:5555>", "key"=>"To" },
# { "value" => "1 INVITE", "key"=>"CSeq" },
# { "value" => "SJphone-M/1.65.382f (SJ Labs)", "key"=>"User-Agent" },
# { "value" => "SIP/2.0/UDP 127.0.0.1:49152;branch=z9000000a9;rport=49152", "key"=>"Via" },
# { "value" => "3FA7C70A1DD211B286B7A583D7B46DDD0xac106207", "key"=>"Call-ID" },
# { "value" => "application/sdp", "key"=>"Content-Type" },
# { "value" => "unknown <sip:127.0.0.1:49152>;tag=750b1b1648e9c876", "key"=>"From" }],
# :id => "3FA7C70A1DD211B286B7A583D7B46DDD0xac106207",
# :to => { :network => "PSTN",
# :channel => "VOICE",
# :name => "unknown",
# :id => "sample.json"},
# :from => { :network => "PSTN",
# :channel => "VOICE",
# :name => "unknown",
# :id => "unknown"}}}
#
post '/start_session.json' do
tropo_session = Tropo::Generator.parse request.env["rack.input"].read
p tropo_session
end
post '/ask.json' do
tropo = Tropo::Generator.new do
on :event => 'hangup', :next => '/hangup.json'
on :event => 'continue', :next => '/answer.json'
ask({ :name => 'account_number',
:bargein => true,
:timeout => 30,
:require => 'true' }) do
say :value => 'Please say your account number'
choices :value => '[5 DIGITS]'
end
end
tropo.response
end
# Produces a Ruby hash, if the user gives a response before hanging up:
#
# { :result =>
# { :actions => { :attempts => 1,
# :disposition => "SUCCESS",
# :interpretation => "12345",
# :confidence => 100,
# :name => "account_number",
# :utterance => "1 2 3 4 5" },
# :session_duration => 3,
# :error => nil,
# :sequence => 1,
# :session_id => "5325C262-1DD2-11B2-8F5B-C16F64C1D62E@127.0.0.1",
# :state => "ANSWERED",
# :complete => true } }
#
post '/answer.json' do
tropo_event = Tropo::Generator.parse request.env["rack.input"].read
p tropo_event
end
# Produces a Ruby hash, if the user hangs up before giving a reponse
# { :result =>
# { :actions => {},
# :session_duration => 1,
# :error => nil,
# :sequence => 1,
# :session_id => "812BEF50-1DD2-11B2-8F5B-C16F64C1D62E@127.0.0.1",
# :state => "DISCONNECTED",
# :complete => true } }
#
post '/hangup.json' do
tropo_event = Tropo::Generator.parse request.env["rack.input"].read
p tropo_event
end
# A redirect method
post '/redirect.json' do
Tropo::Generator.redirect({ :to => 'sip:1234', :from => '4155551212' })
end
# A reject method
post '/reject.json' do
Tropo::Generator.reject
end
post '/speak.json' do
t = Tropo::Generator.new(:voice => 'kate')
t.say 'Hello!' # Will speak as kate now
# or
t = Tropo::Generator.new
t.voice = 'kate'
t.say 'Hello!' # Will speak as kate now
end
post '/ask.json' do
t = Tropo::Generator.new(:recognizer => 'fr-fr')
t.ask({ :name => 'account_number', # Will now use the French speech recognition engine
:bargein => true,
:timeout => 30,
:require => 'true' }) do
say :value => "S'il vous plaît dire votre numéro de compte", :voice => 'florence'
choices :value => '[5 DIGITS]'
end
# or
t = Tropo::Generator.new
t.recognizer = 'fr-fr'
t.ask({ :name => 'account_number', # Will now use the French speech recognition engine
:bargein => true,
:timeout => 30,
:require => 'true' }) do
say :value => "S'il vous plaît dire votre numéro de compte", :voice => 'florence'
choices :value => '[5 DIGITS]'
end
end
May be found by checking out the project from Github, and then looking in $PROJECT_HOME/examples and $PROJECT_HOME/spec/tropo-webapi-ruby_spec.rb.
http://voxeo.github.com/tropo-webapi-ruby
http://docs.tropo.com/webapi/2.0/home.htm
In order to maintain compatibility between Ruby MRI and JRuby the gem requires 'json_pure'. If you are using the Ruby MRI and would prefer to use the C version of 'json', simply install the 'json' gem as follows:
sudo gem install json
Copyright (c) 2014 Tropo, Inc. See LICENSE for details.
FAQs
Unknown package
We found that tropo-webapi-ruby demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.