promptui
Interactive prompt for command-line applications.
We built Promptui because we wanted to make it easy and fun to explore cloud
services with manifold cli.
Code of Conduct |
Contribution Guidelines

Overview
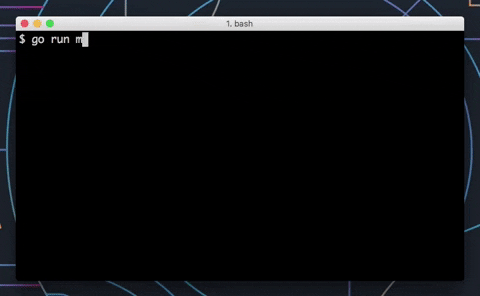
Promptui is a library providing a simple interface to create command-line
prompts for go. It can be easily integrated into
spf13/cobra,
urfave/cli or any cli go application.
Promptui has two main input modes:
-
Prompt
provides a single line for user input. Prompt supports
optional live validation, confirmation and masking the input.
-
Select
provides a list of options to choose from. Select supports
pagination, search, detailed view and custom templates.
For a full list of options check GoDoc.
Basic Usage
Prompt
package main
import (
"errors"
"fmt"
"strconv"
"github.com/manifoldco/promptui"
)
func main() {
validate := func(input string) error {
_, err := strconv.ParseFloat(input, 64)
if err != nil {
return errors.New("Invalid number")
}
return nil
}
prompt := promptui.Prompt{
Label: "Number",
Validate: validate,
}
result, err := prompt.Run()
if err != nil {
fmt.Printf("Prompt failed %v\n", err)
return
}
fmt.Printf("You choose %q\n", result)
}
Select
package main
import (
"fmt"
"github.com/manifoldco/promptui"
)
func main() {
prompt := promptui.Select{
Label: "Select Day",
Items: []string{"Monday", "Tuesday", "Wednesday", "Thursday", "Friday",
"Saturday", "Sunday"},
}
_, result, err := prompt.Run()
if err != nil {
fmt.Printf("Prompt failed %v\n", err)
return
}
fmt.Printf("You choose %q\n", result)
}
More Examples
See full list of examples