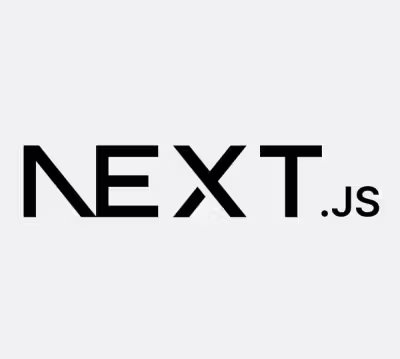
Security News
Next.js Patches Critical Middleware Vulnerability (CVE-2025-29927)
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
github.com/areknoster/hypert
Hypert is an open-source Go library that simplifies testing of HTTP API clients. It provides a convenient way to record and replay HTTP interactions, making it easy to create reliable and maintainable tests for your API clients.
http.Client
go get github.com/areknoster/hypert
hypert.TestClient
to create an http.Client
instance for testing:func TestMyAPI(t *testing.T) {
httpClient := hypert.TestClient(t, true) // true to record real requests
// Use the client to make API requests.
// The requests and responses would be stored in ./testdata/TestMyAPI
myAPI := NewMyAPI(httpClient, os.GetEnv("API_SECRET"))
// Make an API request with your adapter.
// use static arguments, so that validation against recorded requests can happen
stuff, err := myAPI.GetStuff(time.Date(2022, 1, 1, 0, 0, 0, 0, time.UTC))
if err != nil {
t.Fatalf("failed to get stuff: %v", err)
}
// Assertions on the actual API response
if stuff.ID != "ID-FROM-RESP" {
t.Errorf("stuff ")
}
}
After you're done with building and testing your integration, change the mode to replay
func TestMyAPI(t *testing.T) {
httpClient := hypert.TestClient(t, false) // false to replay stored requests
// Now client would validate requests against what's stored in ./testdata/TestMyAPI/*.req.http
// and load the response from ./testdata/TestMyAPI/*.resp.http
myAPI := NewMyAPI(httpClient, os.GetEnv("API_SECRET"))
// HTTP requests are validated against what was prevously recorded.
// This behaviour can be customized using WithRequestValidator option
stuff, err := myAPI.GetStuff(time.Date(2022, 1, 1, 0, 0, 0, 0, time.UTC))
if err != nil {
t.Fatalf("failed to get stuff: %v", err)
}
// Same assertions that were true on actual API responses should be true for replayed API responses.
if stuff.ID != "ID-FROM-RESP" {
t.Errorf("stuff ")
}
}
Now your tests:
I plan to maintain backward compatibility as much as possible, but breaking changes may occur before the first stable release, v1.0.0 if major issues are discovered.
Check out the examples directory for sample usage of Hypert in different scenarios.
Contributions are welcome! If you find a bug or have a feature request, please open an issue on the GitHub repository. If you'd like to contribute code, please fork the repository and submit a pull request.
Hypert is released under the MIT License.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.