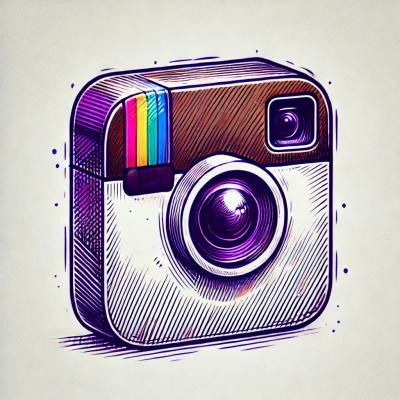
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
github.com/vladcto/yandex-gpt-rest-api
Dart library for working with YandexGPT API.
Create YandexGptApi
instance.
// For passing BaseOptions or Dio use other constructors.
final api = YandexGptApi(
token: AuthToken.api("your_token"), // or AuthToken.iam
// Not necessary, by default uses catalog from AuthToken account.
catalog: "catalog_id?",
);
Now you can use the YandexGPT API.
The names of methods YandexGptApi
are same to the names of API methods.
Available API calls:
When generating large text with configured small dio.options.receiveTimeout
a timeout error may occur.
final response = await api.generateText(
TextGenerationRequest(
model: GModel.yandexGpt('folder_id'),
messages: const [
Message.system("Some joke"),
Message.user("Generate joke"),
],
),
);
print(response.alternatives.first.message);
print(response.usage.totalTokens);
The generateAsyncText
returns the Operation object.
For handling Operation
you can use getOperationTextGenerate.
final response = await api.generateAsyncText(
TextGenerationRequest(
model: GModel.yandexGpt('folder_id'),
messages: const [
Message.system("Some joke"),
Message.user("Generate joke"),
],
),
);
print(response.done);
final asyncText = await api.generateAsyncText(/*request*/);
final response = await api.getOperationTextGenerate(asyncText.id);
print(response.done);
final response = await api.tokenizeCompletion(
TextGenerationRequest(
model: GModel.yandexGpt('folder_id'),
messages: const [
Message.system("Some joke"),
Message.user("Generate joke"),
],
),
);
print(response.tokens.length);
final response = await api.tokenizeText(
TokenizeTextRequest(
model: GModel.yandexGpt('folder_id'),
text: 'some_response_text',
),
);
print(response.tokens.length);
final response = await api.getTextEmbedding(
EmbeddingRequest(
model: VModel.documentation('folder_id'),
text: 'Some text',
),
);
print(response.embedding);
It is enough to catch an error of type ApiError
.
try {
await api.generateText(/*request*/);
} on ApiError catch (e) {
// Handle YandexGPT API errors
} on DioException catch (e) {
// Handle network errors
}
If you need information about the error:
try {
await api.generateText(/*request*/);
} on DetailedApiError catch (e) {
// Handle DetailedApiError
} on ShortApiError catch (e) {
// Handle ShortApiError
} on DioException catch (e) {
// Handle network errors
}
To cancel requests use Dio CancelToken
by passing API requests with cancelToken
param.
The handling cancellation is similar to the example from the Dio doc.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.