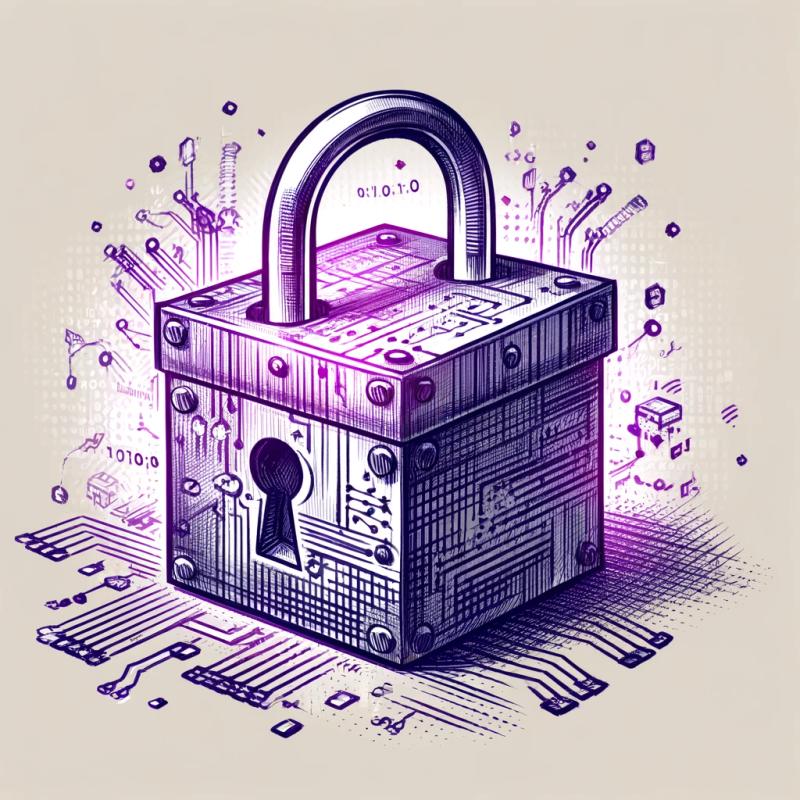
Security News
NIST Drafts New Security Framework to Tackle Emerging Risks of Generative AI
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.