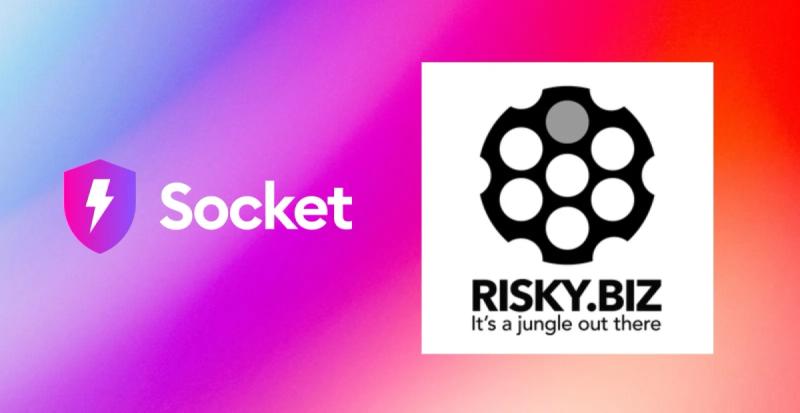
Security News
Risky Biz Podcast: How Shifts in Open Source Made It a Prime Attack Vector
This episode of the Risky Biz podcast discusses how the rise of small open source packages and the shift towards individual maintainers makes the ecosystem more vulnerable to supply chain attacks.