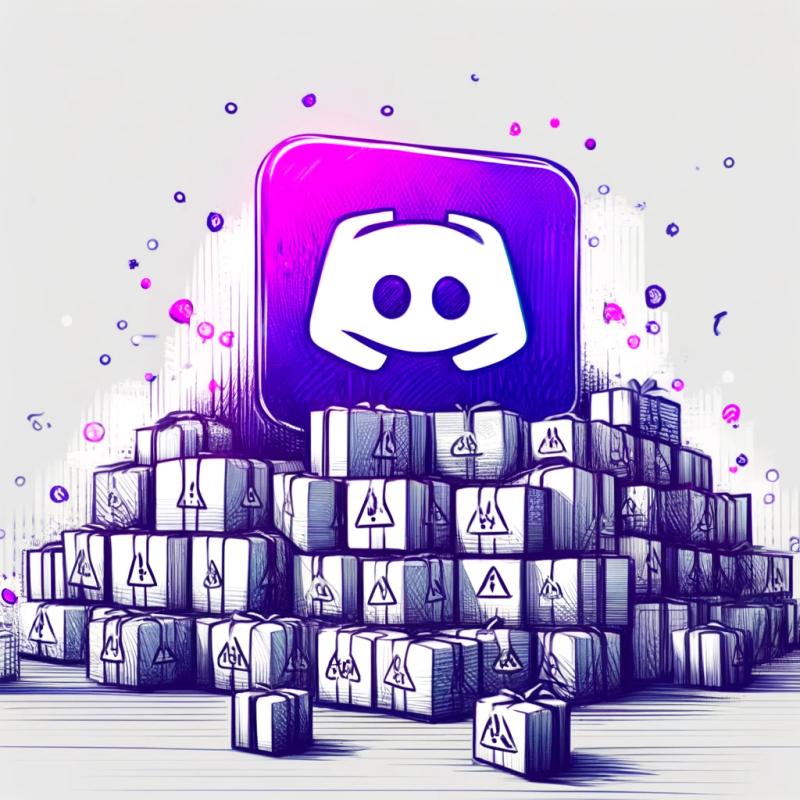
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
101-es6
Advanced tools
Readme
require('101/<util>')
.I ussually agree with this philosophy; however, while in practice, adherence to the module-pattern
can become quite annoying for micro-modules (like those in 101):
npm install 101
Just like ES6's Object.assign
. Extend an object with any number of objects (returns original).
import assign from '101/assign';
var target = { foo: 1 };
var source1 = { bar: 1 };
var source2 = { baz: 1 };
assign(target, source1) // { foo: 1, bar: 1, baz: 1 } target extended with source objects
assign(target, source1, source2) // { foo: 1, bar: 1, baz: 1 } target extended with source objects
Functional version of &&
. Works great with array.reduce
.
import and from '101/and';
and(true, false); // false
and(true, true); // true
Functional version of function.apply
.
Supports partial functionality (great with array functions).
import apply from '101/apply';
[sum].map(apply(null, [1, 2, 3])); // [6] = [sum(1,2,3)] = [1+2+3]
function sum () { /* sums all arguments */ }
apply({ prop: 'val' })(function () { return this.prop; }); // 'val'
It's clone (Only exporting this bc it is used internal to 101)
import clone from '101/clone';
var obj = {
foo: 1,
bar: 2
};
clone(obj); // { foo: 1, bar: 2 }
Functional composition method. Works great with array.reduce
.
import compose from '101/compose';
compose(isNaN, parseInt)('nope'); // isNaN(parseInt('nope')) // true
Functional version of str === process.env.NODE_ENV
.
Or's multiple environments.
import envIs from '101/env-is';
// process.env.NODE_ENV = development
envIs('development'); // true
envIs('production'); // false
envIs('staging', 'production'); // false
envIs('development', 'production'); // true
Functional version of ===
.
Supports partial functionality (great with array functions).
import equals from '101/equals';
equals(1, 1); // true
[1,2,3].some(equals(1)); // true
equals(1, '1'); // false
Simple exists function.
import exists from '101/exists';
exists('foo'); // true
exists(null); // false
exists(undefined); // false
Just like ES6's array.find
.
Finds the first value in the list that passes the given function (predicate) and returns it. If list is not provided find will return a partial-function which accepts a list as the first argument.
import find from '101/find';
import hasProps from '101/has-properties';
var arr = [{ a: 1, b: 1 }, { b: 1 }, { c: 1 }];
var item = find(arr, hasProps({ a:1 }));
// returns { a: 1, b: 1 }
// returns null if not found
Just like ES6's array.findIndex
.
Finds the first value in the list that passes the given function (predicate) and returns it's index. If list is not provided findIndex will return a partial-function which accepts a list as the first argument.
import findIndex from '101/find-index';
var arr = [1, 2, 3];
var index = findIndex(arr, function (val, i, arr) {
return val === 2;
});
// returns 1
// returns -1 if not found
Determines whether the keypaths exist and have the specified values. Supports partial functionality (great with array functions, and 101/find).
import hasKeypaths from '101/has-keypaths';
var obj = {
foo: {
bar: {
qux: 1
}
}
};
hasKeypaths(obj, ['foo.bar.qux']); // true
hasKeypaths(obj, { 'foo.bar.qux': 1 }); // true
hasKeypaths(obj, ['foo.qux']); // false
hasKeypaths(obj, { 'foo.bar': 2 }); // false
hasKeypaths(obj, { 'foo.bar': 1, 'nope': 1 }); // false
// optional 'deep' arg, defaults to true
var barObj = { bar: 1 };
hasKeypaths(obj, { 'foo.bar': barObj }); // true
hasKeypaths(obj, { 'foo.bar': barObj }, true); // true
hasKeypaths(obj, { 'foo.bar': barObj }, false); // false
hasKeypaths(obj, { 'foo.bar': obj.foo }, false); // true
hasKeypaths(obj, ['foo.bar'], false); // true, uses [hasOwnProperty vs in](http://stackoverflow.com/questions/13632999/if-key-in-object-or-ifobject-hasownpropertykey)
// use it with find, findIndex, or filter!
var arr = [obj, { b: 1 }, { c: 1 }];
find(arr, hasProps({ 'foo.bar.qux':1 })); // { foo: { bar: { qux: 1 } } }
find(arr, hasProps(['foo.bar.qux'])); // { foo: { bar: { qux: 1 } } }
Determines whether the keys exist and, if specified, has the values. Supports partial functionality (great with array functions, and 101/find).
import hasProps from '101/has-properties';
var obj = {
foo: {
bar: 1
},
qux: 1
};
hasProps(obj, ['foo', 'qux']); // true
hasProps(obj, { qux: 1 }) // true
// optional 'deep' arg, defaults to true
var barObj = { bar: 1 };
hasProps(obj, { 'foo.bar': barObj }); // true
hasProps(obj, { 'foo.bar': barObj }, true); // true
hasProps(obj, { 'foo.bar': barObj }, false); // false
hasProps(obj, ['foo.bar'], false); // true, uses [hasOwnProperty vs in](http://stackoverflow.com/questions/13632999/if-key-in-object-or-ifobject-hasownpropertykey)
// use it with find, findIndex, or filter!
var arr = [{ a: 1, b: 1 }, { b: 1 }, { c: 1 }];
find(arr, hasProps({ a:1 })); // { a: 1, b: 1 }
find(arr, hasProps(['a'])); // { a: 1, b: 1 }
Functional version of JavaScript's instanceof. Supports partial functionality (great with array functions).
import instanceOf from '101/instance-of';
['foo', 'bar', 1].map(instanceOf('string')); // [true, true, false]
Functional version of typeof val === 'boolean'
.
Supports partial functionality (great with array functions).
import isBoolean from '101/is-boolean';
[true, false, 1].map(isBoolean); // [true, true, false]
Functional version of val empty object, array or object
import isEmpty from '101/is-empty';
isEmpty([]); // true
isEmpty({}); // true
isEmpty(""); // true
isEmpty(" "); // false
Functional version of typeof val === 'function'
import isFunction from '101/is-function';
[parseInt, function () {}, 'foo'].map(isFunction); // [true, true, false]
Functional version of val typeof 'number'
import isNumber from '101/is-number';
['foo', 'bar', 1].map(isString); // [false, false, true]
Functional strict version of val typeof 'object' (and not array or regexp)
import isObject from '101/is-object';
[{}, { foo: 1 }, 100].map(isObject); // [true, true, false]
Functional version of val typeof 'string'
import isString from '101/is-string';
['foo', 'bar', 1].map(isString); // [true, true, false]
Returns the last value of a list
import last from '101/last';
last([1, 2, 3]); // 3
last('hello'); // 'o'
No-op function
require('101/noop'); // function () {}
Functional version of !
.
import not from '101/not';
not(isString)('hey'); // false
not(isString)(100); // true
Returns a new object without the specified keys. Supports partial functionality (great with array functions, like map).
import omit from '101/omit';
var obj = {
foo: 1,
bar: 2
};
omit(obj, 'foo'); // { bar: 1 }
omit(obj, ['foo']); // { bar: 1 }
omit(obj, ['foo', 'bar']); // { }
// use it with array.map
[obj, obj, obj].map(omit('foo')); // [{ bar: 1 }, { bar: 1 }, { bar: 1 }];
Functional version of ||
.
Works great with array.reduce
.
import or from '101/or';
or(true, true); // true
or(true, false); // true
or(false, false); // false
Muxes arguments across many functions and &&
's the results.
Supports partial functionality (great with array functions, like map).
import passAll from '101/pass-all';
['', 'foo', 'bar', 100].map(passAll(isString, isTruthy)); // [false, true, true, false]
Muxes arguments across many functions and ||
's the results.
Supports partial functionality (great with array functions, like map).
import passAny from '101/pass-any';
['', 'foo', 'bar', 100].map(passAny(isString, isNumber)); // [true, true, true, true]
Returns a new object with the specified keys (with key values from obj). Supports partial functionality (great with array functions, like map).
import pick from '101/pick';
var obj = {
foo: 1,
bar: 2
};
pick(obj, 'foo'); // { foo: 1 }
pick(obj, ['foo']); // { foo: 1 }
pick(obj, ['foo', 'bar']); // { foo: 1, bar: 2 }
// use it with array.map
[obj, obj, obj].map(pick('foo')); // [{ foo: 1 }, { foo: 1 }, { foo: 1 }];
Functional version of obj[key], returns the value of the key from obj. Supports partial functionality (great with array functions, like map).
import pluck from '101/pluck';
var obj = {
foo: 1,
bar: 2
};
pluck(obj, 'foo'); // 1
// use it with array.map
[obj, obj, obj].map(pluck('foo')); // [1, 1, 1]
// supports keypaths by default
var obj = {
foo: {
bar: 1
},
'foo.bar': 2
};
pluck(obj, 'foo.bar'); // 1, supports keypaths by default
pluck(obj, 'foo.bar', false); // 2, pass false to not use keypaths
Functional version of obj[key] = val, returns a new obj with the key and value set. Supports partial functionality (great with array functions, like map).
import set from '101/set';
var obj = {
foo: 1,
bar: 2
};
set(obj, 'foo'); // 1
// use it with array.map
[obj, obj, obj].map(set('foo', 100)); // [{ foo: 100, bar: 2 }, {same}, {same}]
// supports keypaths by default
var obj = {
foo: 1,
bar: 2
};
set(obj, 'foo', 100); // { foo: 100, bar:2 }
MIT
FAQs
common javascript utils that can be required selectively that assume es5+
We found that 101-es6 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.