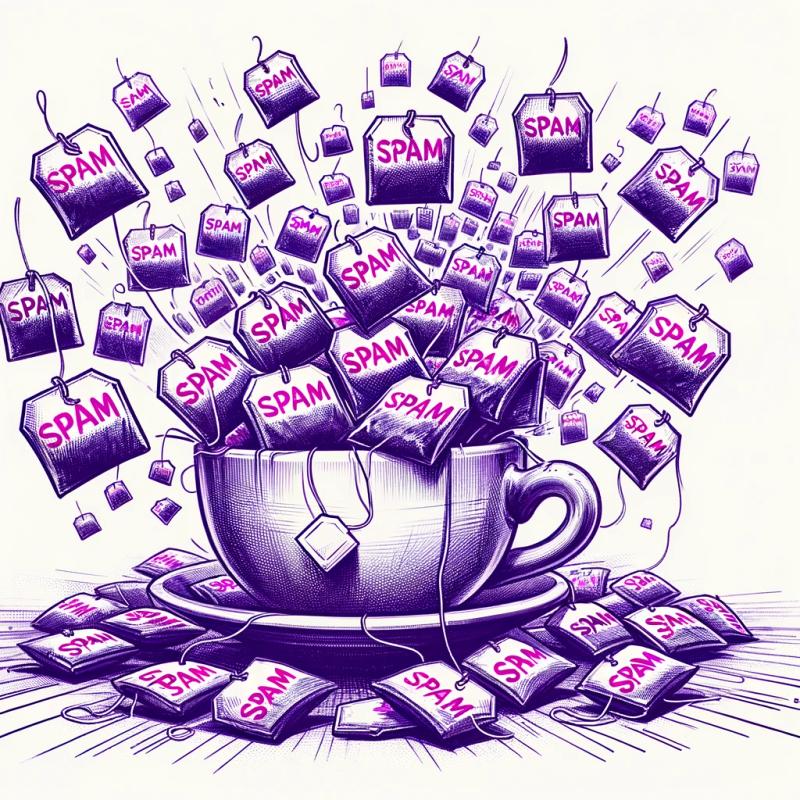
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@agape/api
Advanced tools
Readme
Framework for building APIs
@Service()
class FooService {
foo() {
return { "message": "BARRRRGGHHHHH" }
}
}
@Controller()
class FooController {
constructor( public service: FooService ) {
}
@Get('foo')
foo() {
return this.service.foo()
}
}
@Module({
controllers: [FooController]
})
class FooModule() { }
const app: Application = express();
app.use( express.json() )
app.use( express.urlencoded({ extended: true }))
const router = Router()
bootstrapExpress(router, FooModule)
app.use('/api', router )
Model-View-Controller APIs
There are three primary components to an API application. Modules, Controllers, and Services.
Controllers respond to routed requests by interacting with services
and returning a response to be sent back to the end user. The Controller
class decorator is used to designate a class as a controller.
Controller
@Controller('path/to/controller')
class FooController {
...
}
One method decorator for each HTTP request.
@Get(path)
@Post(path)
@Put(path)
@Patch(path)
@Delete(path)
@Status(code)
Set a custom success status code. Default is 200 OK
.
@Get('teapot')
@Status(418)
teapot() {
...
}
@Respond(model)
@Controller('foos')
class FoosController {
@Get()
list() {
}
@Post()
create( params: undefined, body: IEvent ) {
...
}
@Get(':id')
retrieve( params: { id: string } ) {
...
}
@Put(':id')
update( params: { id: string }, body: IEvent ) {
...
}
@Patch(':id')
update( params: { id: string }, body: Partial<IEvent> ) {
...
}
@Delete(':id')
retrieve( params: { id: string } ) {
...
}
}
Services are injected into controllers and other services through the contstructor.
Declare a service using the Service
decorator. Only one instance of a service is
used across the entire application via dependency injection.
Service
@Service()
class FooService {
foo() {
...
}
}
@Service()
class BarService {
constructor( public foo: FooService ) {
}
}
Modules contain one or more controllers. Controllers need to
be declared in a module to be accessible through the API. Declaring
a service as part of a module through the provides
parameter is
optional.
Module
controllers
modules
path
@Module({
'controllers': [ FooController ],
'modules': [ BarModule ]
})
You must have the compiler options experimentalDecorators
and
emitDecoratorMetadata
set to true
in your tsconfig.json
file.
This means that you cannot bundle with esbuild
which does not
support these compiler options.
Maverik Minett maverik.minett@gmail.com
© 2023 Maverik Minett
MIT
FAQs
Framework for building Rest APIs
The npm package @agape/api receives a total of 2 weekly downloads. As such, @agape/api popularity was classified as not popular.
We found that @agape/api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.