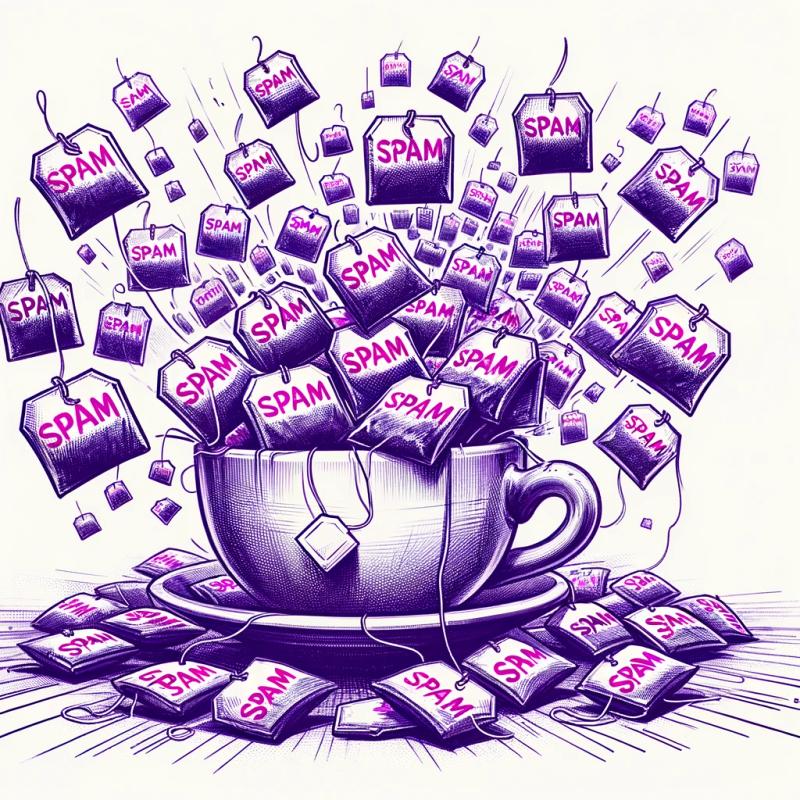
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@aitmed/aitmed-ecos-sdk
Advanced tools
Readme
const sdk = new SDK({ apiVersion, env: 'development' })
const doSomething = async () => {
const phone_number = '+1 1234567890'
const password = '1234567890'
// Request Verification Code
const verification_code = await sdk.account.requestVerificationCode(phone_number)
// Create User
await sdk.account.create(
phone_number,
password,
parseInt(verification_code),
)
// Login
await sdk.account.login(
phone_number,
password,
verification_code,
)
}
type ApiVersion = 'v1beta1' | 'v1beta2'
type ENV = 'development' | 'production'
interface ResponseCatcher {
(response: Response): Response
}
interface ErrorCatcher {
(error: Level2Error): void
}
class Store {
constructor({
apiVersion: ApiVersion
env: ENV
})
public readonly level2SDK
public env: ENV
public apiVersion: ApiVersion
public responseCatcher: ResponseCatcher
public errorCatcher: ErrorCatcher
public setResponseCatcher(catcher?: ResponseCatcher)
public setErrorCatcher(catcher?: ErrorCatcher)
}
class SDK {
constructor({
apiVersion: ApiVersion
env: ENV
})
public readonly account: Account
public readonly notebook: Notebook
public readonly note: Note
public apiVersion: ApiVersion
public setResponseCatcher(catcher?: ResponseCatcher)
public setErrorCatcher(catcher?: ErrorCatcher)
}
AiTmedError {
readonly code: number
readonly name: string
readonly message: string
constructor({
code?: number,
name?: string,
message?: string
})
}
AiTmedError | Name | Default Message |
---|---|---|
-1 | UNKNOW_ERROR | error occurred |
1 | PERMISSION_DENIED | permission denied |
2 | UNREGISTERED | account is not registered |
3 | REGISTERED | account is already registered |
/_ Accoutn _/ | ||
1000 | PHONE_NUMBER_INVALID | phone number is invalid |
1001 | PASSWORD_INVALID | password is invalid |
1002 | VERIFICATION_CODE_INVALID | verification code is invalid |
1003 | REQUIRED_VERIFICATION_CODE | verification code is required |
1004 | REQUIRED_PASSWORD | password is required |
1005 | USER_NOT_FOUND | user is not found |
/_ Notebook _/ | ||
2000 | NOTEBOOK_NOT_EXIST | notebook is not exist |
2001 | NOTEBOOK_PERMISSION_DENIED | notebook permission denied |
/_ Note _/ | ||
3000 | NOTE_NOT_EXIST | note is not exist |
3001 | NOTE_PERMISSION_DENIED | note permission denied |
3002 | NOTE_CONTENT_INVALID | note content is invalid |
interface User {
id: Uint8Array | string
phone_number: string
roles: number
first_name?: string
middle_name?: string
last_name?: string
profile_photo?: string
gender?: 'MALE' | 'FEMALE' | 'PNS'
birthday?: number
languages?: string[]
}
account.requestVerificationCode
requestVerificationCode(
phone_number: string
): Promise<string>
account.create
create(
phone_number: string,
password: string,
verification_code: number
): Promise<void>
account.login
login(
phone_number: string,
password: string,
verification_code: number
): Promise<void>
account.loginByPassword
login(
password: string
): Promise<void>
This method is only able to be used after login new device (
loginByVerificationCode
)
account.loginByVerificationCode
login(
phone_number: string,
verification_code: number
): Promise<void>
account.logout
logout(): Status
account.update
interface UpdateParams {
first_name?: string
middle_name?: string
last_name?: string
profile_photo?: string
gender?: 'MALE' | 'FEMALE' | 'PNS'
birthday?: number
languages?: string[]
}
update(
id: Uint8Array | string,
params: UpdateParams
): Promise<User>
account.updatePhoneNumber
NOT IMPLEMENTupdatePhoneNumber(params: {
phone_number: string,
new_phone_number: string,
verification_code: string,
}): Promise<User>
account.updatePassword
NOT IMPLEMENTinterface UdpatePasswordParams {
phone_number: string
new_password: string
verification_code?: string
password?: string
}
updatePassword(params: UdpatePasswordParams): Promise<void>
account.retrieve
updatePassword(id: Uint8Array | string): Promise<User>
interface Notebook {
id: Uint8Array | string
owner_id: Uint8Array | string
info: {
title: string
edit_mode: number
}
}
account.getStatus
getStatus(): Status
notebook.create
create(title: string): Promise<NotebookType>
notebook.remove
remove(id: string | Uint8Array): Promise<NotebookType>
notebook.update
update(id: string | Uint8Array, fields: {
title?: string
}): Promise<NotebookType>
notebook.retrieve
retrieve(id: string | Uint8Array): Promise<NotebookType>
notebook.list
list(params: {
shared?: Boolean
count?: number
edit_mode?: number
sort_by?: 0 | 1 | 2
}): Promise<{
ids: Set<string | Uint8Array>
mapper: Map<string | Uint8Array, NotebookType>
}>
notebook.share
NOT IMPLEMENTshare(
id: string | Uint8Array,
invite_phone_number: string,
edit_mode: number
): Promise<void>
notebook.unshare
NOT IMPLEMENTunshare(
id: string | Uint8Array,
invited_phone_number: string
): Promise<void>
interface NoteBase {
id: string
owner_id: string
notebook_id: string
edit_mode: number
title: string
tags: string[]
created_at: number
modified_at: number
modified_by: string
}
type NoteTypes =
| 0 // text
| 1 // form
interface Note extends NoteBase {
type: NoteTypes
content: string
}
type NoteFileTypes =
| 2 // image
| 3 // pdf
| 4 // vedio
interface NoteFile extends NoteBase {
// title will be the file name
type: NoteFileTypes
file: Blob
file_size: number
}
note.create
create(params: {
notebook_id: string
title: string
content: string | Blob
type: NoteTypes | NoteFileTypes
}): {
code: Code
data: {
note: Note | NoteFile | null
}
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
NOTEBOOK_NOT_EXIST | |
NOTEBOOK_PERMISSION_DENIED |
note.remove
remove(id: string): {
code: Code
data: {
note: Note | NoteFile | null
}
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
NOTEBOOK_NOT_EXIST | |
NOTEBOOK_PERMISSION_DENIED |
note.update
update(id: string, fields: {
notebook_id?: string
title?: string
content?: string | Blob
}): {
code: Code
data: {
note: Note | NoteFile | null
}
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
NOTEBOOK_NOT_EXIST | |
NOTEBOOK_PERMISSION_DENIED | |
NOTE_NOT_EXIST | |
NOTE_PERMISSION_DENIED | |
NOTE_CONTENT_INVALID |
note.retrieve
retrieve(id: string): {
code: Code
data: {
note: Note | NoteFile | null
}
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
NOTE_NOT_EXIST | |
NOTE_PERMISSION_DENIED |
note.list
list(notebook_id: string, options: {
shared?: Boolean
types?: (NoteTypes | NoteFileTypes)[]
tags?: string[]
count?: number
edit_mode?: number
sort_by?: 0 | 1 | 2
}): {
code: Code
data: {
ids: string[]
mapper: {
[id: string]: Note | NoteFile
}
}
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
NOTE_NOT_EXIST | |
NOTE_PERMISSION_DENIED |
note.share
share(id: string, invite_phone_number: string, edit_mode: number): {
code: Code
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
PHONE_NUMBER_INVALID | |
NOTE_NOT_EXIST | |
NOTE_PERMISSION_DENIED |
note.unshare
unshare(id: string, invited_phone_number: string): {
code: Code
}
Return Code | |
---|---|
SUCCESS | |
PERMISSION_DENIED | |
PHONE_NUMBER_INVALID | |
NOTE_NOT_EXIST | |
NOTE_PERMISSION_DENIED |
FAQs
> Application layer sdk for the AiTmed platform.
The npm package @aitmed/aitmed-ecos-sdk receives a total of 3 weekly downloads. As such, @aitmed/aitmed-ecos-sdk popularity was classified as not popular.
We found that @aitmed/aitmed-ecos-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.