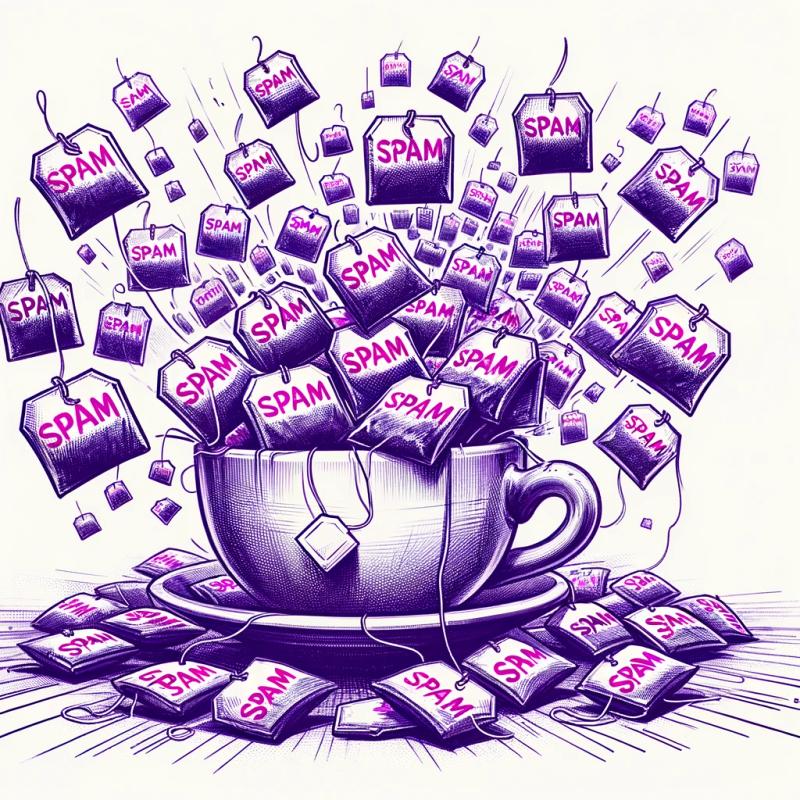
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@alpinesoft/express-plus
Advanced tools
Readme
This lightweight Express.js middleware focuses on two main features:
const expressPlus = require('express-plus')
app.use(expressPlus.createMiddleware())
const { Rule } = require('@cesium133/forgjs')
const bodyRules = {
username: new Rule({
type: 'string',
minLength: 3,
maxLength: 120,
notEmpty: true
}),
password: new Rule({
type: 'password',
minLength: 3,
maxLength: 120,
notEmpty: true
})
}
app.post('/', (req, res) => {
const isValid = req.checkBody(bodyRules)
// Error will be sent automatically
if (!isValid) {
return
}
// Continue handling the request if body is valid
})
This will result in:
{
"status": "ERROR",
"error": {
"name": "Invalid request body",
"message": "Could not parse request body, check for invalid or missing fields"
}
}
app.post('/', (req, res) => {
res.resolve({
yourData: 'FOR-EXAMPLE-A-TOKEN'
})
})
This will result in:
{
"status": "SUCCESS",
"payload": {
"yourData": "FOR-EXAMPLE-A-TOKEN"
}
}
res.reject(expressPlus.createHttpError(500, 'Your error', 'Provide a concise error message.'))
or
next(expressPlus.createHttpError(500, 'Your error', 'Provide a concise error message.'))
app.use(expressPlus.createErrorHandler())
Place this middleware usage call after every other to ensure full error handling. To learn more about Express.js error handling follow this link.
You can see a full example here.
FAQs
Lightweight Express.js middleware aimed to create standardised REST APIs
The npm package @alpinesoft/express-plus receives a total of 1 weekly downloads. As such, @alpinesoft/express-plus popularity was classified as not popular.
We found that @alpinesoft/express-plus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.