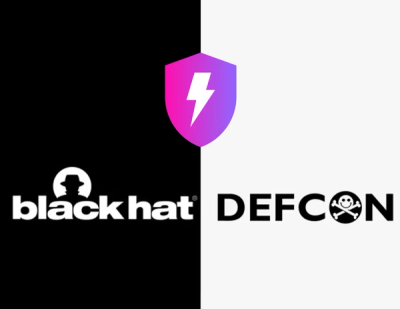
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
@angular-redux/core
Advanced tools
Angular bindings for Redux.
For Angular 1 see ng-redux
@angular-redux/core
lets you easily connect your Angular components with Redux, while still respecting the Angular idiom.
Features include:
Observables
@select
decoratorIn addition, we are committed to providing insight on clean strategies for integrating with Angular 2's change detection and other framework features.
Ng2Redux has a peer dependency on redux, so we need to install it as well.
npm install --save redux @angular-redux/core
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './containers/app.module';
platformBrowserDynamic().bootstrapModule(AppModule);
Import the NgReduxModule
class and add it to your application module as an
import
. Once you've done this, you'll be able to inject NgRedux
into your
Angular components. In your top-level app module, you
can configure your Redux store with reducers, initial state,
and optionally middlewares and enhancers as you would in Redux directly.
import { NgReduxModule, NgRedux } from '@angular-redux/core';
import reduxLogger from 'redux-logger';
import { rootReducer } from './reducers';
interface IAppState { /* ... */ };
@NgModule({
/* ... */
imports: [ /* ... */, NgReduxModule ]
})
export class AppModule {
constructor(ngRedux: NgRedux<IAppState>) {
ngRedux.configureStore(rootReducer, {}, [ createLogger() ]);
}
}
Or if you prefer to create the Redux store yourself you can do that and use the
provideStore()
function instead:
import {
applyMiddleware,
Store,
combineReducers,
compose,
createStore
} from 'redux';
import { NgReduxModule, NgRedux } from '@angular-redux/core';
import reduxLogger from 'redux-logger';
import { rootReducer } from './reducers';
interface IAppState { /* ... */ };
export const store: Store<IAppState> = createStore(
rootReducer,
compose(applyMiddleware(reduxLogger)));
@NgModule({
/* ... */
imports: [ /* ... */, NgReduxModule ]
})
class AppModule {
constructor(ngRedux: NgRedux<IAppState>) {
ngRedux.provideStore(store);
}
}
Now your Angular app has been reduxified! Use the @select
decorator to
access your store state, and .dispatch()
to dispatch actions:
import { select } from '@angular-redux/core';
@Component({
template: '<button (click)="onClick()">Clicked {{ count | async }} times</button>'
})
class App {
@select() count$: Observable<number>;
constructor(private ngRedux: NgRedux<IAppState>) {}
onClick() {
this.ngRedux.dispatch({ type: INCREMENT });
}
}
Here are some examples of Ng2Redux in action:
@angular-redux/core uses an approach to redux based on RxJS Observables to select
and transform
data on its way out of the store and into your UI or side-effect handlers. Observables
are an efficient analogue to reselect
for the RxJS-heavy Angular world.
Read more here: Select Pattern
We also have a number of 'cookbooks' for specific Angular topics:
FAQs
Angular 2 bindings for Redux
We found that @angular-redux/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.