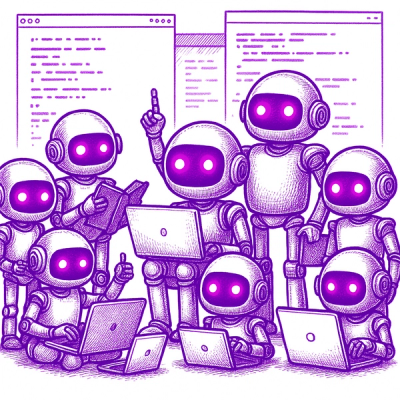
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
@angular/compiler
Advanced tools
The @angular/compiler package is a core part of the Angular framework that is responsible for compiling Angular templates into JavaScript code that can be executed by the browser. It takes the templates and annotations provided in Angular components and generates code that represents the structure and behavior of the application.
Template Compilation
This feature allows you to compile Angular components and modules dynamically at runtime. The code sample demonstrates how to use the JIT compiler to compile a module and its components asynchronously.
import { Compiler, COMPILER_OPTIONS, CompilerFactory } from '@angular/core';
import { JitCompilerFactory } from '@angular/platform-browser-dynamic';
// Get a reference to the JIT compiler factory
const compilerFactory: CompilerFactory = platformBrowserDynamic().injector.get(CompilerFactory);
const compiler = compilerFactory.createCompiler();
// Compile a component dynamically
compiler.compileModuleAndAllComponentsAsync(DynamicModule)
.then(compiled => {
const moduleRef = compiled.ngModuleFactory.create(this.injector);
const componentFactory = compiled.componentFactories[0];
this.container.createComponent(componentFactory);
});
AOT Compilation
Ahead-of-Time (AOT) compilation converts Angular HTML and TypeScript code into efficient JavaScript code during the build process, before the browser downloads and runs the code. This code sample shows how to bootstrap an Angular application using the AOT-compiled module factory.
import { enableProdMode } from '@angular/core';
import { platformBrowser } from '@angular/platform-browser';
import { AppModuleNgFactory } from './app/app.module.ngfactory';
enableProdMode();
platformBrowser().bootstrapModuleFactory(AppModuleNgFactory);
Babel is a JavaScript compiler that converts ECMAScript 2015+ code into a backwards-compatible version of JavaScript that can be run by older JavaScript engines. Babel is similar to @angular/compiler in that it performs code transformation, but it is not specific to Angular and does not compile Angular templates.
TypeScript is a superset of JavaScript that compiles to plain JavaScript. It is similar to @angular/compiler in that it performs a compilation step, but TypeScript focuses on type-checking and converting TypeScript code to JavaScript, rather than compiling framework-specific templates.
The vue-template-compiler package is used to compile Vue.js templates into render functions. It is similar to @angular/compiler as both are used for compiling framework-specific templates, but they target different frameworks (Vue.js vs Angular).
The sources for this package are in the main Angular repo. Please file issues and pull requests against that repo.
Usage information and reference details can be found in Angular documentation.
License: MIT
13.3.12 (2022-11-21)
| Commit | Type | Description | | -- | -- | -- | | b1d7b79ff4 | fix | hardening attribute and property binding rules for <iframe> elements (#48029) |
Andrew Kushnir, Andrew Scott, George Looshch, Joey Perrott and Paul Gschwendtner
<!-- CHANGELOG SPLIT MARKER --><a name="14.2.12"></a>
FAQs
Angular - the compiler library
The npm package @angular/compiler receives a total of 3,241,676 weekly downloads. As such, @angular/compiler popularity was classified as popular.
We found that @angular/compiler demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.