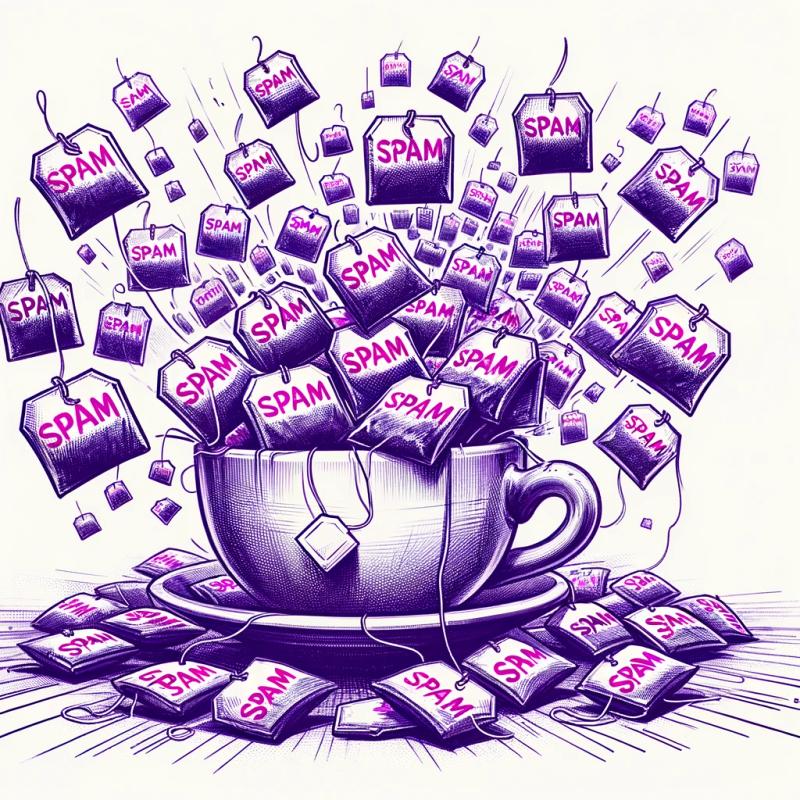
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@antv/coord
Advanced tools
Readme
$ npm install @antv/coord
import { Coordinate, Options } from '@antv/coord';
const optons: Options = {
x: 0,
y: 0,
width: 500,
height: 500,
transformations: [['cartesian']]
};
const coord = new Coordinate(options);
coord.transform('translate', 10, 10);
coord.map([0.5, 0.5]); // [260, 260]
coord.getSize(); // [500, 500]
coord.getCenter(); // [250, 250]
$ git clone git@github.com:antvis/coord.git
$ cd coord
$ npm i
$ npm t
Then send a pull request after coding.
MIT@AntV.
FAQs
Toolkit for mapping elements of sets into geometric objects.
The npm package @antv/coord receives a total of 117,583 weekly downloads. As such, @antv/coord popularity was classified as popular.
We found that @antv/coord demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 67 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.