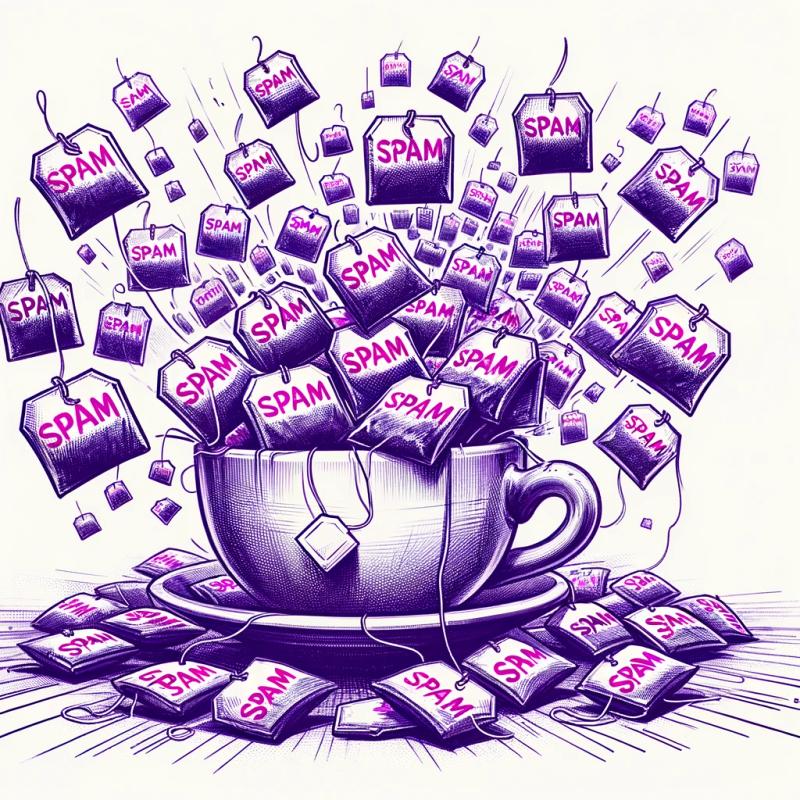
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@antv/react-g
Advanced tools
Readme
react render for @antv/g
npm i @antv/react-g
react-g provide host-component:
Canvas
and Group
.Text
, Circle
, Ellipse
, Image
, Line
, Marker
, Path
, Polygon
and Polyline
.import React, { useState } from 'react';
import { Canvas, Circle } from '@antv/react-g';
import { Renderer as CanvasRenderer } from '@antv/g-canvas';
const renderer = new CanvasRenderer();
const App = () => {
const [size, setSize] = useState(50);
return (
<Canvas width={600} height={400} renderer={renderer}>
<Circle
cx={100}
cy={200}
r={size}
fill="#1890FF"
stroke="#F04864"
lineWidth={4}
onClick={() => {
setSize(100);
}}
/>
</Canvas>
);
};
export default App;
Like react-dom, you can use ref
to access the shape instance.
import React, { useState, useRef } from 'react';
import { Canvas, Circle } from '@antv/react-g';
import { Renderer as CanvasRenderer } from '@antv/g-canvas';
const renderer = new CanvasRenderer();
const App = () => {
const circleRef = useRef();
const [size, setSize] = useState(50);
return (
<Canvas width={600} height={400} renderer={renderer}>
<Circle
ref={circleRef}
cx={100}
cy={200}
r={size}
fill="#1890FF"
stroke="#F04864"
lineWidth={4}
onClick={() => {
setSize(100);
}}
/>
</Canvas>
);
};
export default App;
render
react-g component to target g elementimport React, { useState } from 'react';
import { Canvas as GCanvas } from '@antv/g';
import { Circle, render } from '@antv/react-g';
import { Renderer as CanvasRenderer } from '@antv/g-canvas';
const renderer = new CanvasRenderer();
const CircleComponent = () => {
const [size, setSize] = useState(50);
return (
<Circle
cx={100}
cy={200}
r={size}
fill="#1890FF"
stroke="#F04864"
lineWidth={4}
onMouseenter={() => {
setSize(100);
}}
onMouseleave={() => {
setSize(50);
}}
/>
);
};
const canvas = new GCanvas({
container: 'container', // DOM 节点id
width: 600,
height: 500,
renderer,
});
// canvas can also be group/shape
render(<CircleComponent />, canvas);
FAQs
react render for @antv/g
The npm package @antv/react-g receives a total of 191 weekly downloads. As such, @antv/react-g popularity was classified as not popular.
We found that @antv/react-g demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 64 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.