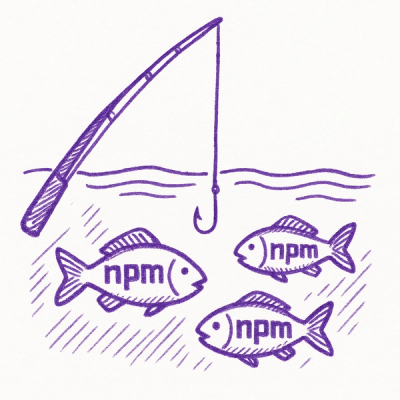
Security News
/Research
npm Phishing Email Targets Developers with Typosquatted Domain
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
@arterial/textfield
Advanced tools
Another React Material Text Field
npm install @arterial/textfield
@use "@material/floating-label/index.scss" as floating-label;
@use "@material/line-ripple/index.scss" as line-ripple;
@use "@material/notched-outline/index.scss" as notched-outline;
@use "@material/textfield/helper-text/index.scss" as textfield-helper-text;
@use "@material/textfield/character-count/index.scss" as textfield-character-count;
@use "@material/textfield/icon/index.scss" as textfield-icon;
@use "@material/textfield/index.scss" as textfield;
@include floating-label.core-styles;
@include line-ripple.core-styles;
@include notched-outline.core-styles;
@include textfield-helper-text.helper-text-core-styles;
@include textfield-character-count.character-count-core-styles;
@include textfield-icon.icon-core-styles;
@include textfield.core-styles;
import '@material/textfield/dist/mdc.textfield.css';
import {TextField, HelperText} from '@arterial/textfield';
Filled text fields have more visual emphasis than outlined text fields, making them stand out when surrounded by other content and components.
function Filled() {
const [value, setValue] = useState('');
return (
<Textfield
id="filled"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
Outlined text fields have less visual emphasis than filled text fields. When they appear in places like forms, where many text fields are placed together, their reduced emphasis helps simplify the layout.
function Outlined() {
const [value, setValue] = useState('');
return (
<Textfield
id="outlined"
label="Outlined"
onChange={e => setValue(e.target.value)}
outlined
value={value}
/>
);
}
function HelperTextObject() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={{
persistent: true,
validationMsg: true,
text: 'Helper text as object.',
}}
id="filled-helper-text-object"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function HelperTextComponent() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-helper-text-component"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function LeadingIcon() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
icon="calendar_today"
id="filled-leading-icon"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function TrailingIcon() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-trailing-icon"
label="Filled"
onChange={e => setValue(e.target.value)}
trailingIcon="search"
value={value}
/>
);
}
function TrailingIconAction() {
const [value, setValue] = useState('');
return (
<TextField
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-trailing-icon"
label="Filled"
onChange={e => setValue(e.target.value)}
onTrailingIconAction={() => setValue('')}
trailingIcon="delete"
value={value}
/>
);
}
function Invalid() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-invalid"
invalid
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function LabelFloating() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-label-floating"
label="Filled"
labelFloating
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function EndAligned() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
endAligned
id="filled-end-aligned"
onChange={e => setValue(e.target.value)}
placeholder="Filled"
value={value}
/>
);
}
function NoLabel() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-no-label"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function Disabled() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
disabled
id="filled-disabled"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function Placeholder() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-placeholder"
label="Filled"
onChange={e => setValue(e.target.value)}
placeholder="Placeholder"
value={value}
/>
);
}
function Required() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-required"
label="Filled"
onChange={e => setValue(e.target.value)}
required
value={value}
/>
);
}
function Loader() {
const [value, setValue] = useState('');
const [loading, setLoading] = useState(false);
return (
<>
<Button
label="Start Loader"
onClick={() => {
setLoading(true);
setTimeout(() => {
setLoading(false);
}, 5000);
}}
style={{marginBottom: '8px'}}
/>
<Textfield
disabled={loading}
helperText={
<HelperText
persistent
validationMsg
text="Helper text as component."
/>
}
id="filled-loader"
label="Filled"
labelFloating
onChange={e => setValue(e.target.value)}
placeholder={loading ? 'Loading...' : null}
trailingIcon={loading ? <CircularProgress small /> : null}
value={value}
/>
</>
);
}
function PreFilled() {
const [value, setValue] = useState('pre-filled');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-pre-filled"
label="Filled"
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function Prefix() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-prefix"
label="Filled"
labelFloating
onChange={e => setValue(e.target.value)}
prefix="$"
value={value}
/>
);
}
function Suffix() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-suffix"
label="Filled"
labelFloating
onChange={e => setValue(e.target.value)}
suffix=".00"
value={value}
/>
);
}
function CharacterCount() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="filled-suffix"
label="Filled"
maxLength={5}
onChange={e => setValue(e.target.value)}
value={value}
/>
);
}
function Textarea() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="textarea"
onChange={e => setValue(e.target.value)}
textarea
value={value}
/>
);
}
function TextareaCharacterCount() {
const [value, setValue] = useState('');
return (
<Textfield
helperText={
<HelperText persistent validationMsg text="Helper text as component." />
}
id="textarea"
maxLength={5}
onChange={e => setValue(e.target.value)}
textarea
value={value}
/>
);
}
Name | Type | Description |
---|---|---|
children | node | Elements to be displayed within root element. |
className | string | Classes to be applied to the root element. |
disabled | boolean | Indicates whether the element is disabled. |
helperText | node | Gives context about a select, such as how the selection will be used. |
icon | string | node | Icon to render within root element. |
id | string | Id of the element. |
invalid | boolean | Indicates the select is invalid. |
fullwidth | string | Sets the text field to full width. |
label | string | Text to be displayed within the root element. |
labelFloating | boolean | Indicates whether the elements label is floating. |
maxLength | string | number | Maximum length in characters of the value. Enables character counter. |
noLabel | boolean | Enables no label variant. |
onChange | function | Change event handler. |
onFocus | function | Focus event handler. |
onIconAction | function | Icon action event handler. |
onTrailingIconAction | function | Trailing icon action event handler. |
outlined | boolean | Enables an outlined variant. |
placeholder | string | Text to be displayed in the select when it has no value. |
prefix | string | Text to be displayed before value. |
style | object | Styles to be applied to the root element. |
suffix | string | Text to be displayed after value. |
textarea | boolean | Enables text area variant. |
trailingIcon | string | node | Icon to render on the right side of the root element. |
type | string | Determines type of text field. Defaults to text. |
value | string | Value of input. |
Name | Type | Description |
---|---|---|
className | string | Classes to be applied to the root element. |
id | string | Id of the element. |
persistent | boolean | Makes the helper text permanently visible. |
validationMsg | boolean | Indicates the helper text is a validation message. |
text | string | Text to be displayed. |
FAQs
Another React Material Text Field
The npm package @arterial/textfield receives a total of 1 weekly downloads. As such, @arterial/textfield popularity was classified as not popular.
We found that @arterial/textfield demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.