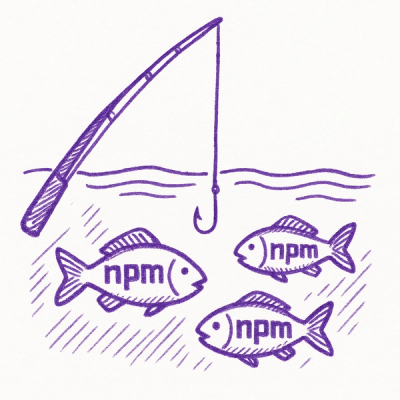
Security News
/Research
npm Phishing Email Targets Developers with Typosquatted Domain
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
@asphalt-react/textfield
Advanced tools
Textfield is an input field that enables users to type in data. It accepts data in various formats like text, numeric and password.Textfield is configurable to accept short or long form entries. You can configure its width and also attach SVG icons, words and React nodes to the input field.
The architecture of the Textfield family is flexible, so you can compose complex input fields as required.
import {
Textfield,
Email,
Search
} from "@asphalt-react/textfield"
<>
<Textfield/>
<Email/>
<Search/>
</>
Textfield suite offers following components specific to the nature of the input -
Qualifiers provide a hint about the expected content of the field. They can be SVG icons or words. You can attach the qualifier using qualifierStart
& qualifierEnd
. Qualifiers works great for use-cases like attaching currency ISO codes or symbols to the Textfield.
Along with qualifiers, Textfields also accept React nodes (a.k.a addon) to attach them beside qualifiers. You can use addons to compose components like for a phone number field with country code selector as an addon. You can add the React nodes on either ends of the field input simultaneously.
By default addons are rendered on the extreme ends of the field. Adjacent to the addons qualifiers are rendered and they are attached to the ends of the input field. The order of these entities from start to end is addOnStart
, qualifierStart
, input field in the middle, qualifierEnd
& addOnEnd
. You can swap the positions of Qualifiers and AddOns such that qualifiers appear on the extreme ends while addOns take the middle slots.
Apply multiline
prop to accept multiple rows of text. Only Textfield
supports multiline. Qualifiers
don't work in multiline fields.
You can compose complex input fields with the help of the following building blocks that are part of the Textfield family.
The Textfield family comes with:
Use the useInput()
hook to get all the prop getter functions and then spread them in the right children components. Pass all the props in the useInput()
hook as parameter.
import {
useInput,
InputWrapper,
InputQualifier,
InputAddOn,
Input
} from "@asphalt-react/textfield"
const { getInputProps, getWrapperProps, getQualifierProps } = useInput(props)
<InputWrapper {...getWrapperProps()}>
<InputQualifier {...getQualifierProps()}>
<IconPlaceHolder />
</InputQualifier>
<Input {...getInputProps()} />
<InputAddOn>
<Button icon>
<Add />
</Button>
</InputAddOn>
</InputWrapper>
data-/*
attributes & DOM attributesTo get access to the underlying DOM element, pass a React ref in the ref
prop.
inputmode="numeric"
.aria-invalid
attribute for components with invalid
prop set to true.React hook which returns prop getter functions. Use these functions to generate prop objects for different building blocks.
useInput accepts the following props:
Use this function to create props for InputWrapper component.
const { getWrapperProps } = useInput({ size: "m", invalid: false, disabled: false, stretch: true });
<InputWrapper {...getWrapperProps()}>
/* contents of InputWrapper */
</InputWrapper>
Use this function to create props for Input component.
const { getInputProps } = useInput({ size: "m", invalid: false, disabled: false, stretch: true });
<InputWrapper>
<Input
{...getInputProps()}
/>
/* rest of the contents */
</InputWrapper>
Use this function to create props for InputQualifier component.
const { getQualifierProps } = useInput({ size: "m" });
<InputWrapper>
<InputQualifier
{...getQualifierProps()}
/>
/* rest of the contents */
</InputWrapper>
Use this function to handle qualifiers and addOns attached to the start of the field. This function comes in handy when you create a new input field using the unit components like InputWrapper
and Input
as it handles the order of the elements attached. getStartSlots()
accepts two arguments:
const { getStartSlots } = useInput({
qualifierStart: <IconPlaceholder />,
addOnStart: <Button>action</Button>,
swapSlots: false,
});
<InputWrapper>
<>{getStartSlots({ qualifierProps: { ariaLabel: "qualifier" } })}</>
<Input />
</InputWrapper>
Use this function to handle qualifiers and addOns attached to the end of the field. This function comes in handy when you create a new input field using the unit components like InputWrapper
and Input
as it handles the order of the elements attached. getEndSlots()
accepts two arguments:
const { getEndSlots } = useInput({
qualifierStart: <IconPlaceholder />,
addOnStart: <Button>action</Button>,
swapSlots: true,
});
<InputWrapper>
<Input />
<>{getEndSlots({ qualifierProps: { ariaLabel: "qualifier" } })}</>
</InputWrapper>
The above code snipped will render the button (addOn) followed by the icon (qualifier) as swapSlots
is true.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Enables the field to accept multiple lines of text.
Qualifiers do not work with multiline.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Controls the height of a multiline
field. Accepts "low", "mid" & "high".
type | required | default |
---|---|---|
enum | false | "low" |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Make the field resizeable in horizontal direction.
This works only when multiline
is true.
type | required | default |
---|---|---|
bool | false | true |
Make the field resizeable in vertical direction.
This works only when multiline
is true.
type | required | default |
---|---|---|
bool | false | true |
Use Email field to accept email addresses
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Password field for securely accepting secrets. It renders a toggle button to show/hide the password. You can override the toggle button using addOnEnd
.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
This will override the default show/hide toggle button
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use URL field to accept or edit a URL
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Numeric field to accept numbers. The Numeric field renders an input element with type="text"
& inputmode="numeric"
to allow browsers to display an appropriate virtual keyboard. It also helps to eliminate some usability issues that comes with input with type="number"
.
For use cases of strictly speaking numbers like age, use Textfield component with type="number"
.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Search field to accept search keywords.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Datefield to work with dates.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Timefield to select a time.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon or text to render as start qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
Icon or text to render as end qualifier. Accepts SVG for icons.
type | required | default |
---|---|---|
union | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Use Pinfield for verification purposes, it can have numbers, alphabets, or characters as input but comes with the numeric
keyboard.
Pinfield allows 4 characters as input by default, but you can customize it using length
prop as per your use case.
Pinfield applies a placeholder value by default but you can override it using placeholder
prop.
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Icon to render as start qualifier, accepts SVG.
type | required | default |
---|---|---|
element | false | null |
Icon to render as end qualifier, accepts SVG.
type | required | default |
---|---|---|
element | false | null |
React node to render before the field content.
type | required | default |
---|---|---|
element | false | null |
React node to render after the field content.
type | required | default |
---|---|---|
element | false | null |
Swap the slots for qualifiers and addOns.
type | required | default |
---|---|---|
bool | false | N/A |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Number of characters allowed in the field.
type | required | default |
---|---|---|
number | false | 4 |
Aligns the input content in center.
type | required | default |
---|---|---|
bool | false | false |
Placeholder value to show in the field.
type | required | default |
---|---|---|
string | false | "" |
Use Phone Number field to accept phone number.
Controls size of the field. Accepts s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes the field disabled if true.
type | required | default |
---|---|---|
bool | false | false |
Field matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Show custom node when no result is found.
type | required | default |
---|---|---|
string | false | N/A |
Hint text to show in input field.
type | required | default |
---|---|---|
string | false | N/A |
Hint text to show in search input field.
type | required | default |
---|---|---|
string | false | "Search Country" |
Show country flag in option and button.
type | required | default |
---|---|---|
bool | false | false |
Array of country objects.
{
id: "+62",
name: "Indonesia",
flag: "https://www.url.co.id/indonesia-flag.svg"
initialSelected: false // true if this item should be initially selected
}
Out of the above id
and name
is required.
type | required | default |
---|---|---|
arrayOf | false | N/A |
Add default country. By add this props the selection will disabled.
type | required | default |
---|---|---|
string | false | "" |
Callback to handle the country selection and input field. It has the following signature
({countryCode, inputValue}, { event }) => {}
type | required | default |
---|---|---|
func | false | N/A |
Use InputWrapper to wrap various building blocks.
React node
type | required | default |
---|---|---|
node | false | null |
Controls size of the input. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Input matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Removes the spacing between its children.
type | required | default |
---|---|---|
bool | false | false |
Adds padding in the container.
type | required | default |
---|---|---|
bool | false | true |
Makes field non interactive.
type | required | default |
---|---|---|
bool | false | false |
Set this props to true if stretch is true and there is a qualifier/addOn at the end.
type | required | default |
---|---|---|
bool | false | false |
Input renders a native input field.
Type of input control.
type | required | default |
---|---|---|
string | false | "text" |
Controls size of the input. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
Input matches the width of its container.
type | required | default |
---|---|---|
bool | false | false |
Adds error styles to the field if true.
type | required | default |
---|---|---|
bool | false | false |
Makes field non interactive.
type | required | default |
---|---|---|
bool | false | false |
Add border on each sides
type | required | default |
---|---|---|
bool | false | true |
Adds padding on each sides
type | required | default |
---|---|---|
bool | false | true |
InputQualifier provides a hint about the expected content of the field. They can be SVG icons or words.
React node for the main content.
type | required | default |
---|---|---|
node | true | N/A |
Controls size of the field. Accepts xs, s, m, l for extra small, small, medium & large.
type | required | default |
---|---|---|
enum | false | "m" |
InputAddOn can be used to add extra React nodes along with Input.
React node for the main content.
type | required | default |
---|---|---|
node | true | N/A |
FAQs
Textfield
The npm package @asphalt-react/textfield receives a total of 609 weekly downloads. As such, @asphalt-react/textfield popularity was classified as not popular.
We found that @asphalt-react/textfield demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.