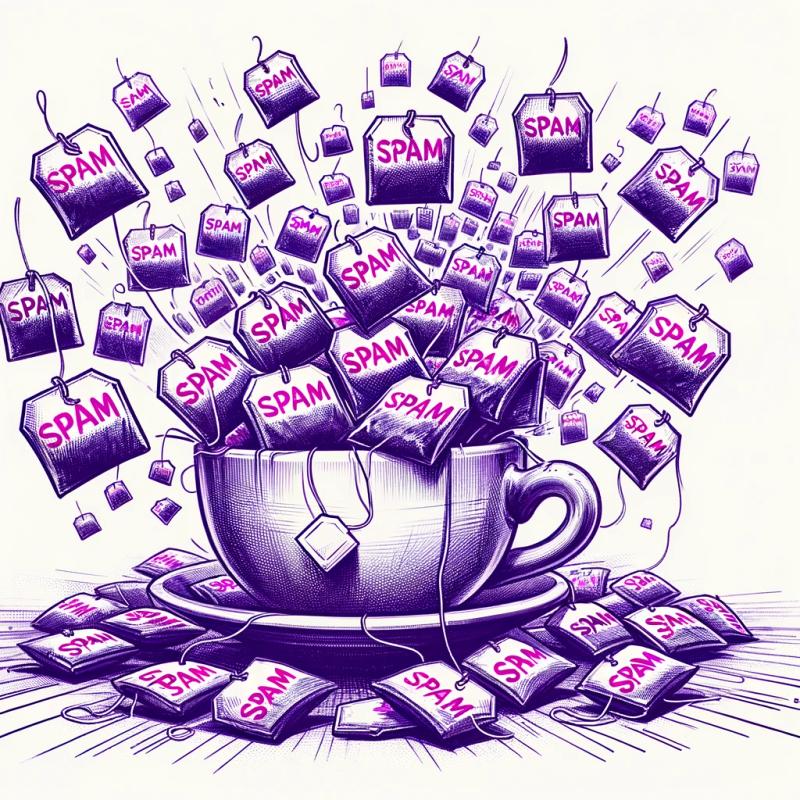
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@aws-sdk/client-cloudwatch-logs
Advanced tools
Package description
The @aws-sdk/client-cloudwatch-logs npm package allows developers to interact with the Amazon CloudWatch Logs service. This service provides monitoring, storing, and accessing log files from Amazon EC2 instances, AWS CloudTrail, and other sources. The package enables programmatic access to all the features of CloudWatch Logs, such as creating and managing log groups and streams, sending log events, and querying log data.
Creating a Log Group
This feature allows you to create a new log group in CloudWatch Logs. A log group is a collection of log streams that share the same retention, monitoring, and access control settings.
const { CloudWatchLogsClient, CreateLogGroupCommand } = require('@aws-sdk/client-cloudwatch-logs');
const client = new CloudWatchLogsClient({ region: 'us-west-2' });
const command = new CreateLogGroupCommand({ logGroupName: 'my-log-group' });
client.send(command);
Creating a Log Stream
This feature allows you to create a log stream within a log group. A log stream represents a sequence of log events that share the same source.
const { CloudWatchLogsClient, CreateLogStreamCommand } = require('@aws-sdk/client-cloudwatch-logs');
const client = new CloudWatchLogsClient({ region: 'us-west-2' });
const command = new CreateLogStreamCommand({ logGroupName: 'my-log-group', logStreamName: 'my-log-stream' });
client.send(command);
Putting Log Events
This feature allows you to send log data to a log stream in CloudWatch Logs. Each log event consists of a timestamp and a message.
const { CloudWatchLogsClient, PutLogEventsCommand } = require('@aws-sdk/client-cloudwatch-logs');
const client = new CloudWatchLogsClient({ region: 'us-west-2' });
const command = new PutLogEventsCommand({
logGroupName: 'my-log-group',
logStreamName: 'my-log-stream',
logEvents: [{ timestamp: Date.now(), message: 'This is a log message.' }]
});
client.send(command);
Searching and Filtering Log Data
This feature allows you to search and filter log data within a log group. You can specify a filter pattern, as well as a time range for the search.
const { CloudWatchLogsClient, FilterLogEventsCommand } = require('@aws-sdk/client-cloudwatch-logs');
const client = new CloudWatchLogsClient({ region: 'us-west-2' });
const command = new FilterLogEventsCommand({
logGroupName: 'my-log-group',
filterPattern: 'ERROR',
startTime: Date.now() - 3600000,
endTime: Date.now()
});
client.send(command);
winston-cloudwatch is a transport for the popular logging library winston, which allows you to send your logs to Amazon CloudWatch. Unlike @aws-sdk/client-cloudwatch-logs, which is the official AWS SDK for JavaScript, winston-cloudwatch integrates with the winston logging framework and provides a more streamlined way to log directly from your application.
pino-cloudwatch is a module that streams pino logs to Amazon CloudWatch. Pino is a very low overhead Node.js logger. This package is similar to @aws-sdk/client-cloudwatch-logs in that it allows logging to CloudWatch, but it is specifically designed to work with the pino logging library and focuses on performance.
Changelog
3.564.0 (2024-04-26)
Readme
AWS SDK for JavaScript CloudWatchLogs Client for Node.js, Browser and React Native.
You can use Amazon CloudWatch Logs to monitor, store, and access your log files from EC2 instances, CloudTrail, and other sources. You can then retrieve the associated log data from CloudWatch Logs using the CloudWatch console. Alternatively, you can use CloudWatch Logs commands in the Amazon Web Services CLI, CloudWatch Logs API, or CloudWatch Logs SDK.
You can use CloudWatch Logs to:
Monitor logs from EC2 instances in real time: You can use CloudWatch Logs to monitor applications and systems using log data. For example, CloudWatch Logs can track the number of errors that occur in your application logs. Then, it can send you a notification whenever the rate of errors exceeds a threshold that you specify. CloudWatch Logs uses your log data for monitoring so no code changes are required. For example, you can monitor application logs for specific literal terms (such as "NullReferenceException"). You can also count the number of occurrences of a literal term at a particular position in log data (such as "404" status codes in an Apache access log). When the term you are searching for is found, CloudWatch Logs reports the data to a CloudWatch metric that you specify.
Monitor CloudTrail logged events: You can create alarms in CloudWatch and receive notifications of particular API activity as captured by CloudTrail. You can use the notification to perform troubleshooting.
Archive log data: You can use CloudWatch Logs to store your log data in highly durable storage. You can change the log retention setting so that any log events earlier than this setting are automatically deleted. The CloudWatch Logs agent helps to quickly send both rotated and non-rotated log data off of a host and into the log service. You can then access the raw log data when you need it.
To install the this package, simply type add or install @aws-sdk/client-cloudwatch-logs using your favorite package manager:
npm install @aws-sdk/client-cloudwatch-logs
yarn add @aws-sdk/client-cloudwatch-logs
pnpm add @aws-sdk/client-cloudwatch-logs
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the CloudWatchLogsClient
and
the commands you need, for example ListAnomaliesCommand
:
// ES5 example
const { CloudWatchLogsClient, ListAnomaliesCommand } = require("@aws-sdk/client-cloudwatch-logs");
// ES6+ example
import { CloudWatchLogsClient, ListAnomaliesCommand } from "@aws-sdk/client-cloudwatch-logs";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new CloudWatchLogsClient({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListAnomaliesCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-cloudwatch-logs";
const client = new AWS.CloudWatchLogs({ region: "REGION" });
// async/await.
try {
const data = await client.listAnomalies(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listAnomalies(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listAnomalies(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-cloudwatch-logs
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
FAQs
AWS SDK for JavaScript Cloudwatch Logs Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-cloudwatch-logs receives a total of 1,424,778 weekly downloads. As such, @aws-sdk/client-cloudwatch-logs popularity was classified as popular.
We found that @aws-sdk/client-cloudwatch-logs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.