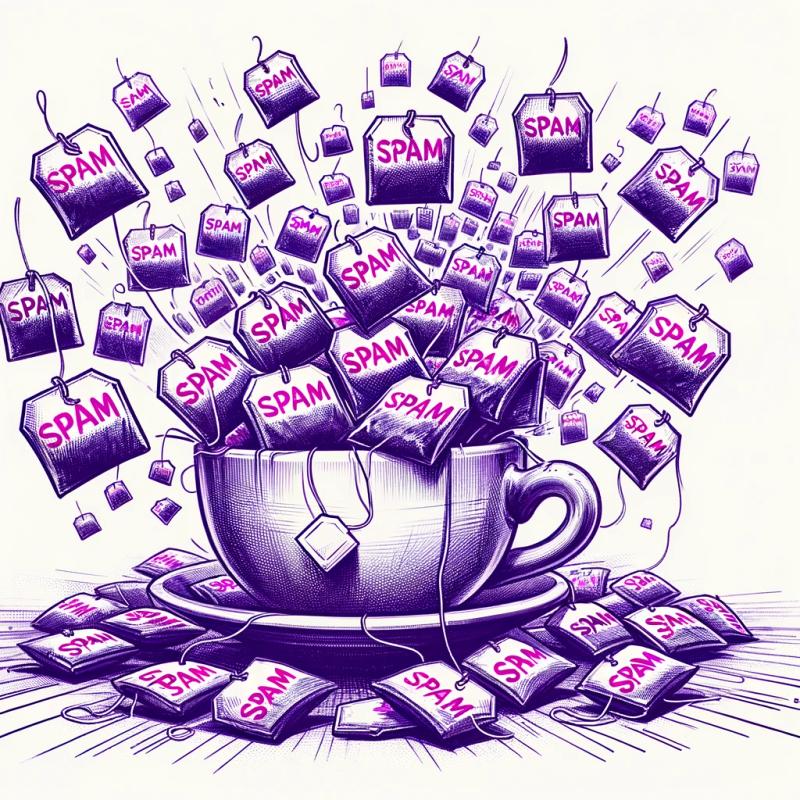
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@aws-sdk/client-securitylake
Advanced tools
Changelog
3.564.0 (2024-04-26)
Readme
AWS SDK for JavaScript SecurityLake Client for Node.js, Browser and React Native.
Amazon Security Lake is a fully managed security data lake service. You can use Security Lake to automatically centralize security data from cloud, on-premises, and custom sources into a data lake that's stored in your Amazon Web Services account. Amazon Web Services Organizations is an account management service that lets you consolidate multiple Amazon Web Services accounts into an organization that you create and centrally manage. With Organizations, you can create member accounts and invite existing accounts to join your organization. Security Lake helps you analyze security data for a more complete understanding of your security posture across the entire organization. It can also help you improve the protection of your workloads, applications, and data.
The data lake is backed by Amazon Simple Storage Service (Amazon S3) buckets, and you retain ownership over your data.
Amazon Security Lake integrates with CloudTrail, a service that provides a record of actions taken by a user, role, or an Amazon Web Services service. In Security Lake, CloudTrail captures API calls for Security Lake as events. The calls captured include calls from the Security Lake console and code calls to the Security Lake API operations. If you create a trail, you can enable continuous delivery of CloudTrail events to an Amazon S3 bucket, including events for Security Lake. If you don't configure a trail, you can still view the most recent events in the CloudTrail console in Event history. Using the information collected by CloudTrail you can determine the request that was made to Security Lake, the IP address from which the request was made, who made the request, when it was made, and additional details. To learn more about Security Lake information in CloudTrail, see the Amazon Security Lake User Guide.
Security Lake automates the collection of security-related log and event data from integrated Amazon Web Services and third-party services. It also helps you manage the lifecycle of data with customizable retention and replication settings. Security Lake converts ingested data into Apache Parquet format and a standard open-source schema called the Open Cybersecurity Schema Framework (OCSF).
Other Amazon Web Services and third-party services can subscribe to the data that's stored in Security Lake for incident response and security data analytics.
To install the this package, simply type add or install @aws-sdk/client-securitylake using your favorite package manager:
npm install @aws-sdk/client-securitylake
yarn add @aws-sdk/client-securitylake
pnpm add @aws-sdk/client-securitylake
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the SecurityLakeClient
and
the commands you need, for example ListDataLakesCommand
:
// ES5 example
const { SecurityLakeClient, ListDataLakesCommand } = require("@aws-sdk/client-securitylake");
// ES6+ example
import { SecurityLakeClient, ListDataLakesCommand } from "@aws-sdk/client-securitylake";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new SecurityLakeClient({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListDataLakesCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-securitylake";
const client = new AWS.SecurityLake({ region: "REGION" });
// async/await.
try {
const data = await client.listDataLakes(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listDataLakes(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listDataLakes(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-securitylake
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
FAQs
AWS SDK for JavaScript Securitylake Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-securitylake receives a total of 78,279 weekly downloads. As such, @aws-sdk/client-securitylake popularity was classified as popular.
We found that @aws-sdk/client-securitylake demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.