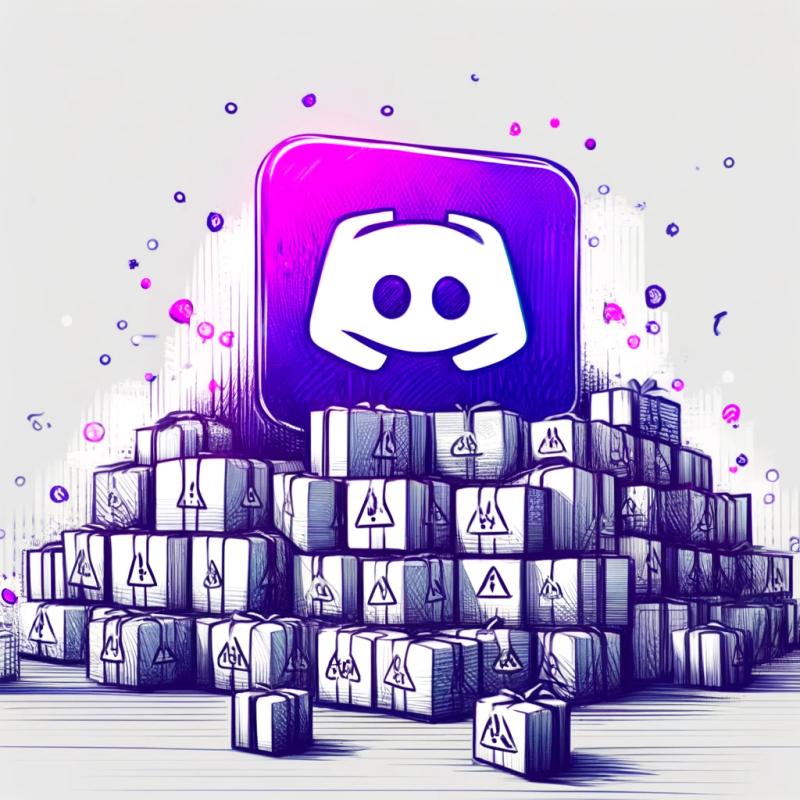
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
@axis-llc/mpesa
Advanced tools
Readme
This is a simple wrapper for Mpesa Daraja API using typescript
npm install --save @axis-llc/mpesa
yarn add @axis-llc/mpesa
Term | Description | Type |
---|---|---|
env | Your API environment. Either `sandbox` or 'live' | string |
type | Identifier type 2 for Till, 4 for Paybill | number |
store | Store number if using a till number | number |
shortcode | Your Buy Goods number or Paybill | number |
key | App consumer key from Daraja | string |
secret | App consumer secret from Daraja | string |
passkey | Your online passkey | string |
username | Org portal username | string |
password | Org portal password | string |
validationUrl | A valid secure URL that is used to validate your transaction details | string |
confirmationUrl | A valid secure URL that is used to receive payment notifications from C2B API. | string |
callbackUrl | A valid secure URL that is used to receive payment notifications from M-Pesa API. | string |
timeoutUrl | This is the URL to be specified in your request that will be used by API Proxy to send notification incase the payment request is timed out while awaiting processing in the queue. | string |
resultsUrl | This is the URL to be specified in your request that will be used by M-PESA to send notification upon processing of the payment request. | string |
import { Mpesa, useMpesa } from '@axis-llc/mpesa';
or
const { Mpesa, useMpesa } = require('@axis-llc/mpesa');
const mpesa = new Mpesa(
{
env //"sandbox",
type //4,
shortcode //174379,
store //174379,
key // Your app consumer key,
secret // Your app consumer secret,
username // Your M-Pesa org username,
password // Your M-Pesa org pass,
passkey // Your online passkey "bfb279f9aa9bdbcf158e97dd71a467cd2e0c893059b10f78e6b72ada1ed2c919",
validationUrl //"/lipwa/validate",
confirmationUrl //"/lipwa/confirm",
callbackUrl //"/lipwa/reconcile",
timeoutUrl //"/lipwa/timeout",
resultUrl //"/lipwa/results",
}
)
Or, individual APIs
const { stkPush, registerUrls } = useMpesa(
{
env //"sandbox",
type //4,
shortcode //174379,
store //174379,
key // Your app consumer key,
secret // Your app consumer secret,
username // Your M-Pesa org username,
password // Your M-Pesa org pass,
passkey // Your online passkey "bfb279f9aa9bdbcf158e97dd71a467cd2e0c893059b10f78e6b72ada1ed2c919",
validationUrl //"/lipwa/validate",
confirmationUrl //"/lipwa/confirm",
callbackUrl //"/lipwa/reconcile",
timeoutUrl //"/lipwa/timeout",
resultUrl //"/lipwa/results",
}
)
mpesa
.stkPush(
254705459494,
10,
'ACCOUNT', // You can ignore this, the code will generate a unique string
'Transaction Description', // Optional
'Remark', // optional
)
.then(({ error, data }) => {
if (data) {
const {
MerchantRequestID,
CheckoutRequestID,
ResponseCode,
ResponseDescription,
CustomerMessage,
} = data;
console.log(MerchantRequestID);
}
if (error) {
const { errorCode, errorMessage } = error;
console.log(errorCode, errorMessage);
}
});
// Or use the API directly
stkPush(
254705459494,
10,
'ACCOUNT', // You can ignore this, the code will generate a unique string
'Transaction Description', // Optional
'Remark', // optional
).then(({ error, data }) => {
if (data) {
const {
MerchantRequestID,
CheckoutRequestID,
ResponseCode,
ResponseDescription,
CustomerMessage,
} = data;
console.log(MerchantRequestID);
}
if (error) {
const { errorCode, errorMessage } = error;
console.log(errorCode, errorMessage);
}
});
Or, if inside an async function
async () => {
const {
error: { errorCode, errorMessage },
data: {
MerchantRequestID,
CheckoutRequestID,
ResponseCode,
ResponseDescription,
CustomerMessage,
},
} = await mpesa.stkPush(
254705459494,
10,
'ACCOUNT',
'Transaction Description',
'Remark',
);
console.log(MerchantRequestID);
// TIP: Save MerchantRequestID and update when you receive the IPN
};
mpesa.registerUrls('Completed' | 'Cancelled').then(({ error, data }) => {
if (data) {
const { ResponseCode, ResponseDescription } = data;
console.log(ResponseDescription);
}
if (error) {
const { errorCode, errorMessage } = error;
console.log(errorCode, errorMessage);
}
});
mpesa
.sendB2C(
phone,
amount,
'BusinessPayment' | 'SalaryPayment' | 'PromotionPayment',
'Some remark',
'Some occasion',
)
.then(({ error, data }) => {
if (data) {
const {
ConversationID,
OriginatorConversationID,
ResponseCode,
ResponseDescription,
} = data;
console.log(OriginatorConversationID);
// TIP: Save `OriginatorConversationID` in the database, and use it as a key once you receive the IPN
}
if (error) {
const { errorCode, errorMessage } = error;
console.log(errorCode, errorMessage);
}
});
mpesa
.sendB2B(
phone,
amount,
'BusinessPayBill' |
'BusinessBuyGoods' |
'DisburseFundsToBusiness' |
'BusinessToBusinessTransfer' |
'MerchantToMerchantTransfer',
'Some remark',
'Some occasion',
)
.then(({ error, data }) => {
if (data) {
const {
ConversationID,
OriginatorConversationID,
ResponseCode,
ResponseDescription,
} = data;
console.log(OriginatorConversationID);
// TIP: Save `OriginatorConversationID` in the database, and use it as a key for update
}
if (error) {
const { errorCode, errorMessage } = error;
console.log(errorCode, errorMessage);
}
});
FAQs
M-Pesa API Typescript SDK
We found that @axis-llc/mpesa demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.