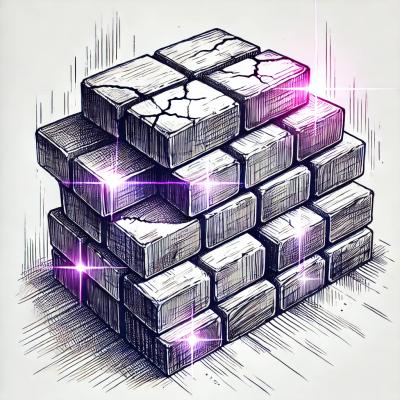
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@bem/bemjson-node
Advanced tools
BEM tree node representation.
$ npm install --save @bem/bemjson-node
const BemjsonNode = require('@bem/bemjson-node');
const bemjsonNode = new BemjsonNode({ block: 'button', elem: 'text' });
bemjsonNode.block; // button
bemjsonNode.elem; // text
bemjsonNode.mods; // {}
bemjsonNode.elemMods; // {}
Parameter | Type | Description |
---|---|---|
block | string | The block name of entity. |
mods | object | An object of modifiers for block entity. Optional. |
elem | string | The element name of entity. Optional. |
elemMods | object | An object of modifiers for element entity. Should not be used without elem field. Optional. |
mix | string , object or array | An array of mixed bemjson nodes. From passed strings and objects will be created bemjson node objects. Optional. |
const BemjsonNode = require('@bem/bemjson-node');
// The block with modifier
new BemjsonNode({
block: 'button',
mods: { view: 'action' }
});
// The element inside block with modifier
new BemjsonNode({
block: 'button',
mods: { view: 'action' },
elem: 'inner'
});
// The element node with modifier
new BemjsonNode({
block: 'button',
elem: 'icon',
elemMods: { type: 'load' }
});
// The block with a mixed element
new BemjsonNode({
block: 'button',
mix: { block: 'button', elem: 'text' }
});
// Invalid value in mods field
new BemjsonNode({
block: 'button',
mods: 'icon'
});
// ➜ AssertionError: @bem/bemjson-node: `mods` field should be a simple object or null.
The name of block to which entity in this node belongs.
const BemjsonNode = require('@bem/bemjson-node');
const name = new BemjsonNode({ block: 'button' });
name.block; // button
The name of element to which entity in this node belongs.
Important: Contains null
value if node is a block entity.
const BemjsonNode = require('@bem/bemjson-node');
const node1 = new BemjsonNode({ block: 'button' });
const node2 = new BemjsonNode({ block: 'button', elem: 'text' });
node1.elem; // null
node2.elem; // "text"
The object with modifiers of this node.
Important: Contains modifiers of a scope (block) node if this node IS an element.
const BemjsonNode = require('@bem/bemjson-node');
const blockNode = new BemjsonNode({ block: 'button' });
const modsNode = new BemjsonNode({ block: 'button', mods: { disabled: true } });
const elemNode = new BemjsonNode({ block: 'button', mods: { disabled: true }, elem: 'text' });
blockNode.mods; // { }
elemNode.mods; // { disabled: true }
modsNode.mods; // { disabled: true }
The object with modifiers of this node.
Important: Contains null
if node IS NOT an element.
const BemjsonNode = require('@bem/bemjson-node');
const blockNode = new BemjsonNode({ block: 'button' });
const modsNode = new BemjsonNode({ block: 'button', mods: { disabled: true } });
const elemNode = new BemjsonNode({ block: 'button', elem: 'text' });
const emodsNode = new BemjsonNode({ block: 'button', elem: 'text', elemMods: { highlighted: true } });
blockNode.elemMods; // null
modsNode.elemMods; // null
elemNode.elemMods; // { }
emodsNode.elemMods; // { disabled: true }
Returns normalized object representing the bemjson node.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({ block: 'button', mods: { focused: true }, elem: 'text' });
node.valueOf();
// ➜ { block: 'button', mods: { focused: true }, elem: 'text', elemMods: { } }
Returns raw data for JSON.stringify()
purposes.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({ block: 'input', mods: { available: true } });
JSON.stringify(node); // {"block":"input","mods":{"available":true}}
Returns string representing the bemjson node.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({
block: 'button', mods: { focused: true },
mix: { block: 'mixed', mods: { bg: 'red' } }
});
node.toString(); // "button _focused mixed _bg_red"
Determines whether specified object is an instance of BemjsonNode.
Parameter | Type | Description |
---|---|---|
bemjsonNode | * | The object to check. |
const BemjsonNode = require('@bem/bemjson-node');
const bemjsonNode = new BemjsonNode({ block: 'input' });
BemjsonNode.isBemjsonNode(bemjsonNode); // true
BemjsonNode.isBemjsonNode({ block: 'button' }); // false
The BemjsonNode
has toJSON
method to support JSON.stringify()
behaviour.
Use JSON.stringify
to serialize an instance of BemjsonNode
.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({ block: 'input', mod: 'available' });
JSON.stringify(node); // {"block":"input","mods":{"available":true}}
Use JSON.parse
to deserialize JSON string and create an instance of BemjsonNode
.
const BemjsonNode = require('@bem/bemjson-node');
const str = '{"block":"input","mods":{"available"::true}}';
new BemjsonNode(JSON.parse(str)); // BemjsonNode({ block: 'input', mods: { available: true } });
In Node.js, console.log()
calls util.inspect()
on each argument without a formatting placeholder.
BemjsonNode
has inspect()
method to get custom string representation of the object.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({ block: 'input', mods: { available: true } });
console.log(node);
// ➜ BemjsonNode { block: 'input', mods: { available: true } }
You can also convert BemjsonNode
object to string
.
const BemjsonNode = require('@bem/bemjson-node');
const node = new BemjsonNode({ block: 'input', mods: { available: true } });
console.log(`node: ${node}`);
// ➜ node: input _available
Code and documentation © 2017 YANDEX LLC. Code released under the Mozilla Public License 2.0.
FAQs
BEM tree node representation
We found that @bem/bemjson-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.