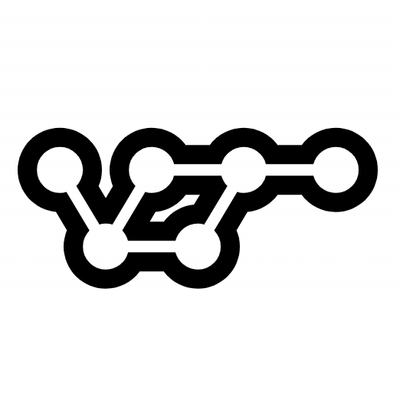
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@bigbinary/neeto-access-control-frontend
Advanced tools
The neeto-access-control-nano
manages access control across neeto products.
The nano exports the @bigbinary/neeto-access-control-frontend
NPM package
and neeto-access-control-engine
Rails engine for development.
The engine is used to manage access control across neeto products.
Add the gem in Gemfile
source "NEETO_GEM_SERVER_URL" do
# ..existing gems
gem "neeto-access-control-engine"
end
Install the gem
bundle install
Add required migrations in the db/migrate
folder. Run the following
commands to copy the migrations from the engine to the host application. Ex:
neetoForm
bundle exec rails neeto_access_control_engine:install:migrations
bundle exec rails db:migrate
Add this line to your application's config/routes.rb
file:
mount NeetoAccessControlEngine::Engine => "/secure"
Add has_one
association to the model you want to add the access control
configuration. For Example - The organization only needs single access
control.
class Organization < ApplicationRecord
has_one :access_control_configuration, as: :owner, class_name: "NeetoAccessControlEngine::Configuration", dependent: :destroy
end
Your controller must include the concern and access_control_configuration method.
class PublicController < ApplicationController
.
.
include NeetoAccessControlEngine::Authenticate
.
private
def access_control_configuration
@_access_control_configuration ||= @organization.access_control_configuration
end
end
Add overrides for the following files:
7.1. google_controller_override.rb
NeetoAccessControlEngine::GoogleController.class_eval do
private
def access_control_configuration
@_access_control_configuration ||= @organization.access_control_configuration
end
end
7.2. invitations_controller_override.rb (Optional)
access_control_configuration
after_invitations_created
If host app doesn't have an override method for the controller, then
neeto_access_control_configuration_id
parameter must be included in the request params.
NeetoAccessControlEngine::Api::V1::Configurations::InvitationsController.class_eval do
private
def access_control_configuration
@_access_control_configuration ||= @organization.access_control_configuration
end
# after_action callback, called after invitations are created.
# Use to send invitation emails or perform any post-invitation tasks.
def after_invitations_created
# @invited_emails is loaded in engine
return if @invited_emails.blank?
EmailInvitationsJob.perform_async(@invited_emails, current_user.id)
end
end
7.3. guest_sessions_controller_override.rb
NeetoAccessControlEngine::GuestSessionsController.class_eval do
private
def access_control_configuration
@_access_control_configuration ||= @organization.access_control_configuration
end
end
7.4. configurations_controller_override.rb
NeetoAccessControlEngine::Api::V1::ConfigurationsController.class_eval do
private
def owner
@organization
end
def update_owner!
# Optional override to update the owner if required when configuration is updated
end
end
8.1. Create Google OAuth account
Watch the demo video
for step-by-step instructions on creating a Google OAuth account. Add the
authorised redirect URI:
https://connect.neetokb.net/secure/google_oauth2/callback
8.2. Set the env variables Add the following secret values to
config/secrets.yml
.
google:
client_id: <%= ENV['GOOGLE_OAUTH_CLIENT_ID'] %>
client_secret: <%= ENV['GOOGLE_OAUTH_CLIENT_SECRET'] %>
neeto-access-control-frontend
package using the below
command:
yarn add @bigbinary/neeto-access-control-frontend
Configuration
(source code)Props
ownerId
: To specify the ID of the instance of the model. The ID is used to
fetch and update access control configuration.ownerType
: Type of the model.onReset
: Callback function that is triggered when the reset button is
clicked.onSuccess
: Callback function that is triggered when the configuration is
successfully updated.handleCancel
: Callback function that is triggered when the cancel button is
clicked.invitationsTableExtraColumnsData
: Prop to add column data for invitations
table. This will override the existing column if the key is already present.
Refer
Column dataonConfigurationChangedToInviteOnly
: Callback function that is triggered when
configuration is changed to invited
. Default: noop
.headerProps
: Props passed to the
Header
component from neetoMolecules.hideAddEmailsFromInvitationPane
: Prop to hide the add emails form from
invitation pane. Default: false
.className
: Additional classes to be added to the form.onDestroyInvitationsSuccess
: Callback function that is triggered when the
invitations are successfully destroyed.inviteButtonProps
: If specified, this will show a button on the top of the
Pane. It can be used to take the users to invitation forms outside the
InvitationsPane.additionalFieldsInitialValues
: Prop to add additional custom fields to the
form.fieldsWrapper
: A component that wraps the fields in the form. Useful for
adding custom fields to the form.translationContext
: Context for translations, it can be used to override the
translations of the field labels. Ref:
https://www.i18next.com/translation-function/contexttransProps
: Props for the Trans
component used in the form field labels.
Each field label can be customized by passing the props in the transProps
object mapped to the auth type: no
, basic
, email
, session
, invited
.enableNavigationBlocking
: If true
, renders the BlockNavigation
component
from neetoUI to show alert when having unsaved changes in the form.Invitations
ObjectThe Invitations
object provides access to invitation-related components,
hooks, and constants.
InvitationsPane
(source code)Props
Check source code for default values
onClose
: Callback function that is triggered when the close button is
clicked.isOpen
: Prop for pane, will reflect state of the pane.extraColumnsData
: Prop to add column data for invitations table. This will
override the existing column if the key is already present. Refer
Column dataownerId
: To specify the ID of the instance of the model. The ID is used to
fetch and update access control configuration.ownerType
: Type of the model.hideAddEmailsForm
: Prop to hide the add emails form from invitation pane.configurationId
: Prop to specify the ID of the instance of the model. The ID
is used to load access control configuration. NOTE: If this prop is not
passed, make sure invitations_controller_override.rb
is added with
access_control_configuration
method as mentioned above in adding overrides
section.onDestroyInvitationsSuccess
: Callback function that is triggered when the
invitations are successfully destroyed.inviteButtonProps
: If specified, this will show a button on the top of the
Pane. It can be used to take the users to invitation forms outside the
InvitationsPane.useBulkCreateInvitations
source code: Bulk
create invitations.Check
usage.INVITATIONS_QUERY_KEY
: For react query cache of invitations/* Required imports */
import { Invitations } from "@bigbinary/neeto-access-control-frontend";
const { InvitationsPane, useBulkCreateInvitations, INVITATIONS_QUERY_KEY } =
Invitations;
const MyComponent = () => {
const [showInviationsPane, setShowInviationsPane] = useState(false);
const [emails, setEmails] = useState("");
const { mutateAsync: addEmails } = useBulkCreateInvitations({
configurationId: null,
});
const queryClient = useQueryClient();
const addTestEmails = async () => {
const payload = { emails: emails.split(",") };
await addEmails(payload);
setEmails("");
queryClient.invalidateQueries({
queryKey: [INVITATIONS_QUERY_KEY],
});
};
return (
<div className="space-4 p-5">
<Input
label="Email"
value={emails}
onChange={e => setEmails(e.target.value)}
/>
<Button label="Add test email " onClick={addTestEmails} />
<Button
label="Open Invitation pane"
onClick={() => setShowInviationsPane(true)}
/>
<InvitationsPane
hideAddEmailsForm
isOpen={showInviationsPane}
onClose={() => setShowInviationsPane(false)}
/>
</div>
);
};
Usage
This is an example usage in neetoKB
import React from "react";
import { Configuration } from "@bigbinary/neeto-access-control-frontend";
import { HELP_SECURITY_DOC_URL } from "src/constants/urls";
import { buildDefaultBreadcrumbs } from "./utils";
const AccessControl = () => {
return (
<Configuration
className="mx-auto max-w-3xl md:px-0 lg:px-6"
handleCancel={() => {}}
headerProps={{
title: "Access control",
description: "Access control description",
helpLink: HELP_SECURITY_DOC_URL,
breadcrumbs: buildDefaultBreadcrumbs({
title: "Access control",
}),
}}
/>
);
};
export default AccessControl;
useShowConfiguration
This is a React Query hook for fetching access control configuration of specified owner.
ownerId
: To specify the ID of the model instance. This ID will be used to
fetch access control configuration.ownerType
: Type of the model.const {
data: { configuration },
} = useShowConfiguration({ ownerId, ownerType });
To customize option labels for authentication, you can set the translations from the host application. To set the translations from the host application:
en.json
file.neetoAccessControl.auth
namespace. For Example:
{
"neetoAccessControl": {
"auth": {
"no": "No auth label",
"basic": "Basic auth label",
"email": "Email auth label"
"session": "Session auth label"
}
}
}
Consult the building and releasing packages guide for details on how to publish.
{latest-version}-beta
(if 1.2.34
is the
current latest version, it will be 1.2.34-beta
) in the target branch for
beta release.v
, eg:
v1.2.34-beta
.If we are releasing a beta version of a product, we have to inform the compliance team as well to avoid overwriting the version with the latest version on the next compliance release.
FAQs
Neeto access control
The npm package @bigbinary/neeto-access-control-frontend receives a total of 219 weekly downloads. As such, @bigbinary/neeto-access-control-frontend popularity was classified as not popular.
We found that @bigbinary/neeto-access-control-frontend demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.