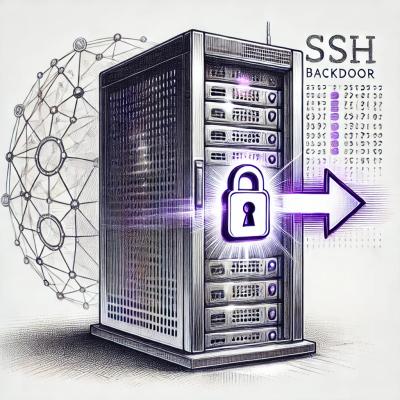
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@bikeshaving/crank
Advanced tools
JSX-based components with functions, promises and generators.
A JavaScript framework for writing JSX-driven components with functions, generators and promises.
I wrote a blog post describing the motivations and journey behind making Crank here. Crank is in an early beta, and some APIs may be changed.
A documentation is in the works, where we dogfood Crank. In the meantime you can access the docs as markdown files in the docs directory.
Crank uses the same JSX syntax and element diffing algorithm popularized by React, so you can write declarative code which looks like HTML directly in your JavaScript. The portability of elements means you can reuse the same code to render DOM nodes on the client and HTML strings on the server.
Crank uses functions and generators for components exclusively. No fancy classes, hooks, proxies or template languages are needed, and you can take advantage of the natural lifecycle of generators to write stateful setup, update and teardown logic all in the same scope.
Crank provides first-class support for promises by allowing components to be defined as async functions and generators. You can await
the rendering of async components just like any other async function, and you can even race renderings to create user interfaces with deferred loading states.
Crank is available on NPM in the ESModule and CommonJS formats.
$ npm install @bikeshaving/crank
/** @jsx createElement */
import {createElement} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
renderer.render(<div id="hello">Hello world</div>, document.body);
If your environment does not support ESModules (you’ll probably see a message like SyntaxError: Unexpected token export
), you can import the CommonJS versions of the library like so:
/** @jsx createElement */
import {createElement} from "@bikeshaving/crank/cjs";
import {renderer} from "@bikeshaving/crank/cjs/dom";
renderer.render(<div id="hello">Hello world</div>, document.body);
/* @jsx createElement */
import {createElement} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
function Greeting ({name = "World"}) {
return (
<div>Hello {name}</div>
);
}
renderer.render(<Greeting />, document.getElementById("app"));
/** @jsx createElement */
import {createElement} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
function *Timer () {
let seconds = 0;
const interval = setInterval(() => {
seconds++;
this.refresh();
}, 1000);
try {
while (true) {
yield <div>Seconds: {seconds}</div>;
}
} finally {
clearInterval(interval);
}
}
renderer.render(<Timer />, document.getElementById("app"));
/** @jsx createElement */
import {createElement} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
async function IPAddress () {
const res = await fetch("https://api.ipify.org");
const address = await res.text();
return <div>Your IP Address: {address}</div>;
}
renderer.render(<IPAddress />, document.getElementById("app"));
/** @jsx createElement */
import {createElement, Fragment} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
async function Fallback({wait = 1000, children}) {
await new Promise(resolve => setTimeout(resolve, wait));
return <Fragment>{children}</Fragment>;
}
async function RandomDog({throttle = false}) {
if (throttle) {
await new Promise(resolve => setTimeout(resolve, 2000));
}
const res = await fetch("https://dog.ceo/api/breeds/image/random");
const data = await res.json();
return (
<a href={data.message}>
<img src={data.message} alt="A Random Dog" width="300" />
</a>
);
}
async function* RandomDogLoader({throttle}) {
for await ({throttle} of this) {
yield (
<Fallback>
<div>Fetching a good boy...</div>
</Fallback>
);
yield <RandomDog throttle={throttle} />;
}
}
function* RandomDogs() {
let throttle = false;
this.addEventListener("click", (ev) => {
if (ev.target.tagName === "BUTTON") {
throttle = !throttle;
this.refresh();
}
});
while (true) {
yield (
<Fragment>
<div>
<button>Show me another dog.</button>
</div>
<RandomDogLoader throttle={throttle} />
</Fragment>
);
}
}
renderer.render(<RandomDogs />, document.body);
FAQs
Write JSX-driven components with functions, promises and generators.
The npm package @bikeshaving/crank receives a total of 179 weekly downloads. As such, @bikeshaving/crank popularity was classified as not popular.
We found that @bikeshaving/crank demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.