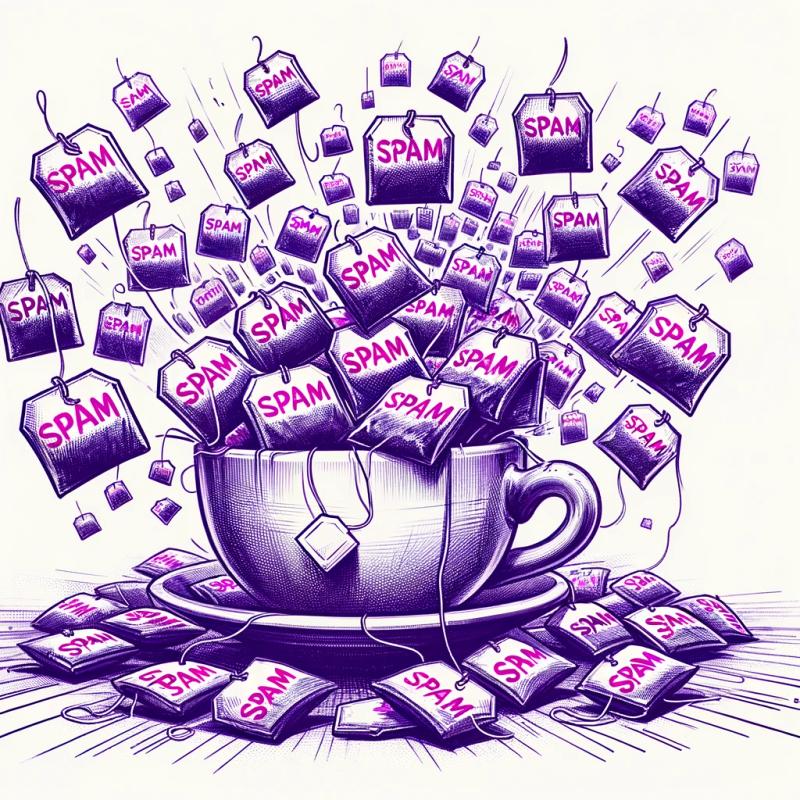
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@bluframe/blublocks
Advanced tools
Readme
Blu Frame UI Building Blocks provides a collection of customizable React components to speed up your web development process. π¨
If you are using yarn
:
yarn add @bluframe/blublocks
And if you are using npm
:
npm i @bluframe/blublocks
You can import the components individually:
import Button from "@bluframe/blublocks/Button";
Or import multiple components at once:
import { Button, InputText } from "@bluframe/blublocks";
This is a Badge component, often used to highlight a certain feature or aspect of a product or a piece of information.
className
: (optional) string - The class name for the badge wrapper.color
: (optional) $Keys - Defaults to "primary"
. The color of the badge. Colors are defined in our Palette type.label
: string - The text to display on the badge. import Badge from "@bluframe/blublocks/Badge"
function App() {
return (
<Badge
className="custom-class"
color="secondary"
label="Apples"
/>
)
}
A simple button component that can be styled to all needs.
bold
: (optional) boolean - If true
, the button label will be displayed in bold. Defaults to false
.className
: (optional) string - The class name for the button wrapper.disabled
: (optional) boolean - If true
, the button will be displayed in a disabled state. Defaults to false
.expanded
: (optional) boolean - If true
, the button will expand to fill its container's width. Defaults to false
.iconEnd
: (optional) React$Node - The icon to display at the end of the button label. πiconStart
: (optional) React$Node - The icon to display at the beginning of the button label. πicon
: (optional) React$Node - The icon to display when iconOnly
is true
. π―iconOnly
: (optional) boolean - If true
, the button will only display an icon. Defaults to false
.label
: string - The label for the button. π·οΈloading
: (optional) boolean - If true
, a loading spinner will be displayed at the end of the button. Defaults to false
.noHoverHighlight
: (optional) boolean - If true
, the button will not have a hover effect. Defaults to false
.onClick
: (optional) function - The callback that is fired when the button is clicked. π±οΈoutlined
: (optional) boolean - If true
, the button will be displayed with an outlined style. Defaults to false
.padded
: (optional) boolean - If true
, the button will have extra padding. Defaults to false
.secondary
: (optional) boolean - If true
, the button will be displayed with a secondary color. Defaults to false
.small
: (optional) boolean - If true
, the button will be displayed in a smaller size. Defaults to false
.transparent
: (optional) boolean - If true
, the button will be displayed with a transparent background. Defaults to false
.type
: (optional) string - The type attribute for the button element. Defaults to "button"
.import Button from "@bluframe/blublocks/Button";
function App() {
const handleClick = () => {
console.log("Button clicked");
};
return (
<Button
bold={true}
buttonType="button"
disabled={false}
expanded={false}
iconEnd={<Icon name="IoArrowForward" />}
iconStart={<Icon name="IoArrowBack" />}
label="Click me"
loading={false}
noHoverHighlight={false}
onClick={handleClick}
outlined={false}
padded={true}
secondary={false}
small={false}
/>
);
}
All our input components support ARIA attributes. π―
A customizable input text component that supports ARIA attributes.
className
: (optional) string - The class name for the input text wrapper.defaultValue
: (optional) string - The default value attribute for the input element. πerror
: (optional) boolean - If true
, the input text field will be displayed in an error state. Defaults to false
. β οΈhelperText
: (optional) string - The helper text to display below the input text field. If the 'error' prop is true
, this text will be displayed in the error color. πinputId
: (optional) string - The id attribute for the input element. πisFullBorder
: (optional) boolean - If true
, the input text field will have a full border instead of just a bottom border. Defaults to false
.label
: string - The label for the input text. π·οΈname
: string - The name attribute for the input element.onBlur
: (optional) function - The callback that is fired when the input text loses focus. π¨onChange
: function - The callback that is fired when the input text value changes. πrequired
: (optional) boolean - If true
, the input will have the "required" attribute. Defaults to false
.step
: (optional) string - The step attribute for the input element. This is applicable when the input type is number
. πtype
: (optional) string - The type attribute for the input element. Defaults to text
.value
: string - The value of the input text.import InputText from "@bluframe/blublocks/InputText";
function App() {
const [value, setValue] = useState("");
const [error, setError] = useState(false);
const handleBlur = (event) => {
if (event.target.value === "") {
setError(true);
} else {
setError(false);
}
};
const handleChange = (event) => {
setValue(event.target.value);
};
return (
<InputText
error={error}
helperText={error ? "This field is required" : ""}
inputId="name-input"
label="Name"
name="name"
required
onBlur={handleBlur}
onChange={handleChange}
value={value}
/>
);
}
A customizable select component that supports ARIA attributes, automatically selects the first option if no selected option is provided, and provides a dropdown with a list of options.
className
: (optional) string - The class name for the select wrapper.defaultValue
: (optional) string - The default value of the select component. πlabel
: React$Node - The label for the select. π·οΈname
: string - The name attribute for the input element.onBlur
: (optional) function - The callback that is fired when the select loses focus. π¨onChange
: function - The callback that is fired when an option is selected.options
: Option[] - An array of options for the select. Each option should have a label
and a value
.placeholder
: (optional) string - The placeholder text to display when no option is selected. πvalue
: (optional) string - The currently selected value in the select. If not provided, the first option will be selected by default.import Select from "@bluframe/blublocks/Select";
function App() {
const [selectedValue, setSelectedValue] = useState(null);
const options = [
{
label: "Apple",
value: "apple"
},
{
label: "Orange",
value: "orange"
},
// ... more options
];
const handleBlur = () => {
console.log("Select lost focus");
};
const handleChange = (newValue) => {
setSelectedValue(newValue);
};
return (
<Select
label="Fruit"
name="fruit"
onBlur={handleBlur}
onChange={handleChange}
options={options}
placeholder="Select a fruit"
value={selectedValue}
/>
);
}
A customizable radio component that supports ARIA attributes. π»
inputId
: (optional) string - The id attribute for the input element. πisChecked
: boolean - Determines whether the radio is checked or not. β
label
: React$Node - The label for the radio. π·οΈname
: string - The name attribute for the input element.onChange
: function - The callback that is fired when the radio is clicked. π±οΈvalue
: OptionValue - The value of the radio.import Radio from "@bluframe/blublocks/Radio";
function App() {
const [selectedValue, setSelectedValue] = useState("apple");
const handleChange = (newValue) => {
setSelectedValue(newValue);
};
return (
<>
<Radio
inputId="apple-radio"
isChecked={selectedValue === "apple"}
label="Apple"
name="fruit"
onChange={handleChange}
value="apple"
/>
<Radio
inputId="orange-radio"
isChecked={selectedValue === "orange"}
label="Orange"
name="fruit"
onChange={handleChange}
value="orange"
/>
</>
);
}
A customizable radio group component that supports ARIA attributes and automatically selects the first option if no selected option is provided. ποΈ
className
: (optional) string - The class name for the radio group wrapper.label
: React$Node - The label for the radio group. π·οΈname
: string - The name attribute for the input elements.onChange
: function - The callback that is fired when a radio within the group is clicked. π±οΈoptions
: Option[] - An array of options for the radio group. Each option should have a label
and a value
.selected
: (optional) OptionValue | null - The currently selected value in the radio group. If not provided, the first option will be selected by default.import RadioGroup from "@bluframe/blublocks/RadioGroup";
function App() {
const [selectedValue, setSelectedValue] = useState(null);
const handleChange = (newValue) => {
setSelectedValue(newValue);
};
const options = [
{
label: "Apple",
value: "apple"
},
{
label: "Banana",
value: "banana"
}
];
return (
<RadioGroup
label="Select a fruit"
name="fruit"
onChange={handleChange}
options={options}
selected={selectedValue}
/>
);
}
A customizable checkbox component that supports ARIA attributes. βοΈ
inputId
: (optional) string - The id attribute for the input element. πisChecked
: boolean - Determines whether the checkbox is checked or not. β
label
: React$Node - The label for the checkbox. π·οΈname
: string - The name attribute for the input element.value
: OptionValue - The value of the checkbox.onChange
: function - The callback that is fired when the checkbox is clicked. π±οΈimport Checkbox from "@bluframe/blublocks/Checkbox";
function App() {
const [isChecked, setIsChecked] = useState(true);
const handleChange = (value) => {
setIsChecked(!isChecked);
};
return (
<Checkbox
inputId="apple-checkbox"
isChecked={isChecked}
label="Apple"
name="fruit"
value="apple"
onChange={handleChange}
/>
);
}
A versatile TextArea component for those larger inputs. Supports customization and theming.
className
: (optional) string - The class name for the textarea wrapper.cols
: (optional) number - The visible width of the text control, in average character widths. Defaults to 50
.defaultValue
: (optional) string - The default value of the textarea.inputId
: (optional) string - The id attribute for the textarea element. πlabel
: (optional) React$Node - The label for the textarea.labelId
: (optional) string - The id for the label element.name
: (required) string - The name attribute for the textarea element.onBlur
: (optional) function - The callback that is fired when the textarea loses focus.onChange
: (required) function - The callback that is fired when the content of the textarea changes.placeholder
: (optional) string - The placeholder text for the textarea.required
: (optional) boolean - If true
, the textarea will be required.rows
: (optional) number - The number of visible text lines for the control. Defaults to 10
.value
: (optional) string - The value of the textarea.import TextArea from "@bluframe/blublocks/TextArea";
function App() {
const [content, setContent] = useState("");
const handleChange = (event) => {
setContent(event.target.value);
};
return (
<TextArea
inputId="my-textarea"
label="My Textarea"
name="myTextArea"
onChange={handleChange}
placeholder="Start typing..."
value={content}
/>
);
}
A secure and customizable login component.
isLoading
: (optional) boolean - Indicates if the login process is ongoing. Defaults to false
.name
: (optional) string - Displays a welcome message or heading above the login form.onSubmitLogin
: function - The callback function that is executed when the login form is submitted. It should handle the actual login logic, like API calls.import { useState } from "react";
import Login from "@bluframe/blublocks/Login";
function App() {
const [isLoading, setIsLoading] = useState(false);
const handleSubmitLogin = ({ username, password }) => {
console.log(username, password);
setIsLoading(true);
// Here you can handle the login logic, e.g., making an API call.
setIsLoading(false);
};
return (
<Login
isLoading={isLoading}
name="Welcome to Our App"
onSubmitLogin={handleSubmitLogin}
/>
);
}
The Login
component makes it simple to add a login form to your application. It supports loading states and customization for a welcome message. This component requires you to handle the actual login logic in the onSubmitLogin
prop, where you receive the username and password entered by the user.
Like other components in the Blu Frame UI Building Blocks library, the Login
component can be customized in terms of styling and appearance. You can override the default styles using styled-components or CSS classes to match your application's branding and design requirements.
Beautiful and customizable typography components. βοΈ
import H1 from "@bluframe/blublocks/Typography/H1";
import SubtitleH1 from "@bluframe/blublocks/Typography/SubtitleH1";
function App() {
return (
<>
<H1>This is a Heading</H1>
<SubtitleH1>This is a Subtitle</SubtitleH1>
</>
);
}
BluFrame UI Building Blocks makes it easy to customize the styling of the typography components according to your needs. You can override the default styles using styled-components and theming. π¨
Here's an example of how to create a custom H1 component with your own styles:
// CustomH1.js
import styled from "styled-components";
import H1 from "@bluframe/blublocks/Typography/H1";
const CustomH1 = styled(H1)`
color: #ff0000;
font-family: "Comic Sans MS", cursive, sans-serif;
font-weight: 400;
`;
export default CustomH1;
And then you can use your custom H1 component in your app:
import CustomH1 from "./CustomH1";
function App() {
return <CustomH1>This is a custom Heading</CustomH1>;
}
A versatile component that accepts markdown and beautifully displays it on your page. π
className
: (optional) string - The class name for the content wrapper.components
: (optional) ContentComponents - Custom components to override default markdown components.content
: string - The markdown content to be displayed.max
: (optional) number - The maximum number of characters to display from the content. Defaults to Infinity
. πparagraphAs
: (optional) string - The HTML tag to use for rendering paragraphs. Defaults to "p"
.stripMarkdown
: (optional) boolean - If true
, all markdown formatting will be stripped from the content. Defaults to false
.import Content from "@bluframe/blublocks/Content";
function App() {
const markdownContent = `
# This is a heading
This is a paragraph with **bold** and *italic* text.
- This is a list item
- Another list item
`;
return (
<Content
className="custom-class"
content={markdownContent}
max={100}
paragraphAs="div"
stripMarkdown={false}
/>
);
}
A modal component to manage and display overlay content.
children
: React$Node - The content to display inside the modal.isOpen
: boolean - Determines whether the modal is open or not.onClose
: function - The callback that is fired when the modal is closed.import Modal from "@bluframe/blublocks/Modal";
function App() {
const [isOpen, setIsOpen] = useState(false);
const handleOpen = () => setIsOpen(true);
const handleClose = () => setIsOpen(false);
return (
<div>
<button onClick={handleOpen}>Open Modal</button>
<Modal isOpen={isOpen} onClose={handleClose}>
<p>Modal Content!</p>
</Modal>
</div>
);
}
Our Bio component is a versatile, customizable block that allows you to display a biography section with ease. Perfect for about pages, team member profiles and more. π
avatar
: (required) string | React$Node - A link to an avatar image or a React component to display as the avatar.description
: (required) string - A string containing the bio description. This description can include markdown format text.name
: (required) string - The name to be displayed.color
: (optional) string - A color definition for the bio content links.First, import the Bio component from blublocks:
import Bio from "@bluframe/blublocks/Bio"
Use the Bio component in your app:
function App() {
return (
<Bio
avatar="https://somelinktoanimage.jpg"
description="This is my awesome bio!"
name="John Doe" />
)
}
The avatar prop can accept a URL string indicating the path to an image:
<Bio
avatar="https://somelinktoanimage.jpg"
description="This is my awesome bio!"
name="John Doe"
/>
Alternatively, the avatar prop can accept a React component:
import CustomAvatar from "./CustomAvatar"
<Bio
avatar={<CustomAvatar />}
description="This is an awesome bio!"
name="John Doe"
/>
The description prop supports markdown formatting. Here's an example:
<Bio
avatar="https://somelinktoanimage.jpg"
description="This is my *awesome* bio! [Visit my website!](https://mywebsite.com)"
name="John Doe"
/>
This will render the description with italic text and a hyperlink to the website.
If you want to customize the appearance even further, you can use styled-components to override the styles. Here's an example how to create a custom Bio component with extra styles:
import styled from "styled-components";
import Bio from "@bluframe/blublocks/Bio";
const CustomBio = styled(Bio)`
font-family: "Comic Sans MS", cursive, sans-serif;
color: #ff0000;
`;
function App() {
return (
<CustomBio
avatar="https://somelinktoanimage.jpg"
description="This is my awesome bio!"
name="John Doe"
/>
);
}
A classic Hamburger component made with 100% CSS. π
isMenuExpanded
: (optional) boolean - Determines whether the menu is expanded or not. Defaults to false
.menuId
: (optional) string - The id attribute for the menu that the Hamburger controls. πonClick
: (optional) function - The callback that is fired when the Hamburger is clicked. π±οΈimport Hamburger from "@bluframe/blublocks/Hamburger";
function App() {
const [isMenuExpanded, setIsMenuExpanded] = useState(false);
const handleHamburgerClick = () => {
setIsMenuExpanded(!isMenuExpanded);
};
return (
<Hamburger
isMenuExpanded={isMenuExpanded}
menuId="main-navigation"
onClick={handleHamburgerClick}
/>
);
}
We welcome contributions to improve Blu Frame UI Building Blocks! If you'd like to contribute, please follow these steps: π€
Please ensure that your code follows our coding style and passes all tests. π
Blu Frame UI Building Blocks is MIT licensed.
FAQs
Blu Frame UI Components Library (Blu Blocks)
The npm package @bluframe/blublocks receives a total of 7 weekly downloads. As such, @bluframe/blublocks popularity was classified as not popular.
We found that @bluframe/blublocks demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.