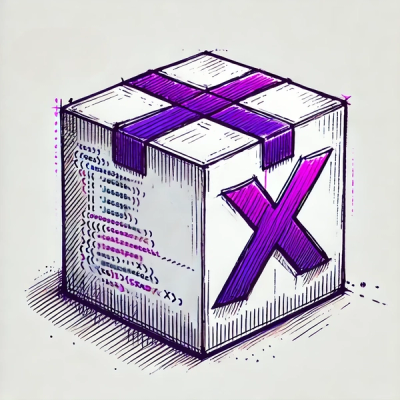
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@botmock/client
Advanced tools
@botmock/client
This package is used to extract data from Botmock projects.
The package is available to install on npm.
npm install @botmock/client
The following guides assumes a TypeScript environment with LTS Node.js installed.
Note: You can bootstrap such an environment by running
npx @botmock/cli
Note: Tokens and ids are created and accessed using the Botmock dashboard
Let's walk through building a small Node.js app that fetches data from multiple Botmock projects.
// index.ts
import { Client } from "@botmock/client";
const client = new Client();
(async () => {
const projects = await client
.withToken("YOUR_TOKEN")
.forTeam("YOUR_TEAM_ID")
.withProjectIds(["PROJECT_ID_1", "PROJECT_ID_2"])
.getProjects();
console.log(projects); // array of project objects
})();
Running ts-node index.ts
should print an array of project objects.
Client
Once a new instance of Client
is made, the following chaining methods can be chained together to build requests.
.withToken(string)
.forTeam(string)
.onBoard(string)
.withProjectIds(string[])
To execute requests, the following methods can be used at the end of a build chain. Listed below each of these dispatch methods are the required chaining methods.
.getProjects()
.withToken(string
)`.forTeam(string)
.withProjectIds(string[])
.getBoards()
.withToken(string
)`.forTeam(string)
.onBoard(string)
.withProjectIds(string[])
.getTeams()
.withToken()
.forTeam(string)
.withProjectIds(string[])
.getPaths()
.withToken(string
)`.forTeam(string)
.onBoard(string)
.withProjectIds(string[])
getResources
As an alternative to the chaining approach outlined above, resources can be fetched in a single method call.
Returns a Promise
which resolves an object containing payloads for each of resources
provided in the options object.
The options object contains the following.
Key name | Type | Description |
---|---|---|
teamId | string | id of the team |
projectId | string | id of the project |
boardId | string | (optional) id of the board |
resources | string[] | array of "project", "team", "board", "intents", "variables", or "entities" |
import { Client, Resource } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const { project, team, board, intents, variables, entities } = await client.getResources({
teamId: "YOUR_TEAM_ID",
projectId: "YOUR_PROJECT_ID",
boardId: "YOUR_BOARD_ID",
resources: [
Resource.PROJECT,
Resource.TEAM,
Resource.BOARD,
Resource.INTENTS,
Resource.VARIABLES,
Resource.ENTITIES,
]
});
// Each destructured property above contains that slice of data from the Botmock project specified in the options object.
})();
The object returned by getResources
can look like the following.
{
"project": {
"id": "637882f0-93ab-11ea-8c14-4d6810dd96fc",
"name": "complex",
"type": "flow",
"platform": "multi-channel",
"channels": [
"generic"
],
"locales": [
"en"
],
"created_at": {
"date": "2020-05-11 17:18:10.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"updated_at": {
"date": "2020-06-26 16:39:01.000000",
"timezone_type": 3,
"timezone": "UTC"
}
},
"team": {
"id": 1831,
"name": "Botmock Team",
"photo": "http://az6wlss5f1.cloud.wal-mart.com/storage/profiles/Wp5VXSQ4LUTSkfLKlH7IIOgXmkDcQh5OXhaghtFv.png",
"created_at": {
"date": "2017-08-12 03:38:19.000000",
"timezone_type": 3,
"timezone": "UTC"
}
},
"board": {
"board": {
"root_messages": [
"637a5d70-93ab-11ea-a217-33512b13de41"
],
"messages": [
{
"message_id": "637a5f60-93ab-11ea-a478-8debb9e9d485",
"message_type": "response",
"next_message_ids": [
{
"message_id": "27074d90-b3df-44b3-8e4e-c9b8802dad61",
"action": "*",
"intent": {
"label": "give_party_size",
"value": "da7ccf40-b7ca-11ea-8ff6-c37e8170426d"
},
"conditional": {
"id": "g-DsAWYCMF1acBzPXVRVi0z",
"rules": [],
"combinator": "and",
"not": false
}
}
],
"previous_message_ids": [
{
"message_id": "637a5d70-93ab-11ea-a217-33512b13de41",
"action": "*"
}
],
"is_root": false,
"node_name": "Bot Says",
"payload": {
"en": {
"generic": {
"blocks": [
{
"component_type": "text",
"nodeName": "Bot Says",
"context": [
{
"id": "a0a11bd0-b7ca-11ea-a1cf-4155954aa7c8",
"name": "%name%",
"type": "text",
"entity": "",
"default_value": "",
"start_index": 3
}
],
"text": "Hi %name%, how many for your reservation?",
"audio_file": "",
"ssml": "",
"delay": 2000,
"alternate_replies": [
"one"
]
}
]
}
}
}
}
]
},
"slots": {},
"variables": [
{
"id": "a0a11bd0-b7ca-11ea-a1cf-4155954aa7c8",
"name": "name",
"default_value": "",
"type": "text",
"entity": "",
"created_at": {
"date": "2020-06-26 16:32:29.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"updated_at": {
"date": "2020-06-26 16:32:29.000000",
"timezone_type": 3,
"timezone": "UTC"
}
}
],
"created_at": {
"date": "2020-05-11 17:18:10.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"updated_at": {
"date": "2020-06-26 16:36:24.000000",
"timezone_type": 3,
"timezone": "UTC"
}
},
"intents": [
{
"id": "1fbe2430-b7cb-11ea-86a3-61a8fe026c9c",
"name": "done",
"utterances": {
"en": [
{
"text": "done",
"variables": []
}
]
},
"created_at": {
"date": "2020-06-26 16:36:02.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"updated_at": {
"date": "2020-06-26 16:36:11.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"is_global": false,
"slots": []
}
],
"variables": [
{
"id": "a0a11bd0-b7ca-11ea-a1cf-4155954aa7c8",
"name": "name",
"default_value": "",
"type": "text",
"entity": "",
"created_at": {
"date": "2020-06-26 16:32:29.000000",
"timezone_type": 3,
"timezone": "UTC"
},
"updated_at": {
"date": "2020-06-26 16:32:29.000000",
"timezone_type": 3,
"timezone": "UTC"
}
}
],
"entities": []
}
getProject
Returns a Promise
which resolves an object containing high-level project data.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const project = await client.getProject("YOUR_TEAM_ID", "YOUR_PROJECT_ID");
// `project` contains the project data specified by the team id and project id passed to the method.
})();
getProjects
Returns a Promise
which resolves an array of objects containing high-level project data.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const projects = await client.getProjects([{ teamId: "YOUR_TEAM_ID", projectId: "YOUR_PROJECT_ID" }]);
// `projects` contains the project data specified by the series of team ids and project ids passed to the method.
})();
getTeam
Returns a Promise
which resolves an object containing high-level team data.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const team = await client.getTeam("YOUR_TEAM_ID");
// `team` contains the team data specified by the team id passed to the method.
})();
getTeams
Returns a Promise
which resolves an array of objects containing high-level team data.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const teams = await client.getTeams(["YOUR_TEAM_ID"]);
// `teams` contains the team data specified by the team ids passed to the method.
})();
getBoard
Returns a Promise
which resolves an object describing the specified board.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const board = await client.getBoard("YOUR_TEAM_ID", "YOUR_PROJECT_ID", "YOUR_BOARD_ID");
// `board` contains the board data specified by the ids passed to the method.
})();
getBoards
Returns a Promise
which resolves an array of objects describing the specified boards.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const boards = await client.getBoards("YOUR_TEAM_ID", "YOUR_PROJECT_ID", ["YOUR_BOARD_ID_1"]);
// `boards` contains the board data specified by the ids passed to the method.
})();
getIntents
Returns a Promise
which resolves an array of objects containing intent data for the specified project.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const intents = await client.getIntents("YOUR_TEAM_ID", "YOUR_PROJECT_ID");
// `intents` contains the intent data specified by the team id and project id passed to the method.
})();
getVariables
Returns a Promise
which resolves an array of objects containing variables data for the specified project.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const variables = await client.getVariables("YOUR_TEAM_ID", "YOUR_PROJECT_ID");
// `variables` contains the variables data specified by the team id and project id passed to the method.
})();
getEntities
Returns a Promise
which resolves an array of objects containing entities data for the specified project.
import { Client } from "@botmock/client";
const client = new Client({ token: "YOUR_TOKEN" });
(async () => {
const entities = await client.getVariables("YOUR_TEAM_ID", "YOUR_PROJECT_ID");
// `entities` contains the entities data specified by the team id and project id passed to the method.
})();
FAQs
## Overview
The npm package @botmock/client receives a total of 23 weekly downloads. As such, @botmock/client popularity was classified as not popular.
We found that @botmock/client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.