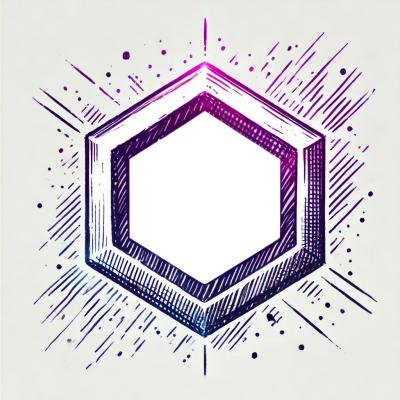
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
@bowtie/api
Advanced tools
JavaScript utilities and helpers
npm install --save @bowtie/api
// Require @bowtie/api class definition
const Api = require('@bowtie/api')
// Create api instance
const api = new Api({
root: 'api.example.com' // Will default to https:// if no protocol is provided here
})
// REST GET /todos - Get all todos
api.get('todos')
.then(resp => {
// resp = response from GET https://api.example.com/todos
})
.catch(err => {
// Something went wrong
})
// REST POST /todos - Create new todo
api.post('todos', { name: 'new todo' })
.then(resp => {
// resp = response from POST https://api.example.com/todos
})
.catch(err => {
// Something went wrong
})
// REST PUT /todos/1 - Update todo with id=1
api.put('todos/1', { name: 'changed name' })
.then(resp => {
// resp = response from PUT https://api.example.com/todos/1
})
.catch(err => {
// Something went wrong
})
// REST DELETE /todos/1 - Delete todo with id=1
api.delete('todos/1')
.then(resp => {
// resp = response from DELETE https://api.example.com/todos/1
})
.catch(err => {
// Something went wrong
})
// Create api instance
const api = new Api({
root: 'api.example.com', // Set beginning of the baseUrl to https://api.example.com
stage: 'test', // Append "/test" to baseUrl (i.e. https://api.example.com/test)
prefix: 'api', // Append "/api" to baseUrl (i.e. https://api.example.com/test/api)
version: 'v1' // Append "/v1" to baseUrl (i.e. https://api.example.com/test/api/v1)
})
// baseUrl is constructed as follows: (only api.root is required, any combination of stage/prefix/version is allowed)
baseUrl = `${api.root}/${api.stage}/${api.prefix}/${api.version}`;
// Create api instance
const api = new Api({
root: 'api.example.com', // Will default to https:// if no protocol is provided here
authorization: 'Basic' // Specify Basic authorization type for this api
})
// Authorize api for basic auth with username & password
api.authorize({
username: 'user',
password: 'pass'
})
// Create api instance
const api = new Api({
root: 'api.example.com', // Will default to https:// if no protocol is provided here
authorization: 'Bearer' // Specify Bearer authorization type for this api
})
// Authorize with static token value
api.authorize({
token: 'abc123'
})
// Authorize with dynamic token function
api.authorize({
token: () => localStorage.getItem('access_token')
})
// Create api instance
const api = new Api({
root: 'api.example.com', // Will default to https:// if no protocol is provided here
authorization: 'Custom' // Specify Custom authorization type for this api
})
// Authorize with static headers
api.authorize({
headers: {
'token': 'abc123'
},
validate: () => {
// Function to validate the custom auth, returns true/false
return true
}
})
// Authorize with dynamic headers
api.authorize({
token: () => ({
'token': localStorage.getItem('access_token'),
'uid': localStorage.getItem('uid')
}),
validate: () => {
// Function to validate the custom auth, returns true/false
return true
}
})
Example using the j-toker package with headers from devise_token_auth
const Auth = require('j-toker')
// Configure j-toker apiUrl to match api.baseUrl()
Auth.configure({
apiUrl: api.baseUrl()
})
// Authorize with custom headers that retrieve authHeaders from j-toker "Auth" instance
api.authorize({
headers: () => Auth.retrieveData('authHeaders'),
validate: () => Auth.user.signedIn
})
// Create api instance
const api = new Api({
root: 'http://localhost:3000', // Use a local development API over HTTP (not secure HTTPS)
secureOnly: false // Disable secure HTTPS requirement
})
// Create api instance
const api = new Api({
root: 'api.example.com', // Will default to https:// if no protocol is provided here
verbose: true // Set verbose=true to enable debug log output
})
FAQs
A simple class to standardize API interactions
We found that @bowtie/api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.