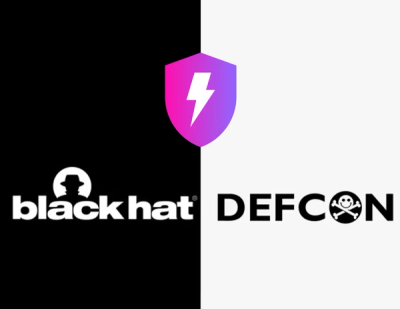
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
@catte_/pastebin.js
Advanced tools
An object-oriented JavaScript wrapper for the Pastebin API.
An object-oriented JavaScript wrapper for the Pastebin API.
$ npm install @catte_/pastebin.js
const { PastebinClient } = require("@catte_/pastebin.js")
const pastebin = new PastebinClient(process.env.API_KEY)
const paste = await pastebin.pastes.create("console.log('hello, world')", {
title: "pastebin.js test",
format: "javascript",
privacy: "unlisted"
})
console.log(paste.url)
The module exports the following classes, you can import them like so:
const {
PastebinClient,
PastebinError,
Paste,
User,
ClientUser,
PasteStore,
UserStore,
UserPasteStore
} = require("@catte_/pastebin.js")
The client used to interact with the Pastebin API.
new PastebinClient(apiKey, username, password)
name | description | type | default |
---|---|---|---|
apiKey | Your Pastebin API key | string | null |
username | Your Pastebin username | string | null |
password | Your Pastebin password | string | null |
Your Pastebin credentials.
Type: PastebinCredentials
The user the client logged in with, if it has.
Type: ?ClientUser
All of the cached users.
Type: ?UserStore
All of the cached pastes.
Type: ?PasteStore
Login with the stored username and password and store the user key.
Thrown when there's an error related to the Pastebin API or pastebin.js.
new PastebinError(message)
name | description | type | default |
---|---|---|---|
message | The error message | string |
The error message.
A Pastebin paste.
new Paste(client, data)
name | description | type | default |
---|---|---|---|
client | The client used to get this paste | PastebinClient | |
data | The data obtained from the API | Object |
The client used to get this paste.
Type: PastebinClient
The key of this paste.
Type: string
The URL of this paste.
Type: string
The title of this paste.
Type: ?string
The author of this paste.
Type: ?User
The content of this paste.
Type: ?string
The length of the content of this paste.
Type: ?number
The date this paste was posted.
Type: ?Date
The format of this paste.
Type: ?string
The privacy setting of this paste.
Type: ?number
The expiry time of this paste.
Type: ?string
The number of times anyone saw this paste.
Type: ?number
Fetch the content of this paste, and store it in the cache.
Delete this paste.
A Pastebin user.
new User(client, username)
name | description | type | default |
---|---|---|---|
client | The client used to get this paste | PastebinClient | |
username | The user's username | string |
The client used to get this user.
Type: PastebinClient
This user's username.
Type: string
Whether this user is the same as the client's user.
Type: boolean
The Pastebin user of the logged in client.
new ClientUser(client, data)
name | description | type | default |
---|---|---|---|
client | The client used to get this paste | PastebinClient | |
data | The data obtained from the API | Object |
This user's username.
Type: string
This user's format setting.
Type: ?string
This user's expiry setting.
Type: ?string
This user's avatar URL.
Type: ?string
This user's privacy setting.
Type: ?number
This user's website.
Type: ?string
This user's e-mail.
Type: ?string
This user's location.
Type: ?string
Whether this user is a PRO account.
Type: ?boolean
All of this user's cached pastes.
Type: ?UserPasteStore
A structure that holds all of the cached pastes.
new PasteStore(client, entries)
name | description | type | default |
---|---|---|---|
client | The client the store belongs to | PastebinClient | |
entries | Array<Array<string, Paste>> | null |
The client this store belongs to.
Type: PastebinClient
Fetch a paste by its key, and store it in the cache.
name | description | type | default |
---|---|---|---|
key | The paste's key | string |
Create a paste, and store it in the cache.
name | description | type | default |
---|---|---|---|
content | The paste's content | any | |
options | Array<Array<string, Paste>> | null | |
options.title | The paste's title | string | {} |
options.format | The paste's format | Format | null |
options.privacy | The paste's privacy setting | Privacy | null |
options.expiry | The paste's expiry time | Expiry | null |
A structure that holds all of the cached users.
new UserStore(client, entries)
name | description | type | default |
---|---|---|---|
client | The client the store belongs to | PastebinClient | |
entries | Array<Array<string, Paste>> | null |
The client this store belongs to.
Type: PastebinClient
Fetch a user by their username, and store them in the cache.
name | description | type | default |
---|---|---|---|
username | The user's username | string |
A structure that holds all of a user's cached pastes.
new UserPasteStore()
name | description | type | default |
---|---|---|---|
client | The client the store belongs to | PastebinClient | |
user | The user the store belongs to | User | |
entries | Array<Array<string, Paste>> | null |
The client this store belongs to.
Type: PastebinClient
The user this store belongs to.
Type: User
Fetch this user's pastes, and store them in the cache.
name | description | type | default |
---|---|---|---|
max | The maximum number of pastes to fetch | number | 50 |
The credentials provided to and stored in the PastebinClient.
Type: { apiKey?, username?, password?, userKey? }
name | description | type | default |
---|---|---|---|
apiKey | Your Pastebin API key | string | null |
username | Your Pastebin username | string | null |
password | Your Pastebin password | string | null |
userKey | Your Pastebin user key, obtained when logging in | string | null |
Options to create a paste.
Type: { title?, format?, privacy?, expiry? }
name | description | type | default |
---|---|---|---|
title | Your Pastebin API key | string | null |
format | Your Pastebin username | Format | null |
privacy | Your Pastebin password | Privacy | null |
expiry | Your Pastebin user key, obtained when logging in | Expiry | null |
A "format," which will be used for syntax highlighting. You can see the full list of formats here.
Type: string
A privacy setting. Can be one of the following:
0
(or "public"
)1
(or "unlisted"
)2
(or "private"
)Type: string
or number
An expiry setting. Can be one of the following:
"NEVER"
(or "N"
)"10 MINUTES"
(or "10M"
)"1 HOUR"
(or "1H"
)"1 DAY"
(or "1D"
)"1 WEEK"
(or "1W"
)"2 WEEKS"
(or "2W"
)"1 MONTH"
(or "1M"
)"6 MONTHS"
(or "6M"
)"1 YEAR"
(or "1Y"
)FAQs
An object-oriented JavaScript wrapper for the Pastebin API.
We found that @catte_/pastebin.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.